SocketGUS
Fork of Socket by
Embed:
(wiki syntax)
Show/hide line numbers
TCPSocketConnection.cpp
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 #include "TCPSocketConnection.h" 00019 #include <cstring> 00020 00021 using std::memset; 00022 using std::memcpy; 00023 00024 TCPSocketConnection::TCPSocketConnection() : 00025 _is_connected(false) 00026 { 00027 } 00028 00029 int TCPSocketConnection::connect(const char* host, const int port) 00030 { 00031 if (init_socket(SOCK_STREAM) < 0) 00032 return -1; 00033 //quitar else 00034 else if (set_address(host, port) != 0) 00035 return -1; 00036 //quitar else 00037 else if (lwip_connect(_sock_fd, (const struct sockaddr *) &_remoteHost, sizeof(_remoteHost)) < 0) { 00038 close(); 00039 return -1; 00040 } else {//quitar else 00041 _is_connected = true; 00042 return 0; 00043 }//quitar 00044 } 00045 00046 bool TCPSocketConnection::is_connected(void) 00047 { 00048 return _is_connected; 00049 } 00050 00051 int TCPSocketConnection::send(char* data, int length) 00052 { 00053 if ((_sock_fd < 0) || !_is_connected) 00054 return -1; 00055 00056 if (!_blocking) { 00057 TimeInterval timeout(_timeout); 00058 if (wait_writable(timeout) != 0) 00059 return -1; 00060 } 00061 00062 int n = lwip_send(_sock_fd, data, length, 0); 00063 _is_connected = (n != 0); 00064 00065 return n; 00066 } 00067 00068 // -1 if unsuccessful, else number of bytes written 00069 int TCPSocketConnection::send_all(char* data, int length) 00070 { 00071 if ((_sock_fd < 0) || !_is_connected) 00072 return -1; 00073 00074 int writtenLen = 0; 00075 TimeInterval timeout(_timeout); 00076 while (writtenLen < length) { 00077 if (!_blocking) { 00078 // Wait for socket to be writeable 00079 if (wait_writable(timeout) != 0) 00080 return writtenLen; 00081 } 00082 00083 int ret = lwip_send(_sock_fd, data + writtenLen, length - writtenLen, 0); 00084 if (ret > 0) { 00085 writtenLen += ret; 00086 continue; 00087 } else if (ret == 0) { 00088 _is_connected = false; 00089 return writtenLen; 00090 } else { 00091 return -1; //Connnection error 00092 } 00093 } 00094 return writtenLen; 00095 } 00096 00097 int TCPSocketConnection::receive(char* data, int length) 00098 { 00099 if ((_sock_fd < 0) || !_is_connected) 00100 return -1; 00101 00102 if (!_blocking) { 00103 TimeInterval timeout(_timeout); 00104 if (wait_readable(timeout) != 0) 00105 return -1; 00106 } 00107 00108 int n = lwip_recv(_sock_fd, data, length, 0); 00109 _is_connected = (n != 0); 00110 00111 return n; 00112 } 00113 00114 // -1 if unsuccessful, else number of bytes received 00115 int TCPSocketConnection::receive_all(char* data, int length) 00116 { 00117 if ((_sock_fd < 0) || !_is_connected) 00118 return -1; 00119 00120 int readLen = 0; 00121 TimeInterval timeout(_timeout); 00122 while (readLen < length) { 00123 if (!_blocking) { 00124 //Wait for socket to be readable 00125 if (wait_readable(timeout) != 0) 00126 return readLen; 00127 } 00128 00129 int ret = lwip_recv(_sock_fd, data + readLen, length - readLen, 0); 00130 if (ret > 0) { 00131 readLen += ret; 00132 } else if (ret == 0) { 00133 _is_connected = false; 00134 return readLen; 00135 } else { 00136 return -1; //Connnection error 00137 } 00138 } 00139 return readLen; 00140 }
Generated on Wed Jul 13 2022 04:30:44 by
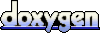