Library for communicating with a Wii classic controller using the I2C bus.
Dependents: WiiClassicControllerTest
WiiClassicController.cpp
00001 /* 00002 * SOURCE FILE : WiiClassicController.cpp 00003 * 00004 * Definition of class WiiClassicController. 00005 * Allows use of a Wii classic controller using an I2C bus. 00006 * 00007 */ 00008 00009 #include "WiiClassicController.h" 00010 00011 // Delay to slow things down. Doesn;t work without this. 00012 #define WAIT_TIME ((float)0.01 ) // in seconds 00013 00014 /** Constructor. 00015 * @param sda pin to use for SDA. 00016 * @param scl pin to use for SCL. 00017 */ 00018 WiiClassicController::WiiClassicController( PinName sda, PinName scl ) : 00019 controllerPort( sda, scl ), 00020 initialised( false ) 00021 { 00022 } 00023 00024 /** Destructor. 00025 */ 00026 WiiClassicController::~WiiClassicController() { 00027 } 00028 00029 /** Read from the controller. 00030 * @returns true on success, false on failure. 00031 */ 00032 bool WiiClassicController::Read( void ) { 00033 // Don't expect client to remember to send an init to the nunchuck 00034 // so do it for them here. 00035 if( ! initialised ) { 00036 initialised = ControllerInit(); 00037 } 00038 // Don't start reading if init failed 00039 return initialised && ControllerRead(); 00040 } 00041 00042 /** Initialise the controller. 00043 * @returns true on success, false on failure. 00044 */ 00045 bool WiiClassicController::ControllerInit( void ) { 00046 const UInt8 cmd[] = { CONTROLLER_REGADDR, 0x00 }; 00047 bool ok = ( controllerPort.write( CONTROLLER_ADDR, (const char*)cmd, sizeof(cmd) ) == 0 ); 00048 wait( WAIT_TIME ); 00049 return ok; 00050 } 00051 00052 /** Read from the controller, assuming it has been initialised. 00053 * @returns true on success, false on failure. 00054 */ 00055 bool WiiClassicController::ControllerRead() { 00056 bool ok = false; 00057 // write the address we want to read from 00058 const UInt8 cmd[] = { 0x00 }; 00059 if( controllerPort.write( CONTROLLER_ADDR, (const char*)cmd, sizeof(cmd) ) == 0 ) { 00060 // The Wii Classic Controller is non-standard I2C 00061 // and can't manage setting the read address and immediately supplying the data 00062 // so wait a bit. 00063 wait( WAIT_TIME ); 00064 if( controllerPort.read( CONTROLLER_ADDR, (char*)readBuf, sizeof(readBuf) ) == 0 ) { 00065 for( int i = 0; i < CONTROLLER_READLEN; ++i ) { 00066 readBuf[ i ] = Decode( readBuf[ i ] ); 00067 } 00068 ok = true; 00069 } 00070 } 00071 // wait( WAIT_TIME ); 00072 return ok; 00073 }
Generated on Tue Jul 12 2022 20:58:08 by
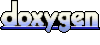