My implementation of VT100 ESC-sequence utility
Dependents: test_vt100 maze_vt100_MMA8451Q funcgen test_MAG3110 ... more
vt100.cpp
00001 #include "serial_api.h" 00002 #include "vt100.h" 00003 #include "mbed.h" 00004 00005 #define BLACK 0 00006 #define RED 1 00007 #define GREEN 2 00008 #define YELLOW 3 00009 #define BLUE 4 00010 #define PURPLE 5 00011 #define CIAN 6 00012 #define WHITE 7 00013 00014 char ESC = '\033' ; 00015 00016 vt100::vt100(int baud) 00017 { 00018 extern serial_t stdio_uart ; 00019 serial_baud(&stdio_uart, baud) ; 00020 } 00021 00022 vt100::~vt100() 00023 { 00024 } 00025 00026 void vt100::cls(void) 00027 { 00028 printf("%c[2J", ESC) ; 00029 locate(1,1) ; 00030 } 00031 00032 void vt100::locate(int x, int y) 00033 { 00034 printf("%c[%d;%dH",ESC,y,x) ; 00035 } 00036 00037 void vt100::putChar(int x, int y, char c) 00038 { 00039 locate(x,y) ; 00040 printf("%c",c) ; 00041 } 00042 00043 void vt100::putStr(int x, int y, char *str) 00044 { 00045 locate(x,y) ; 00046 printf("%s", str) ; 00047 } 00048 00049 void vt100::line(int x1, int y1, int x2, int y2, char c) 00050 { 00051 int x, y, dx, dy, w, h, step ; 00052 dx = x2 - x1 ; 00053 dy = y2 - y1 ; 00054 w = (dx >= 0)? dx : -dx ; 00055 h = (dy >= 0)? dy : -dy ; 00056 00057 if (dx == 0) { /* vertical line */ 00058 step = (dy >= 0) ? 1 : -1 ; 00059 for (y = 0 ; y <= h ; y++) { 00060 putChar(x1, y1 + (step * y), c) ; 00061 } 00062 } else if (dy == 0) { /* Horizontal line */ 00063 step = (dx >= 0) ? 1 : -1 ; 00064 for (x = 0 ; x <= w ; x++) { 00065 putChar(x1 + (step * x), y1, c) ; 00066 } 00067 } else { 00068 if (w >= h) { /* use x as step */ 00069 step = (dx >= 0) ? 1 : -1 ; 00070 for (x = 0 ; x <= w ; x++ ) { 00071 putChar( x1 + step*x, y1 + ((2*x+1) * dy)/(2 * w), c) ; 00072 } 00073 } else { /* use y as step */ 00074 step = (dy >= 0) ? 1 : -1 ; 00075 for (y = 0 ; y <= h ; y++ ) { 00076 putChar( x1 + ((2*y+1) * dx)/(2*h), y1 + step*y, c) ; 00077 } 00078 } 00079 } 00080 } 00081 00082 /**************************************************** 00083 * frame(x1, y1, x2, y2) 00084 * draw textual frame 00085 * (x1,y1) (x2,y1) 00086 * +--------------------------+ 00087 * | | 00088 * +--------------------------+ 00089 * (x1,y2) (x2,y2) 00090 */ 00091 void vt100::frame(int x1, int y1, int x2, int y2) 00092 { 00093 int tmp ; 00094 if (x1 > x2) { 00095 tmp = x1 ; 00096 x1 = x2 ; 00097 x2 = tmp ; 00098 } 00099 if (y1 > y2) { 00100 tmp = y1 ; 00101 y1 = y2 ; 00102 y2 = tmp ; 00103 } 00104 putChar(x1, y1, '+') ; 00105 line(x1+1,y1,x2-1,y1, '-') ; 00106 putChar(x2, y1, '+') ; 00107 line(x2,y1+1,x2,y2-1, '|') ; 00108 putChar(x2, y2, '+') ; 00109 line(x2-1,y2,x1+1,y2, '-') ; 00110 putChar(x1, y2, '+') ; 00111 line(x1,y2-1,x1,y1+1, '|') ; 00112 } 00113 00114 /*************************************************** 00115 * circle(x, y, r, c) 00116 * Based on Jack Elton Bresenham's 00117 * Midpoint circle algorithm. 00118 * http://en.wikipedia.org/wiki/Midpoint_circle_algorithm 00119 */ 00120 void vt100::circle(int x0, int y0, int r, char c) 00121 { 00122 int f = 1 - r ; 00123 int dFx = 1 ; 00124 int dFy = -2 * r ; 00125 int x = 0 ; 00126 int y = r ; 00127 00128 putChar(x0, y0 + r, c) ; 00129 putChar(x0, y0 - r, c) ; 00130 putChar(x0 + 2*r, y0, c) ; 00131 putChar(x0 - 2*r, y0, c) ; 00132 00133 while(x < y) { 00134 if (f >= 0) { 00135 y-- ; 00136 dFy += 2 ; 00137 f += dFy ; 00138 } 00139 x++ ; 00140 dFx += 2 ; 00141 f += dFx ; 00142 putChar(x0 + 2*x, y0 + y, c) ; 00143 putChar(x0 - 2*x, y0 + y, c) ; 00144 putChar(x0 + 2*x, y0 - y, c) ; 00145 putChar(x0 - 2*x, y0 - y, c) ; 00146 putChar(x0 + 2*y, y0 + x, c) ; 00147 putChar(x0 - 2*y, y0 + x, c) ; 00148 putChar(x0 + 2*y, y0 - x, c) ; 00149 putChar(x0 - 2*y, y0 - x, c) ; 00150 } 00151 } 00152 00153 int vt100::setFG(int newFG) 00154 { 00155 int oldFG = foreground ; 00156 printf("\033[3%dm", newFG) ; 00157 foreground = newFG ; 00158 return( oldFG ) ; 00159 } 00160 00161 int vt100::getFG() 00162 { 00163 return( foreground ) ; 00164 } 00165 00166 int vt100::setBG(int newBG) 00167 { 00168 int oldBG = background ; 00169 printf("\033[4%dm", newBG) ; 00170 return( oldBG ) ; 00171 } 00172 00173 int vt100::getBG() 00174 { 00175 return( background ) ; 00176 } 00177 00178 void vt100::black() 00179 { 00180 setFG( BLACK ) ; 00181 } 00182 00183 void vt100::red() 00184 { 00185 setFG( RED ) ; 00186 } 00187 00188 void vt100::green() 00189 { 00190 setFG( GREEN ) ; 00191 } 00192 00193 void vt100::yellow() 00194 { 00195 setFG( YELLOW ) ; 00196 } 00197 00198 void vt100::blue() 00199 { 00200 setFG( BLUE ) ; 00201 } 00202 00203 void vt100::purple() 00204 { 00205 setFG( PURPLE ) ; 00206 } 00207 00208 void vt100::cian() 00209 { 00210 setFG( CIAN ) ; 00211 } 00212 00213 void vt100::white() 00214 { 00215 setFG( WHITE ) ; 00216 }
Generated on Fri Jul 15 2022 08:24:33 by
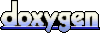