libuav original
Dependents: UAVCAN UAVCAN_Subscriber
ccand_11xx.h
00001 /* 00002 * @brief LPC11xx CCAN ROM API declarations and functions 00003 * 00004 * @note 00005 * Copyright(C) NXP Semiconductors, 2012 00006 * All rights reserved. 00007 * 00008 * @par 00009 * Software that is described herein is for illustrative purposes only 00010 * which provides customers with programming information regarding the 00011 * LPC products. This software is supplied "AS IS" without any warranties of 00012 * any kind, and NXP Semiconductors and its licensor disclaim any and 00013 * all warranties, express or implied, including all implied warranties of 00014 * merchantability, fitness for a particular purpose and non-infringement of 00015 * intellectual property rights. NXP Semiconductors assumes no responsibility 00016 * or liability for the use of the software, conveys no license or rights under any 00017 * patent, copyright, mask work right, or any other intellectual property rights in 00018 * or to any products. NXP Semiconductors reserves the right to make changes 00019 * in the software without notification. NXP Semiconductors also makes no 00020 * representation or warranty that such application will be suitable for the 00021 * specified use without further testing or modification. 00022 * 00023 * @par 00024 * Permission to use, copy, modify, and distribute this software and its 00025 * documentation is hereby granted, under NXP Semiconductors' and its 00026 * licensor's relevant copyrights in the software, without fee, provided that it 00027 * is used in conjunction with NXP Semiconductors microcontrollers. This 00028 * copyright, permission, and disclaimer notice must appear in all copies of 00029 * this code. 00030 */ 00031 00032 #ifndef __CCAND_11XX_H_ 00033 #define __CCAND_11XX_H_ 00034 00035 #ifdef __cplusplus 00036 extern "C" { 00037 #endif 00038 00039 /** @defgroup CCANROM_11XX CHIP: LPC11xx CCAN ROM Driver 00040 * @ingroup CHIP_11XX_Drivers 00041 * @{ 00042 */ 00043 00044 /** 00045 * CCAN ROM error status bits 00046 */ 00047 #define CAN_ERROR_NONE 0x00000000UL 00048 #define CAN_ERROR_PASS 0x00000001UL 00049 #define CAN_ERROR_WARN 0x00000002UL 00050 #define CAN_ERROR_BOFF 0x00000004UL 00051 #define CAN_ERROR_STUF 0x00000008UL 00052 #define CAN_ERROR_FORM 0x00000010UL 00053 #define CAN_ERROR_ACK 0x00000020UL 00054 #define CAN_ERROR_BIT1 0x00000040UL 00055 #define CAN_ERROR_BIT0 0x00000080UL 00056 #define CAN_ERROR_CRC 0x00000100UL 00057 00058 /** 00059 * CCAN ROM control bits for CAN_MSG_OBJ.mode_id 00060 */ 00061 #define CAN_MSGOBJ_STD 0x00000000UL /* CAN 2.0a 11-bit ID */ 00062 #define CAN_MSGOBJ_EXT 0x20000000UL /* CAN 2.0b 29-bit ID */ 00063 #define CAN_MSGOBJ_DAT 0x00000000UL /* data frame */ 00064 #define CAN_MSGOBJ_RTR 0x40000000UL /* rtr frame */ 00065 00066 typedef struct CCAN_MSG_OBJ { 00067 uint32_t mode_id; 00068 uint32_t mask; 00069 uint8_t data[8]; 00070 uint8_t dlc; 00071 uint8_t msgobj; 00072 } CCAN_MSG_OBJ_T; 00073 00074 /************************************************************************** 00075 SDO Abort Codes 00076 **************************************************************************/ 00077 #define SDO_ABORT_TOGGLE 0x05030000UL // Toggle bit not alternated 00078 #define SDO_ABORT_SDOTIMEOUT 0x05040000UL // SDO protocol timed out 00079 #define SDO_ABORT_UNKNOWN_COMMAND 0x05040001UL // Client/server command specifier not valid or unknown 00080 #define SDO_ABORT_UNSUPPORTED 0x06010000UL // Unsupported access to an object 00081 #define SDO_ABORT_WRITEONLY 0x06010001UL // Attempt to read a write only object 00082 #define SDO_ABORT_READONLY 0x06010002UL // Attempt to write a read only object 00083 #define SDO_ABORT_NOT_EXISTS 0x06020000UL // Object does not exist in the object dictionary 00084 #define SDO_ABORT_PARAINCOMP 0x06040043UL // General parameter incompatibility reason 00085 #define SDO_ABORT_ACCINCOMP 0x06040047UL // General internal incompatibility in the device 00086 #define SDO_ABORT_TYPEMISMATCH 0x06070010UL // Data type does not match, length of service parameter does not match 00087 #define SDO_ABORT_UNKNOWNSUB 0x06090011UL // Sub-index does not exist 00088 #define SDO_ABORT_VALUE_RANGE 0x06090030UL // Value range of parameter exceeded (only for write access) 00089 #define SDO_ABORT_TRANSFER 0x08000020UL // Data cannot be transferred or stored to the application 00090 #define SDO_ABORT_LOCAL 0x08000021UL // Data cannot be transferred or stored to the application because of local control 00091 #define SDO_ABORT_DEVSTAT 0x08000022UL // Data cannot be transferred or stored to the application because of the present device state 00092 00093 typedef struct CCAN_ODCONSTENTRY { 00094 uint16_t index; 00095 uint8_t subindex; 00096 uint8_t len; 00097 uint32_t val; 00098 } CCAN_ODCONSTENTRY_T; 00099 00100 // upper-nibble values for CAN_ODENTRY.entrytype_len 00101 #define OD_NONE 0x00 // Object Dictionary entry doesn't exist 00102 #define OD_EXP_RO 0x10 // Object Dictionary entry expedited, read-only 00103 #define OD_EXP_WO 0x20 // Object Dictionary entry expedited, write-only 00104 #define OD_EXP_RW 0x30 // Object Dictionary entry expedited, read-write 00105 #define OD_SEG_RO 0x40 // Object Dictionary entry segmented, read-only 00106 #define OD_SEG_WO 0x50 // Object Dictionary entry segmented, write-only 00107 #define OD_SEG_RW 0x60 // Object Dictionary entry segmented, read-write 00108 00109 typedef struct CCAN_ODENTRY { 00110 uint16_t index; 00111 uint8_t subindex; 00112 uint8_t entrytype_len; 00113 uint8_t *val; 00114 } CCAN_ODENTRY_T; 00115 00116 typedef struct CCAN_CANOPENCFG { 00117 uint8_t node_id; 00118 uint8_t msgobj_rx; 00119 uint8_t msgobj_tx; 00120 uint8_t isr_handled; 00121 uint32_t od_const_num; 00122 CCAN_ODCONSTENTRY_T *od_const_table; 00123 uint32_t od_num; 00124 CCAN_ODENTRY_T *od_table; 00125 } CCAN_CANOPENCFG_T; 00126 00127 // Return values for CANOPEN_sdo_req() callback 00128 #define CAN_SDOREQ_NOTHANDLED 0 // process regularly, no impact 00129 #define CAN_SDOREQ_HANDLED_SEND 1 // processed in callback, auto-send returned msg 00130 #define CAN_SDOREQ_HANDLED_NOSEND 2 // processed in callback, don't send response 00131 00132 // Values for CANOPEN_sdo_seg_read/write() callback 'openclose' parameter 00133 #define CAN_SDOSEG_SEGMENT 0 // segment read/write 00134 #define CAN_SDOSEG_OPEN 1 // channel is opened 00135 #define CAN_SDOSEG_CLOSE 2 // channel is closed 00136 00137 typedef struct CCAN_CALLBACKS { 00138 void (*CAN_rx)(uint8_t msg_obj_num); 00139 void (*CAN_tx)(uint8_t msg_obj_num); 00140 void (*CAN_error)(uint32_t error_info); 00141 uint32_t (*CANOPEN_sdo_read)(uint16_t index, uint8_t subindex); 00142 uint32_t (*CANOPEN_sdo_write)(uint16_t index, uint8_t subindex, uint8_t *dat_ptr); 00143 uint32_t (*CANOPEN_sdo_seg_read)(uint16_t index, uint8_t subindex, uint8_t openclose, uint8_t *length, 00144 uint8_t *data, uint8_t *last); 00145 uint32_t (*CANOPEN_sdo_seg_write)(uint16_t index, uint8_t subindex, uint8_t openclose, uint8_t length, 00146 uint8_t *data, uint8_t *fast_resp); 00147 uint8_t (*CANOPEN_sdo_req)(uint8_t length_req, uint8_t *req_ptr, uint8_t *length_resp, uint8_t *resp_ptr); 00148 } CCAN_CALLBACKS_T; 00149 00150 typedef struct CCAN_API { 00151 void (*init_can)(uint32_t *can_cfg, uint8_t isr_ena); 00152 void (*isr)(void); 00153 void (*config_rxmsgobj)(CCAN_MSG_OBJ_T *msg_obj); 00154 uint8_t (*can_receive)(CCAN_MSG_OBJ_T *msg_obj); 00155 void (*can_transmit)(CCAN_MSG_OBJ_T *msg_obj); 00156 void (*config_canopen)(CCAN_CANOPENCFG_T *canopen_cfg); 00157 void (*canopen_handler)(void); 00158 void (*config_calb)(CCAN_CALLBACKS_T *callback_cfg); 00159 } CCAN_API_T; 00160 00161 #define LPC_CCAN_API ((CCAN_API_T *) (LPC_ROM_API->candApiBase)) 00162 /** 00163 * @} 00164 */ 00165 00166 #ifdef __cplusplus 00167 } 00168 #endif 00169 00170 #endif /* __CCAND_11XX_H_ */
Generated on Tue Jul 12 2022 17:17:30 by
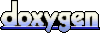