libuav original
Dependents: UAVCAN UAVCAN_Subscriber
c_can.hpp
00001 /* 00002 * Bosch C_CAN controller API. 00003 * 00004 * Copyright (C) 2015 Pavel Kirienko <pavel.kirienko@gmail.com> 00005 */ 00006 00007 #pragma once 00008 00009 #include <cstdint> 00010 #include <cstddef> 00011 00012 namespace uavcan_lpc11c24 00013 { 00014 namespace c_can 00015 { 00016 00017 struct MsgIfaceType 00018 { 00019 std::uint32_t CMDREQ; 00020 00021 union 00022 { 00023 std::uint32_t W; 00024 std::uint32_t R; 00025 } CMDMSK; 00026 00027 std::uint32_t MSK1; 00028 std::uint32_t MSK2; 00029 00030 std::uint32_t ARB1; 00031 std::uint32_t ARB2; 00032 00033 std::uint32_t MCTRL; 00034 00035 std::uint32_t DA1; 00036 std::uint32_t DA2; 00037 std::uint32_t DB1; 00038 std::uint32_t DB2; 00039 00040 const std::uint32_t _skip[13]; 00041 }; 00042 00043 static_assert(offsetof(MsgIfaceType, CMDMSK) == 0x04, "C_CAN offset"); 00044 static_assert(offsetof(MsgIfaceType, MSK1) == 0x08, "C_CAN offset"); 00045 static_assert(offsetof(MsgIfaceType, ARB1) == 0x10, "C_CAN offset"); 00046 static_assert(offsetof(MsgIfaceType, MCTRL) == 0x18, "C_CAN offset"); 00047 static_assert(offsetof(MsgIfaceType, DA1) == 0x1c, "C_CAN offset"); 00048 static_assert(offsetof(MsgIfaceType, DB2) == 0x28, "C_CAN offset"); 00049 00050 static_assert(sizeof(MsgIfaceType) == 96, "C_CAN size"); 00051 00052 00053 struct Type 00054 { 00055 std::uint32_t CNTL; 00056 std::uint32_t STAT; 00057 const std::uint32_t EC; 00058 std::uint32_t BT; 00059 const std::uint32_t INT; 00060 std::uint32_t TEST; 00061 std::uint32_t BRPE; 00062 00063 const std::uint32_t _skip_a[1]; 00064 00065 MsgIfaceType IF[2]; // [0] @ 0x020, [1] @ 0x080 00066 00067 const std::uint32_t _skip_b[8]; 00068 00069 const std::uint32_t TXREQ[2]; // 0x100 00070 00071 const std::uint32_t _skip_c[6]; 00072 00073 const std::uint32_t ND[2]; // 0x120 00074 00075 const std::uint32_t _skip_d[6]; 00076 00077 const std::uint32_t IR[2]; // 0x140 00078 00079 const std::uint32_t _skip_e[6]; 00080 00081 const std::uint32_t MSGV[2]; // 0x160 00082 00083 const std::uint32_t _skip_f[6]; 00084 00085 std::uint32_t CLKDIV; // 0x180 00086 }; 00087 00088 static_assert(offsetof(Type, CNTL) == 0x000, "C_CAN offset"); 00089 static_assert(offsetof(Type, STAT) == 0x004, "C_CAN offset"); 00090 static_assert(offsetof(Type, TEST) == 0x014, "C_CAN offset"); 00091 static_assert(offsetof(Type, BRPE) == 0x018, "C_CAN offset"); 00092 static_assert(offsetof(Type, IF[0]) == 0x020, "C_CAN offset"); 00093 static_assert(offsetof(Type, IF[1]) == 0x080, "C_CAN offset"); 00094 static_assert(offsetof(Type, TXREQ) == 0x100, "C_CAN offset"); 00095 static_assert(offsetof(Type, ND) == 0x120, "C_CAN offset"); 00096 static_assert(offsetof(Type, IR) == 0x140, "C_CAN offset"); 00097 static_assert(offsetof(Type, MSGV) == 0x160, "C_CAN offset"); 00098 static_assert(offsetof(Type, CLKDIV) == 0x180, "C_CAN offset"); 00099 00100 static_assert(offsetof(Type, IF[0].DB2) == 0x048, "C_CAN offset"); 00101 static_assert(offsetof(Type, IF[1].DB2) == 0x0A8, "C_CAN offset"); 00102 00103 00104 volatile Type& CAN = *reinterpret_cast<volatile Type*>(0x40050000); 00105 00106 00107 /* 00108 * CNTL 00109 */ 00110 static constexpr std::uint32_t CNTL_TEST = 1 << 7; 00111 static constexpr std::uint32_t CNTL_CCE = 1 << 6; 00112 static constexpr std::uint32_t CNTL_DAR = 1 << 5; 00113 static constexpr std::uint32_t CNTL_EIE = 1 << 3; 00114 static constexpr std::uint32_t CNTL_SIE = 1 << 2; 00115 static constexpr std::uint32_t CNTL_IE = 1 << 1; 00116 static constexpr std::uint32_t CNTL_INIT = 1 << 0; 00117 00118 static constexpr std::uint32_t CNTL_IRQ_MASK = CNTL_EIE | CNTL_IE | CNTL_SIE; 00119 00120 /* 00121 * TEST 00122 */ 00123 static constexpr std::uint32_t TEST_RX = 1 << 7; 00124 static constexpr std::uint32_t TEST_LBACK = 1 << 4; 00125 static constexpr std::uint32_t TEST_SILENT = 1 << 3; 00126 static constexpr std::uint32_t TEST_BASIC = 1 << 2; 00127 static constexpr std::uint32_t TEST_TX_SHIFT = 5; 00128 00129 enum class TestTx : std::uint32_t 00130 { 00131 Controller = 0, 00132 SamplePoint = 1, 00133 LowDominant = 2, 00134 HighRecessive = 3 00135 }; 00136 00137 /* 00138 * STAT 00139 */ 00140 static constexpr std::uint32_t STAT_BOFF = 1 << 7; 00141 static constexpr std::uint32_t STAT_EWARN = 1 << 6; 00142 static constexpr std::uint32_t STAT_EPASS = 1 << 5; 00143 static constexpr std::uint32_t STAT_RXOK = 1 << 4; 00144 static constexpr std::uint32_t STAT_TXOK = 1 << 3; 00145 static constexpr std::uint32_t STAT_LEC_MASK = 7; 00146 static constexpr std::uint32_t STAT_LEC_SHIFT = 0; 00147 00148 enum class StatLec : std::uint32_t 00149 { 00150 NoError = 0, 00151 StuffError = 1, 00152 FormError = 2, 00153 AckError = 3, 00154 Bit1Error = 4, 00155 Bit0Error = 5, 00156 CRCError = 6, 00157 Unused = 7 00158 }; 00159 00160 /* 00161 * IF.CMDREQ 00162 */ 00163 static constexpr std::uint32_t IF_CMDREQ_BUSY = 1 << 15; 00164 00165 /* 00166 * IF.CMDMSK 00167 */ 00168 static constexpr std::uint32_t IF_CMDMSK_W_DATA_A = 1 << 0; 00169 static constexpr std::uint32_t IF_CMDMSK_W_DATA_B = 1 << 1; 00170 static constexpr std::uint32_t IF_CMDMSK_W_TXRQST = 1 << 2; 00171 static constexpr std::uint32_t IF_CMDMSK_W_CTRL = 1 << 4; 00172 static constexpr std::uint32_t IF_CMDMSK_W_ARB = 1 << 5; 00173 static constexpr std::uint32_t IF_CMDMSK_W_MASK = 1 << 6; 00174 static constexpr std::uint32_t IF_CMDMSK_W_WR_RD = 1 << 7; 00175 00176 /* 00177 * IF.MCTRL 00178 */ 00179 static constexpr std::uint32_t IF_MCTRL_NEWDAT = 1 << 15; 00180 static constexpr std::uint32_t IF_MCTRL_MSGLST = 1 << 14; 00181 static constexpr std::uint32_t IF_MCTRL_INTPND = 1 << 13; 00182 static constexpr std::uint32_t IF_MCTRL_UMASK = 1 << 12; 00183 static constexpr std::uint32_t IF_MCTRL_TXIE = 1 << 11; 00184 static constexpr std::uint32_t IF_MCTRL_RXIE = 1 << 10; 00185 static constexpr std::uint32_t IF_MCTRL_RMTEN = 1 << 9; 00186 static constexpr std::uint32_t IF_MCTRL_TXRQST = 1 << 8; 00187 static constexpr std::uint32_t IF_MCTRL_EOB = 1 << 7; 00188 static constexpr std::uint32_t IF_MCTRL_DLC_MASK = 15; 00189 00190 } 00191 }
Generated on Tue Jul 12 2022 17:17:30 by
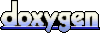