FTPClient
Dependents: FTPClient_example FTP_TESTClient
FTPClient.cpp
00001 #include "mbed.h" 00002 #include "EthernetInterface.h" 00003 #include "FTPClient.h" 00004 //#define DEBUG 00005 FTPClient::FTPClient(PinName mosi, PinName miso, PinName sclk, PinName ssel, const char* root) : _SDFileSystem(mosi, miso, sclk, ssel, root){ 00006 blogin = false; 00007 bopenflag = false; 00008 brfileflag = false; 00009 bdirflag = false; 00010 blsflag = false; 00011 bfdeleteflag = false; 00012 bmkdirflag = false; 00013 bcdflag = false; 00014 bquitflag = false; 00015 } 00016 bool FTPClient::open(char* ip, int port, char* id, char* pass){ 00017 00018 FTPClientControlSock = new TCPSocketConnection; 00019 FTPClientDataSock = new TCPSocketConnection; 00020 00021 #if 0 00022 do{ 00023 FTPClientControlSock->connect(ip, port); 00024 }while(!FTPClientControlSock->is_connected()); 00025 #endif 00026 #if 1 00027 while (FTPClientControlSock->connect(ip, port) < 0) { 00028 #ifdef DEBUG 00029 printf("Unable to connect to (%s) on port (%d)\r\n", ip, port); 00030 #endif 00031 wait(1); 00032 } 00033 #endif 00034 00035 while(!blogin){ 00036 size = FTPClientControlSock->receive(rbuf, sizeof(rbuf)); 00037 if(size > 0){ 00038 #ifdef DEBUG 00039 printf("Received message from server: %s\r\n", rbuf); 00040 #endif 00041 if (!strncmp(rbuf, "220", 3)){ 00042 printf("%s\r\n", rbuf); 00043 sprintf(sbuf, "user %s\r\n", id); 00044 FTPClientControlSock->send(sbuf, strlen(sbuf)); 00045 } 00046 else if (!strncmp(rbuf, "331", 3)){ 00047 sprintf(sbuf, "pass %s\r\n", pass); 00048 FTPClientControlSock->send(sbuf, strlen(sbuf)); 00049 } 00050 else if (!strncmp(rbuf, "230", 3)){ 00051 blogin = true; 00052 } 00053 else{ 00054 blogin = true; 00055 } 00056 } 00057 } 00058 return 1; 00059 } 00060 00061 bool FTPClient::getfile(char* myfilename, char* filename){ 00062 00063 if(blogin){ 00064 00065 sprintf(sbuf, "pasv\r\n"); 00066 FTPClientControlSock->send(sbuf, strlen(sbuf)); 00067 00068 while(!brfileflag){ 00069 size = FTPClientControlSock->receive(rbuf, sizeof(rbuf)); 00070 if(size > 0){ 00071 #ifdef DEBUG 00072 printf("Received message from server: %s\r\n", rbuf); 00073 #endif 00074 if (!strncmp(rbuf, "150", 3)){ 00075 fp = fopen(myfilename, "w"); 00076 #ifdef DEBUG 00077 printf("myfilename : %s\r\n", myfilename); 00078 #endif 00079 while(true){ 00080 memset(rbuf, 0, sizeof(rbuf)); 00081 remain_datasize = FTPClientDataSock->receive(rbuf, sizeof(rbuf)); 00082 #ifdef DEBUG 00083 printf("remain_datasize : %d\r\n", remain_datasize); 00084 #endif 00085 if(remain_datasize>0){ 00086 for (i = 0; i < remain_datasize; i++) { 00087 //printf("%c", rbuf[i]); 00088 fprintf(fp, "%c", rbuf[i]); 00089 } 00090 #ifdef DEBUG 00091 printf("#"); 00092 #endif 00093 } 00094 else{ 00095 brfileflag = true; 00096 fclose(fp); 00097 FTPClientDataSock->close(); 00098 break; 00099 } 00100 } 00101 } 00102 else if (!strncmp(rbuf, "227", 3)){ 00103 pportc(rbuf); 00104 00105 #if 1 00106 while (FTPClientDataSock->connect(ftpServer_data_ip_addr_str, remote_port) < 0) { 00107 #ifdef DEBUG 00108 printf("Unable to connect to (%s) on port (%d)\r\n", ftpServer_data_ip_addr_str, remote_port); 00109 #endif 00110 wait(1); 00111 } 00112 #endif 00113 00114 #if 0 00115 do{ 00116 FTPClientDataSock->connect(ftpServer_data_ip_addr_str, remote_port); 00117 }while(!FTPClientDataSock->is_connected()); 00118 #endif 00119 sprintf(sbuf, "retr %s\r\n", filename); 00120 FTPClientControlSock->send(sbuf, strlen(sbuf)); 00121 } 00122 } 00123 } 00124 brfileflag = false; 00125 return 1; 00126 } 00127 else return 0; 00128 } 00129 bool FTPClient::putfile(char* myfilename, char* filename){ 00130 00131 if(blogin){ 00132 00133 sprintf(sbuf, "pasv\r\n"); 00134 FTPClientControlSock->send(sbuf, strlen(sbuf)); 00135 00136 while(!bsfileflag){ 00137 size = FTPClientControlSock->receive(rbuf, sizeof(rbuf)); 00138 if(size > 0){ 00139 #ifdef DEBUG 00140 printf("Received message from server: %s\r\n", rbuf); 00141 #endif 00142 if (!strncmp(rbuf, "150", 3)){ 00143 fp = fopen(myfilename, "r"); 00144 fseek(fp, 0, SEEK_END); // seek to end of file 00145 remain_filesize = ftell(fp); // get current file pointer 00146 fseek(fp, 0, SEEK_SET); // seek back to beginning of file 00147 do{ 00148 memset(sbuf, 0, sizeof(sbuf)); 00149 if(remain_filesize > MAX_SS) 00150 send_byte = MAX_SS; 00151 else 00152 send_byte = remain_filesize; 00153 fread (sbuf, 1, send_byte, fp); 00154 FTPClientDataSock->send(sbuf, send_byte); 00155 remain_filesize -= send_byte; 00156 #ifdef DEBUG 00157 printf("#"); 00158 #endif 00159 }while(remain_filesize!=0); 00160 fclose(fp); 00161 bsfileflag = true; 00162 FTPClientDataSock->close(); 00163 break; 00164 } 00165 else if (!strncmp(rbuf, "227", 3)){ 00166 pportc(rbuf); 00167 #if 0 00168 do{ 00169 FTPClientDataSock->connect(ftpServer_data_ip_addr_str, remote_port); 00170 }while(!FTPClientDataSock->is_connected()); 00171 #endif 00172 00173 #if 1 00174 while (FTPClientDataSock->connect(ftpServer_data_ip_addr_str, remote_port) < 0) { 00175 #ifdef DEBUG 00176 printf("Unable to connect to (%s) on port (%d)\r\n", ftpServer_data_ip_addr_str, remote_port); 00177 #endif 00178 wait(1); 00179 } 00180 #endif 00181 sprintf(sbuf, "stor %s\r\n", filename); 00182 FTPClientControlSock->send(sbuf, strlen(sbuf)); 00183 } 00184 } 00185 } 00186 bsfileflag = false; 00187 return 1; 00188 } 00189 else return 0; 00190 } 00191 bool FTPClient::dir(){ 00192 00193 if(blogin){ 00194 00195 sprintf(sbuf, "pasv\r\n"); 00196 FTPClientControlSock->send(sbuf, strlen(sbuf)); 00197 00198 while(!bdirflag){ 00199 size = FTPClientControlSock->receive(rbuf, sizeof(rbuf)); 00200 if(size > 0){ 00201 #ifdef DEBUG 00202 printf("Received message from server: %s\r\n", rbuf); 00203 #endif 00204 if (!strncmp(rbuf, "150", 3)){ 00205 while(true){ 00206 memset(rbuf, 0, sizeof(rbuf)); 00207 size = FTPClientDataSock->receive(rbuf, sizeof(rbuf)); 00208 rbuf[size] = '\0'; 00209 if(size>0){ 00210 printf("%s", rbuf); 00211 } 00212 else{ 00213 bdirflag = true; 00214 FTPClientDataSock->close(); 00215 break; 00216 } 00217 } 00218 } 00219 else if (!strncmp(rbuf, "227", 3)){ 00220 pportc(rbuf); 00221 #if 0 00222 do{ 00223 FTPClientDataSock->connect(ftpServer_data_ip_addr_str, remote_port); 00224 }while(!FTPClientDataSock->is_connected()); 00225 #endif 00226 #if 1 00227 while (FTPClientDataSock->connect(ftpServer_data_ip_addr_str, remote_port) < 0) { 00228 #ifdef DEBUG 00229 printf("Unable to connect to (%s) on port (%d)\r\n", ftpServer_data_ip_addr_str, remote_port); 00230 #endif 00231 wait(1); 00232 } 00233 #endif 00234 sprintf(sbuf, "list\r\n"); 00235 FTPClientControlSock->send(sbuf, strlen(sbuf)); 00236 } 00237 } 00238 } 00239 bdirflag = false; 00240 return 1; 00241 } 00242 else return 0; 00243 } 00244 00245 bool FTPClient::ls(){ 00246 00247 if(blogin){ 00248 00249 sprintf(sbuf, "pasv\r\n"); 00250 FTPClientControlSock->send(sbuf, strlen(sbuf)); 00251 00252 while(!blsflag){ 00253 size = FTPClientControlSock->receive(rbuf, sizeof(rbuf)); 00254 if(size > 0){ 00255 #ifdef DEBUG 00256 printf("Received message from server: %s\r\n", rbuf); 00257 #endif 00258 if (!strncmp(rbuf, "150", 3)){ 00259 while(true){ 00260 memset(rbuf, 0, sizeof(rbuf)); 00261 size = FTPClientDataSock->receive(rbuf, sizeof(rbuf)); 00262 rbuf[size] = '\0'; 00263 if(size>0){ 00264 printf("%s", rbuf); 00265 } 00266 else{ 00267 blsflag = true; 00268 FTPClientDataSock->close(); 00269 break; 00270 } 00271 } 00272 } 00273 else if (!strncmp(rbuf, "227", 3)){ 00274 pportc(rbuf); 00275 #if 0 00276 do{ 00277 FTPClientDataSock->connect(ftpServer_data_ip_addr_str, remote_port); 00278 }while(!FTPClientDataSock->is_connected()); 00279 #endif 00280 #if 1 00281 while (FTPClientDataSock->connect(ftpServer_data_ip_addr_str, remote_port) < 0) { 00282 #ifdef DEBUG 00283 printf("Unable to connect to (%s) on port (%d)\r\n", ftpServer_data_ip_addr_str, remote_port); 00284 #endif 00285 wait(1); 00286 } 00287 #endif 00288 sprintf(sbuf, "nlst\r\n"); 00289 FTPClientControlSock->send(sbuf, strlen(sbuf)); 00290 } 00291 } 00292 } 00293 blsflag = false; 00294 return 1; 00295 } 00296 else return 0; 00297 } 00298 00299 bool FTPClient::fdelete(char* filename){ 00300 00301 if(blogin){ 00302 00303 sprintf(sbuf, "dele %s\r\n", filename); 00304 FTPClientControlSock->send(sbuf, strlen(sbuf)); 00305 00306 while(!bfdeleteflag){ 00307 size = FTPClientControlSock->receive(rbuf, sizeof(rbuf)); 00308 if(size > 0){ 00309 #ifdef DEBUG 00310 printf("Received message from server: %s\r\n", rbuf); 00311 #endif 00312 if (!strncmp(rbuf, "250", 3)){ 00313 bfdeleteflag = true; 00314 } 00315 else { 00316 return 0; 00317 } 00318 } 00319 } 00320 bfdeleteflag = false; 00321 return 1; 00322 } 00323 else return 0; 00324 } 00325 bool FTPClient::mkdir(char* dirname){ 00326 00327 if(blogin){ 00328 00329 sprintf(sbuf, "xmkd %s\r\n", dirname); 00330 FTPClientControlSock->send(sbuf, strlen(sbuf)); 00331 00332 while(!bmkdirflag){ 00333 size = FTPClientControlSock->receive(rbuf, sizeof(rbuf)); 00334 if(size > 0){ 00335 #ifdef DEBUG 00336 printf("Received message from server: %s\r\n", rbuf); 00337 #endif 00338 if (!strncmp(rbuf, "257", 3)){ 00339 bmkdirflag = true; 00340 } 00341 else { 00342 return 0; 00343 } 00344 } 00345 } 00346 bmkdirflag = false; 00347 return 1; 00348 } 00349 else return 0; 00350 } 00351 bool FTPClient::cd(char* dirname){ 00352 00353 if(blogin){ 00354 00355 sprintf(sbuf, "cwd %s\r\n", dirname); 00356 FTPClientControlSock->send(sbuf, strlen(sbuf)); 00357 00358 while(!bcdflag){ 00359 size = FTPClientControlSock->receive(rbuf, sizeof(rbuf)); 00360 if(size > 0){ 00361 #ifdef DEBUG 00362 printf("Received message from server: %s\r\n", rbuf); 00363 #endif 00364 if (!strncmp(rbuf, "250", 3)){ 00365 bcdflag = true; 00366 } 00367 else { 00368 return 0; 00369 } 00370 } 00371 } 00372 bcdflag = false; 00373 return 1; 00374 } 00375 else return 0; 00376 } 00377 bool FTPClient::quit(){ 00378 00379 if(blogin){ 00380 00381 sprintf(sbuf, "quit \r\n"); 00382 FTPClientControlSock->send(sbuf, strlen(sbuf)); 00383 00384 while(!bquitflag){ 00385 size = FTPClientControlSock->receive(rbuf, sizeof(rbuf)); 00386 if(size > 0){ 00387 #ifdef DEBUG 00388 printf("Received message from server: %s\r\n", rbuf); 00389 #endif 00390 if (!strncmp(rbuf, "250", 3)){ 00391 printf("%s\r\n", rbuf); 00392 bquitflag = true; 00393 } 00394 else { 00395 return 0; 00396 } 00397 } 00398 } 00399 00400 bquitflag = false; 00401 blogin = false; 00402 delete FTPClientControlSock; 00403 delete FTPClientDataSock; 00404 00405 return 1; 00406 } 00407 else return 0; 00408 } 00409 int FTPClient::pportc(char * arg) 00410 { 00411 char* tok=0; 00412 00413 strtok(arg,"("); 00414 for (i = 0; i < 4; i++) 00415 { 00416 if(i==0) tok = strtok(NULL,",\r\n"); 00417 else tok = strtok(NULL,","); 00418 ftpServer_data_ip_addr[i] = (uint8_t)atoi(tok); 00419 if (!tok){ 00420 #ifndef DEBUG 00421 printf("bad pport : %s\r\n", arg); 00422 #endif 00423 return -1; 00424 } 00425 } 00426 remote_port = 0; 00427 for (i = 0; i < 2; i++){ 00428 tok = strtok(NULL,",\r\n"); 00429 remote_port <<= 8; 00430 remote_port += atoi(tok); 00431 if (!tok){ 00432 #ifdef DEBUG 00433 printf("bad pport : %s\r\n", arg); 00434 #endif 00435 return -1; 00436 } 00437 } 00438 sprintf(ftpServer_data_ip_addr_str, "%d.%d.%d.%d", ftpServer_data_ip_addr[0], ftpServer_data_ip_addr[1], ftpServer_data_ip_addr[2], ftpServer_data_ip_addr[3]); 00439 return 0; 00440 } 00441 00442 00443 00444
Generated on Sat Jul 23 2022 03:17:03 by
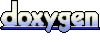