
test program for RTC8564
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "MSS.h" 00003 #include "RTC8564.h" 00004 00005 #define RTC8564_I2C_ADDRESS (0x51) 00006 00007 char *dayName[] = { 00008 "Sunday", 00009 "Monday", 00010 "Tuesday", 00011 "Wednesday", 00012 "Thursday", 00013 "Friday", 00014 "Saturday", 00015 0 00016 } ; 00017 00018 RTC8564 *rtc = 0 ; 00019 00020 void dumpData(uint8_t data[]) 00021 { 00022 int i ; 00023 for (i = 0 ; i < 0x10 ; i++ ) { 00024 printf("%02X ", data[i]) ; 00025 } 00026 printf("\n") ; 00027 } 00028 00029 void prompt(char *str) 00030 { 00031 printf("%s > ", str) ; 00032 fflush(stdout) ; 00033 } 00034 00035 void setTime(void) 00036 { 00037 int year, month, day, dayofweek ; 00038 int hour, minute, second ; 00039 rtc->stop() ; 00040 prompt("Enter Year") ; scanf("%d", &year) ; 00041 rtc->setYears(year-2000) ; 00042 00043 prompt("Enter Month") ; scanf("%d", &month) ; 00044 rtc->setMonths(month) ; 00045 00046 prompt("Enter Day") ; scanf("%d", &day) ; 00047 rtc->setDays(day) ; 00048 00049 prompt("Enter Day of week(0: Sun... 6: Sat)") ; 00050 scanf("%d", &dayofweek) ; 00051 rtc->setWeekdays( dayofweek ) ; 00052 00053 prompt("Enter hour (0..23)") ; scanf("%d", &hour) ; 00054 rtc->setHours(hour) ; 00055 00056 prompt("Enter min (0..59)") ; scanf("%d", &minute) ; 00057 rtc->setMinutes( minute ) ; 00058 00059 prompt("Enter sec (0..59)") ; scanf("%d", &second ) ; 00060 rtc->setSeconds( second ) ; 00061 00062 rtc->setMinuteAlarm(0x00) ; 00063 rtc->setHourAlarm(0x00) ; 00064 rtc->setDayAlarm(0x00) ; 00065 rtc->setWeekdayAlarm(0x00) ; 00066 rtc->setCLKOUTFrequency(0x00) ; 00067 rtc->setTimerControl(0x00) ; 00068 rtc->setTimer(0x00) ; 00069 00070 rtc->start() ; 00071 } 00072 00073 void printTime(void) 00074 { 00075 if (rtc) { 00076 printf("20%02d/%02d/%02d %s %02d:%02d:%02d\n", 00077 rtc->getYears(), 00078 rtc->getMonths(), 00079 rtc->getDays(), 00080 dayName[ rtc->getWeekdays() ], 00081 rtc->getHours(), 00082 rtc->getMinutes(), 00083 rtc->getSeconds() 00084 ) ; 00085 } 00086 } 00087 00088 int main() { 00089 rtc = new RTC8564(PIN_SDA, PIN_SCL, RTC8564_I2C_ADDRESS) ; 00090 printf("=== test RTC8564 for %s (%s) ===\n",BOARD_NAME, __DATE__) ; 00091 setTime() ; 00092 while(1){ 00093 printTime() ; 00094 wait(1) ; 00095 } 00096 }
Generated on Wed Aug 10 2022 11:52:14 by
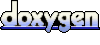