
FTPClient
Dependencies: FTPClient SDFileSystem WIZnetInterface_Ricky mbed
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetInterface.h" 00003 #include "FTPClient.h" 00004 00005 uint8_t mac_addr[6] = {0x00, 0x08, 0xdc, 0x12, 0x34, 0x45}; 00006 const char ip_addr[] = "192.168.0.123"; 00007 const char mask_addr[] = "255.255.255.0"; 00008 const char gateway_addr[] = "192.168.0.1"; 00009 00010 FTPClient ftp(PB_3, PB_2, PB_1, PB_0, "ftp"); 00011 00012 int main (void) 00013 { 00014 int n; 00015 EthernetInterface eth; 00016 eth.init(mac_addr, ip_addr, mask_addr, gateway_addr); //Use Static 00017 eth.connect(); 00018 00019 n = ftp.open("192.168.0.2", 21, "abc", "123"); 00020 if(n>1){ 00021 printf("Connect Success to FTPServer\r\n"); 00022 } 00023 printf("FTPServer dir...\r\n"); 00024 ftp.dir(); 00025 printf("FTPServer ls...\r\n"); 00026 ftp.ls(); 00027 00028 ftp.getfile("/ftp/1.jpg", "1.jpg"); 00029 ftp.getfile("/ftp/2.jpg", "2.jpg"); 00030 ftp.getfile("/ftp/3.jpg", "3.jpg"); 00031 ftp.getfile("/ftp/4.jpg", "4.jpg"); 00032 00033 ftp.mkdir("FTP Test"); 00034 00035 ftp.cd("FTP Test"); 00036 00037 ftp.putfile("/ftp/1.jpg", "1.jpg"); 00038 ftp.putfile("/ftp/2.jpg", "2.jpg"); 00039 ftp.putfile("/ftp/3.jpg", "3.jpg"); 00040 ftp.putfile("/ftp/4.jpg", "4.jpg"); 00041 00042 printf("FTPServer dir...\r\n"); 00043 ftp.dir(); 00044 printf("FTPServer ls...\r\n"); 00045 ftp.ls(); 00046 00047 ftp.cd("/"); 00048 00049 printf("FTPServer dir...\r\n"); 00050 ftp.dir(); 00051 printf("FTPServer ls...\r\n"); 00052 ftp.ls(); 00053 00054 ftp.quit(); 00055 } 00056 00057 00058 00059 00060 00061
Generated on Fri Jul 15 2022 07:19:26 by
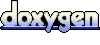