libuav original
Dependents: UAVCAN UAVCAN_Subscriber
board.h
00001 /* 00002 * Copyright (C) 2014 Pavel Kirienko <pavel.kirienko@gmail.com> 00003 */ 00004 00005 #pragma once 00006 00007 /// Assert is needed for STM32 SPL (if it is being used, that is) 00008 #include <assert.h> 00009 #define assert_param(x) assert(x) 00010 00011 #define STM32_LSECLK 32768 00012 #define STM32_HSECLK 25000000 00013 00014 #define STM32F10X_CL 00015 00016 /* 00017 * GPIO 00018 */ 00019 // LED 00020 #define GPIO_PORT_LED GPIOB 00021 #define GPIO_PIN_LED 9 00022 00023 // GPIOD 10 is configured as OUTPUT, it is used as board reboot monitor. 00024 00025 /* 00026 * I/O ports initial setup, this configuration is established soon after reset 00027 * in the initialization code. 00028 * 00029 * The digits have the following meaning: 00030 * 0 - Analog input. 00031 * 1 - Push Pull output 10MHz. 00032 * 2 - Push Pull output 2MHz. 00033 * 3 - Push Pull output 50MHz. 00034 * 4 - Digital input. 00035 * 5 - Open Drain output 10MHz. 00036 * 6 - Open Drain output 2MHz. 00037 * 7 - Open Drain output 50MHz. 00038 * 8 - Digital input with PullUp or PullDown resistor depending on ODR. 00039 * 9 - Alternate Push Pull output 10MHz. 00040 * A - Alternate Push Pull output 2MHz. 00041 * B - Alternate Push Pull output 50MHz. 00042 * C - Reserved. 00043 * D - Alternate Open Drain output 10MHz. 00044 * E - Alternate Open Drain output 2MHz. 00045 * F - Alternate Open Drain output 50MHz. 00046 * Please refer to the STM32 Reference Manual for details. 00047 */ 00048 00049 #define VAL_GPIOACRL 0x88888888 // 7..0 00050 #define VAL_GPIOACRH 0x88888888 // 15..8 00051 #define VAL_GPIOAODR 0x00000000 00052 00053 #define VAL_GPIOBCRL 0x84488888 // CAN2 TX initialized as INPUT, it must be configured later! 00054 #define VAL_GPIOBCRH 0x88888828 00055 #define VAL_GPIOBODR 0x00000000 00056 00057 #define VAL_GPIOCCRL 0x88888888 00058 #define VAL_GPIOCCRH 0x88888888 00059 #define VAL_GPIOCODR 0x00000000 00060 00061 #define VAL_GPIODCRL 0x88b88844 // CAN1 TX initialized as INPUT, it must be configured later! 00062 #define VAL_GPIODCRH 0x88888288 00063 #define VAL_GPIODODR ((1 << 10)) 00064 00065 #define VAL_GPIOECRL 0x88888888 00066 #define VAL_GPIOECRH 0x88888888 00067 #define VAL_GPIOEODR 0x00000000 00068 00069 #if !defined(_FROM_ASM_) 00070 #ifdef __cplusplus 00071 extern "C" { 00072 #endif 00073 void boardInit(void); 00074 #ifdef __cplusplus 00075 } 00076 #endif 00077 #endif /* _FROM_ASM_ */
Generated on Tue Jul 12 2022 17:17:30 by
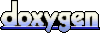