
Another short time hack. This one is for test the behavior of the spi module. Probably an Oscilloscope is required to check the wave.
main.cpp
00001 /** Test Spi a quick and dirty spi test program 00002 */ 00003 00004 #include "mbed.h" 00005 #include <string.h> 00006 #include <stdio.h> 00007 #include "MSS.h" 00008 00009 #if 0 00010 #define PIN_MOSI PTD2 00011 #define PIN_MISO PTD3 00012 #define PIN_SCLK PTD1 00013 #define PIN_CS_TSC PTA13 00014 #define PIN_TSC_INTR PTC9 00015 #define PIN_MSS_CS PTD0 00016 #endif 00017 00018 int freq = 1000000 ; 00019 int bits = 8 ; 00020 int mode = 0 ; 00021 int loop = 10 ; 00022 00023 SPI mySpi(PIN_MOSI, PIN_MISO, PIN_SCK) ; 00024 DigitalOut cs(PIN_INT0, 1) ; 00025 00026 typedef void (*func_ptr)(void) ; 00027 00028 void doHelp(void) ; 00029 void doStatus(void) ; 00030 void doFreq(void) ; 00031 void doMode(void) ; 00032 void doBit(void) ; 00033 void doWrite(void) ; 00034 void doRead(void) ; 00035 void doLoop(void) ; 00036 00037 typedef struct _cmd_func { 00038 char *name ; 00039 func_ptr func ; 00040 } cmd_func_type ; 00041 00042 cmd_func_type cmd_list[] = { 00043 {"help", doHelp}, 00044 {"status", doStatus}, 00045 {"freq", doFreq}, 00046 {"mode", doMode}, 00047 {"bit", doBit}, 00048 {"write", doWrite}, 00049 {"read", doRead}, 00050 {"loop", doLoop}, 00051 { 0, 0 } 00052 } ; 00053 00054 func_ptr getFunc(char *cmd) 00055 { 00056 int i = 0 ; 00057 while(cmd_list[i].name != 0) { 00058 if (strcmp(cmd, cmd_list[i].name) == 0) { 00059 return(cmd_list[i].func) ; ; 00060 } 00061 i++ ; 00062 } 00063 return(0) ; 00064 } 00065 00066 void doHello() 00067 { 00068 printf("=== spi test program ===\n\r") ; 00069 printf("please set your terminal program\n\r") ; 00070 printf("local echo on\n\r") ; 00071 printf("\n\r") ; 00072 } 00073 00074 int main() { 00075 char cmd[32] ; 00076 func_ptr func ; 00077 doHello() ; 00078 while(1) { 00079 printf("> ") ; 00080 scanf("%s", cmd) ; 00081 if ((func = getFunc(cmd)) != 0) { 00082 (*func)() ; 00083 } else { 00084 doHelp() ; 00085 } 00086 printf("\n\r") ; 00087 } 00088 } 00089 00090 void doHelp(void) 00091 { 00092 printf("=== spi test ===\n\r") ; 00093 printf("commands available\n\r") ; 00094 printf("help\n\r") ; 00095 printf("status\n\r") ; 00096 printf("freq freq_in_hz\n\r") ; 00097 printf("mode (0 | 1 | 2 | 3)\n\r") ; 00098 printf("bit (4 - 16)\n\r") ; 00099 printf("write value\n\r") ; 00100 printf("read\n\r") ; 00101 printf("loop number (set repeat number for read/write)\n\r") ; 00102 } 00103 00104 void doStatus(void) 00105 { 00106 printf("=== Status Report ===\n\r") ; 00107 printf("bits: %d\n\r", bits) ; 00108 printf("mode: %d\n\r", mode) ; 00109 printf("freq: %d Hz\n\r", freq) ; 00110 printf("loop: %d\n\r", loop) ; 00111 } 00112 00113 void doFreq(void) 00114 { 00115 int freq = 0 ; 00116 scanf("%d", &freq) ; 00117 printf("setting frequency to %d\n\r", freq) ; 00118 mySpi.frequency(freq) ; 00119 } 00120 00121 void doMode(void) 00122 { 00123 scanf("%d", &mode) ; 00124 printf("setting format(%d, %d)\n\r",bits, mode) ; 00125 mySpi.format(bits, mode) ; 00126 } 00127 00128 void doBit(void) 00129 { 00130 scanf("%d", &bits) ; 00131 printf("setting format(%d, %d)\n\r",bits, mode) ; 00132 mySpi.format(bits, mode) ; 00133 } 00134 00135 void doWrite(void) 00136 { 00137 int value, i ; 00138 scanf("%X", &value) ; 00139 printf("writing value 0x%X (%d) \n\r",value, value) ; 00140 cs = 0 ; 00141 for (i = 0 ; i < loop ; i++ ) { 00142 mySpi.write(value) ; 00143 } 00144 cs = 1 ; 00145 } 00146 00147 void doRead(void) 00148 { 00149 int dummy = 0 ; 00150 int i ; 00151 int value = 0 ; 00152 cs = 0 ; 00153 for (i = 0 ; i < loop ; i++ ) { 00154 value = mySpi.write(dummy) ; 00155 } 00156 cs = 1 ; 00157 printf("%d\n", value) ; 00158 } 00159 00160 void doLoop(void) 00161 { 00162 scanf("%d", &loop) ; 00163 printf("repeat number has been set to %d\n\r", loop) ; 00164 }
Generated on Thu Jul 14 2022 04:19:25 by
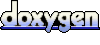