FTPClient
Dependents: FTPClient_example FTP_TESTClient
FTPClient.h
00001 #ifndef FTP_CLIENT_H 00002 #define FTP_CLIENT_H 00003 #include "mbed.h" 00004 #include "SDFileSystem.h" 00005 #define MAX_SS 256 00006 /** FTPClient class. 00007 * Used file transfer with FTPServer like ALFTP(http://software.altools.co.kr/ko-kr/closed.html) 00008 * This test was completed in ALFTP 00009 */ 00010 class FTPClient{ 00011 public: 00012 /** Create FTPClient instance */ 00013 FTPClient(PinName mosi, PinName miso, PinName sclk, PinName ssel, const char* root); 00014 ~FTPClient() {}; 00015 00016 /** Connect to FTPServer 00017 * 00018 * @param FTPServer IP, FTPServer PORT, FTPServer login ID, FTPServer login PASS 00019 * @returns 00020 * 1 on success, 00021 * 0 on open error 00022 */ 00023 bool open(char* ip, int port, char* id, char* pass); 00024 00025 /** Get file from FTPServer 00026 * 00027 * @param My file name, FTPServer file name 00028 * @returns 00029 * 1 on success, 00030 * 0 on getfile error 00031 */ 00032 bool getfile(char* myfilename, char* filename); 00033 00034 /** Put file to FTPServer 00035 * 00036 * @param My file name, FTPServer file name 00037 * @returns 00038 * 1 on success, 00039 * 0 on putfile error 00040 */ 00041 bool putfile(char* myfilename, char* filename); 00042 00043 /** View FTPServer directory 00044 * 00045 * @param 00046 * @returns 00047 * 1 on success, 00048 * 0 on dir error 00049 */ 00050 bool dir(); 00051 00052 /** View FTPServer directory 00053 * 00054 * @param 00055 * @returns 00056 * 1 on success, 00057 * 0 on ls error 00058 */ 00059 bool ls(); 00060 00061 /** Delete FTPServer file 00062 * 00063 * @param FTPServer file name 00064 * @returns 00065 * 1 on success, 00066 * 0 on delete error 00067 */ 00068 bool fdelete(char* filename); 00069 00070 /** Make FTPServer directory 00071 * 00072 * @param FTPServer directory name 00073 * @returns 00074 * 1 on success, 00075 * 0 on mkdir error 00076 */ 00077 bool mkdir(char* dirname); 00078 00079 /** Change current FTPServer directory 00080 * 00081 * @param FTPServer directory name 00082 * @returns 00083 * 1 on success, 00084 * 0 on mkdir error 00085 */ 00086 bool cd(char* dirname); 00087 00088 /** Disconnect from FTPServer 00089 * 00090 * @param 00091 * @returns 00092 * 1 on success, 00093 * 0 on Disconnect error 00094 */ 00095 bool quit(); 00096 00097 private: 00098 TCPSocketConnection* FTPClientControlSock; 00099 TCPSocketConnection* FTPClientDataSock; 00100 00101 bool blogin; 00102 bool bopenflag; 00103 bool brfileflag; 00104 bool bsfileflag; 00105 bool bdirflag; 00106 bool blsflag; 00107 bool bfdeleteflag; 00108 bool bmkdirflag; 00109 bool bcdflag; 00110 bool bquitflag; 00111 00112 char ftpServer_data_ip_addr[4]; 00113 char ftpServer_data_ip_addr_str[20]; 00114 int remote_port; 00115 00116 char rbuf[256]; 00117 char sbuf[256]; 00118 00119 int remain_datasize; 00120 int i; 00121 int remain_filesize; 00122 int send_byte; 00123 int size; 00124 FILE *fp; 00125 SDFileSystem _SDFileSystem; 00126 00127 int pportc(char * arg); 00128 }; 00129 #endif
Generated on Sat Jul 23 2022 03:17:03 by
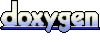