
emg dingetje met moving avarage
Dependencies: HIDScope biquadFilter circular_buffer mbed
Fork of EMG by
main.cpp
00001 #include "mbed.h" 00002 #include "HIDScope.h" 00003 #include "BiQuad.h" 00004 #include "math.h" 00005 00006 00007 //Define objects 00008 AnalogIn emg0( A0 ); 00009 AnalogIn emg1( A1 ); 00010 00011 Ticker sample_timer; 00012 HIDScope scope( 3 ); 00013 DigitalOut led(LED1); 00014 00015 /** Sample function 00016 * this function samples the emg and sends it to HIDScope 00017 **/ 00018 int P= 200; 00019 double A[200]; 00020 00021 00022 void sample() 00023 { 00024 /* Set the sampled emg values in channel 0 (the first channel) and 1 (the second channel) in the 'HIDScope' instance named 'scope' */ 00025 scope.set(0, emg0.read() ); 00026 scope.set(1, emg1.read() ); 00027 /* Repeat the step above if required for more channels of required (channel 0 up to 5 = 6 channels) 00028 * Ensure that enough channels are available (HIDScope scope( 2 )) 00029 * Finally, send all channels to the PC at once */ 00030 scope.send(); 00031 /* To indicate that the function is working, the LED is toggled */ 00032 led = !led; 00033 } 00034 00035 BiQuadChain bqc; 00036 BiQuad bq1( 0.6844323315947305,1.368864663189461, 0.6844323315947305,1.2243497755555954,0.5133795508233265); 00037 BiQuad bq2( 0.6844323315947306, -1.3688646631894612, 0.6844323315947306, -1.2243497755555959, 0.5133795508233266); 00038 BiQuad bq3( 0.7566897754116633, -1.2243497755555959, 0.7566897754116633, -1.2243497755555959, 0.5133795508233266); 00039 00040 Ticker emgSampleTicker; 00041 AnalogIn emg( A0 ); 00042 00043 void emgSample() { 00044 00045 double emgFiltered = bqc.step( emg.read() ); 00046 double emgabs = abs(emgFiltered); 00047 scope.set(0, emgFiltered ); 00048 scope.set(1, emgabs ); 00049 00050 for(int i = P-1; i >= 0; i--){ 00051 if (i == 0) { 00052 A[i] = emgabs; 00053 } 00054 else { 00055 A[i] = A[i-1]; 00056 } 00057 } 00058 double sum = 0; 00059 for (int n = 0; n < P-1; n++) { 00060 sum = sum + A[n]; 00061 } 00062 00063 double movmean = sum/P; 00064 00065 scope.set(2, movmean); 00066 scope.send(); 00067 } 00068 00069 00070 int main() 00071 { 00072 bqc.add( &bq1 ).add( &bq2 ).add( &bq3 ); 00073 emgSampleTicker.attach( &emgSample, 0.002 ); 00074 /**Attach the 'sample' function to the timer 'sample_timer'. 00075 * this ensures that 'sample' is executed every... 0.002 seconds = 500 Hz 00076 */ 00077 //sample_timer.attach(&sample, 0.01); 00078 00079 /*empty loop, sample() is executed periodically*/ 00080 while(1) {} 00081 }
Generated on Tue Aug 16 2022 01:08:28 by
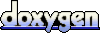