Yet another implementation of wave function generator
Embed:
(wiki syntax)
Show/hide line numbers
sinwave.h
00001 /** sinwave class 00002 * Copyright (c) 2014, 2015 Motoo Tanaka @ Design Methodology Lab 00003 * 00004 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00005 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00006 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00007 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00008 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00009 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00010 * THE SOFTWARE. 00011 */ 00012 00013 /** The sin_table.h was generated with the following c code 00014 * @code 00015 * #include <stdio.h> 00016 * #include <math.h> 00017 * 00018 * int main(int argc, char *argv[]) 00019 * { 00020 * long i ; 00021 * double value ; 00022 * double pi = 3.14159265359 ; 00023 * 00024 * printf("#ifndef _SIN_TABLE_H_\n") ; 00025 * printf("#define _SIN_TABLE_H_\n") ; 00026 * printf("#define SIN_ZERO 0x8000\n") ; 00027 * printf("#define NUM_SIN_TABLE 2500\n") ; 00028 * printf("unsigned short sin_table[] = {\n") ; 00029 * for (i = 0 ; i <= 2500 ; i++) { 00030 * value = sin(pi * i / 5000.0) ; 00031 * printf("0x%04X,", (int)((0x07FFF * value) + 0.5)) ; 00032 * if (((i+1) % 0x8) == 0) { 00033 * printf("\n") ; 00034 * } 00035 * } 00036 * printf("} ;\n") ; 00037 * printf("#endif\n") ; 00038 * } 00039 * @endcode 00040 */ 00041 #ifndef _SIN_WAVE_H_ 00042 #define _SIN_WAVE_H_ included 00043 #include "wave.h" 00044 00045 /** sinwave sine wave class 00046 */ 00047 class sinwave : public wave { 00048 public: 00049 /** constructor 00050 * 00051 * @param float v amplitude in voltage (0.0 ~ 3.28V for FRDM-KL25Z) 00052 * @param int f frequency in Hz (1 ~ 10000Hz) 00053 * @param int d duty, ignored for sinwave 00054 * @param int p phase in degree (0 ~ 359 degree) 00055 * @returns sinwave class instance 00056 */ 00057 sinwave(float v, int f, int d = 50, int p = 0) ; 00058 00059 /** destructor 00060 */ 00061 ~sinwave(void) ; 00062 00063 /** mutator for duty (ignored for sin) 00064 */ 00065 virtual void duty(int newvalue) ; 00066 00067 /** inspector for duty always returns 50 */ 00068 virtual int duty(void) ; 00069 00070 /** inspector for the value at _pos 00071 * 00072 * @returns int value at current position 00073 */ 00074 virtual int value(void) ; //returns current value 00075 00076 } ; 00077 00078 #endif /* _SIN_WAVE_H_ */
Generated on Wed Jul 13 2022 01:58:56 by
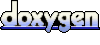