
2a
Dependencies: BSP_DISCO_F429ZI LCD_DISCO_F429ZI TS_DISCO_F429ZI mbed
main.cpp
00001 #include "mbed.h" 00002 #include "TS_DISCO_F429ZI.h" 00003 #include "LCD_DISCO_F429ZI.h" 00004 00005 LCD_DISCO_F429ZI lcd; 00006 TS_DISCO_F429ZI ts; 00007 bool touchInRect(uint16_t TouchXpos, uint16_t TouchYpos,uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00008 { 00009 if((TouchXpos>=Xpos)&&(TouchXpos<=Xpos+Width)&&(TouchYpos>=Ypos)&&(TouchYpos<=Ypos+Height))return (true); 00010 else return(false); 00011 } 00012 00013 void drawNumber() 00014 { 00015 uint8_t text[3]; 00016 lcd.SetTextColor(LCD_COLOR_WHITE); 00017 lcd.SetBackColor(LCD_COLOR_RED); 00018 for(uint8_t i=0; i<4; i++) { 00019 sprintf((char*)text, "%d", i); 00020 lcd.DisplayStringAt(0, 0+i*80, (uint8_t *)&text, LEFT_MODE); 00021 } 00022 lcd.SetBackColor(LCD_COLOR_BLACK); 00023 } 00024 int main() 00025 { 00026 TS_StateTypeDef TS_State; 00027 uint16_t x, y; 00028 uint8_t text[30]; 00029 uint8_t status; 00030 bool UpdateAfterTouch=true; 00031 00032 BSP_LCD_SetFont(&Font20); 00033 00034 lcd.DisplayStringAt(0, LINE(5), (uint8_t *)"TOUCHSCREEN", CENTER_MODE); 00035 lcd.DisplayStringAt(0, LINE(6), (uint8_t *)"DEMO", CENTER_MODE); 00036 wait_ms(500); 00037 00038 status = ts.Init(lcd.GetXSize(), lcd.GetYSize()); 00039 00040 if (status != TS_OK) { 00041 lcd.Clear(LCD_COLOR_RED); 00042 lcd.SetBackColor(LCD_COLOR_RED); 00043 lcd.SetTextColor(LCD_COLOR_WHITE); 00044 lcd.DisplayStringAt(0, LINE(5), (uint8_t *)"TOUCHSCREEN", CENTER_MODE); 00045 lcd.DisplayStringAt(0, LINE(6), (uint8_t *)"INIT FAIL", CENTER_MODE); 00046 } else { 00047 lcd.Clear(LCD_COLOR_GREEN); 00048 lcd.SetBackColor(LCD_COLOR_GREEN); 00049 lcd.SetTextColor(LCD_COLOR_WHITE); 00050 lcd.DisplayStringAt(0, LINE(5), (uint8_t *)"TOUCHSCREEN", CENTER_MODE); 00051 lcd.DisplayStringAt(0, LINE(6), (uint8_t *)"INIT OK", CENTER_MODE); 00052 } 00053 00054 wait_ms(500); 00055 00056 00057 lcd.Clear(LCD_COLOR_BLACK); 00058 lcd.SetBackColor(LCD_COLOR_BLACK); 00059 lcd.SetTextColor(LCD_COLOR_GREEN); 00060 00061 for(uint8_t i=0; i<4; i++) 00062 lcd.DrawRect(0,0+80*i,80,80); 00063 00064 lcd.SetFont(&Font24); 00065 00066 while(1) { 00067 wait_ms(100); 00068 00069 00070 ts.GetState(&TS_State); 00071 if (TS_State.TouchDetected) { 00072 UpdateAfterTouch=true; 00073 x = TS_State.X; 00074 y = TS_State.Y; 00075 lcd.SetTextColor(LCD_COLOR_GREEN); 00076 for(uint8_t i=0; i<4; i++) 00077 if(touchInRect(x,y,1,1+80*i,79,79)) 00078 lcd.FillRect(1,1+80*i,79,79); 00079 drawNumber(); 00080 } else if(UpdateAfterTouch == true) { 00081 UpdateAfterTouch=false; 00082 lcd.SetTextColor(LCD_COLOR_BLUE); 00083 for(uint8_t i=0; i<4; i++) 00084 lcd.FillRect(1,1+80*i,79,79); 00085 drawNumber(); 00086 } 00087 00088 00089 } 00090 }
Generated on Sat Aug 6 2022 09:42:19 by
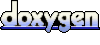