
A test program which communicates with a Gameduino shield and writes some text on a VGA display.
Dependencies: Gameduino CommonTypes mbed
main.cpp
00001 #include "mbed.h" 00002 #include "Gameduino.h" 00003 00004 int main() { 00005 // Make a digital output for use with Gameduino. 00006 DigitalOut cs( p8 ); 00007 // Initialise an SPI link for communications with Gameduino. 00008 // Use pin 5 for MOSI. 00009 // Use pin 6 for MISO. 00010 // Use pin 7 for SCK. 00011 SPI spi( p5, p6, p7 ); 00012 // 8MHz clock should be OK. 00013 spi.frequency( 8000000 ); 00014 // Set SPI format to use. 00015 // Use 8 bits per SPI frame. 00016 // Use SPI mode 0. 00017 spi.format( 8, 0 ); 00018 // Make a Gameduino and pass SPI link and digital output for chip select. 00019 Gameduino gd( &spi, &cs ); 00020 // Reset the Gameduino. 00021 gd.begin(); 00022 // Lets have a default ASCII character set. 00023 gd.ascii(); 00024 // Write something to character memory. 00025 gd.__wstart( Gameduino::RAM_PIC ); 00026 for( UInt8 c = 'A'; c <= 'Z'; ++c ) { 00027 gd.__tr8( c ); 00028 } 00029 gd.__end(); 00030 // Test copy method. 00031 UInt8 copyData[] = "HELLO"; 00032 gd.copy( Gameduino::RAM_PIC + 64, copyData, 5 ); 00033 // Test putstr method. 00034 gd.putstr( 3, 10, "Ambidextrous!" ); 00035 // Finished with Gameduino. 00036 gd.end(); 00037 }
Generated on Wed Jul 20 2022 14:01:48 by
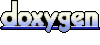