Freedman v2
Fork of WizFi250Interface by
Embed:
(wiki syntax)
Show/hide line numbers
TCPSocketConnection.cpp
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 /* Copyright (C) 2014 Wiznet, MIT License 00019 * port to the Wiznet Module WizFi250 00020 */ 00021 00022 00023 #include "TCPSocketConnection.h" 00024 #include <algorithm> 00025 00026 TCPSocketConnection::TCPSocketConnection() {} 00027 00028 int TCPSocketConnection::connect(const char* host, const int port) 00029 { 00030 if (set_address(host, port) != 0) return -1; 00031 00032 _server = false; 00033 _cid = _wizfi250->open(WizFi250::PROTO_TCP, get_address(), get_port()); 00034 if (_cid < 0 ) return -1; 00035 00036 return 0; 00037 } 00038 00039 bool TCPSocketConnection::is_connected(void) 00040 { 00041 bool _is_connected = _wizfi250->isConnected(_cid); 00042 if(!_is_connected) _cid = -1; 00043 00044 return _is_connected; 00045 } 00046 00047 int TCPSocketConnection::send(char *data, int length) 00048 { 00049 if (_cid < 0 || !is_connected()) return -1; 00050 00051 return _wizfi250->send(_cid, data, length); 00052 } 00053 00054 int TCPSocketConnection::send_all(char *data, int length) 00055 { 00056 Timer tmr; 00057 int idx = 0; 00058 00059 if(_cid <0 || !is_connected()) return -1; 00060 00061 tmr.start(); 00062 while((tmr.read_ms() < _timeout) || _blocking) 00063 { 00064 idx += _wizfi250->send(_cid, &data[idx], length - idx); 00065 if(idx < 0) return -1; 00066 00067 if(idx == length) 00068 return idx; 00069 } 00070 return (idx == 0) ? -1 : idx; 00071 } 00072 00073 int TCPSocketConnection::receive(char* data, int length) 00074 { 00075 Timer tmr; 00076 int time = -1; 00077 int len = 0; 00078 00079 if(_cid < 0 || !is_connected()) return -1; 00080 00081 if(_blocking) 00082 { 00083 tmr.start(); 00084 while(time < _timeout) 00085 { 00086 len = _wizfi250->readable(_cid); 00087 if(len == -1) 00088 return len; 00089 00090 if(len > 0) 00091 { 00092 WIZ_DBG("receive readable : %d\r\n",len); 00093 break; 00094 } 00095 time = tmr.read_ms(); 00096 } 00097 if(time >= _timeout) 00098 { 00099 //WIZ_DBG("receive timeout"); 00100 return 0; 00101 } 00102 } 00103 00104 return _wizfi250->recv(_cid, data, length); 00105 } 00106 00107 int TCPSocketConnection::receive_all(char* data, int length) 00108 { 00109 Timer tmr; 00110 int idx = 0; 00111 int time = -1; 00112 00113 if(_cid < 0 || !is_connected()) return -1; 00114 00115 tmr.start(); 00116 00117 while(time < _timeout || _blocking) 00118 { 00119 idx += _wizfi250->recv(_cid, &data[idx], length - idx); 00120 if (idx < 0) return -1; 00121 00122 if(idx == length) 00123 break; 00124 00125 time = tmr.read_ms(); 00126 } 00127 00128 return (idx == 0) ? -1 : idx; 00129 } 00130 00131 void TCPSocketConnection::acceptCID (int cid) 00132 { 00133 char *ip; 00134 int port; 00135 _server = true; 00136 _cid = cid; 00137 00138 00139 if( _wizfi250->cmdSMGMT(cid) ) return; 00140 if( !_wizfi250->getRemote(_cid, &ip, &port)) 00141 { 00142 set_address(ip, port); 00143 } 00144 }
Generated on Sat Jul 16 2022 22:06:24 by
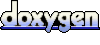