
SPI_Encoder_AMT203-V how to Note: Encoder was tested on the 3.3Vout
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 00002 // program used to read SPI 12 bit Encoder AMT203-V in steps of 16 00003 #include "mbed.h" 00004 00005 Serial pc(USBTX, USBRX); // tx, rx 00006 SPI EncoderSpi(p5, p6, p7);//Mosi Miso Sclk 00007 DigitalOut cs(p8); //Chip select 00008 00009 uint16_t EncoderByteData = 0; 00010 uint16_t Encoderposition_last = 0; 00011 uint8_t temp[2]; 00012 uint16_t Steps; 00013 void wait_ms(int us); 00014 00015 00016 uint8_t SPI_T (uint8_t SPITransmit)//Repetive SPI transmit sequence 00017 { 00018 uint8_t SPI_temp = 0; //vairable to hold recieved data 00019 cs=0; //select spi device 00020 SPI_temp = EncoderSpi.write(SPITransmit);//send and recieve 00021 cs=1;//deselect spi device 00022 return(SPI_temp); //return recieved byte 00023 } 00024 00025 void start() 00026 { 00027 uint8_t recieved = 0xA5;//just a temp vairable 00028 EncoderByteData = 0;//reset position vairable 00029 cs=0; 00030 SPI_T(0x10);//issue read command 00031 wait_ms(50);//give time to read. Timmig is critical 00032 00033 while (recieved != 0x10) //loop while encoder is not ready to send 00034 { 00035 recieved = SPI_T(0x00); //cleck again if encoder is still working 00036 wait_ms(1); //again,give time to read. Timmig is critical 00037 } 00038 00039 temp[0] = SPI_T(0x00); //Recieve MSB 00040 temp[1] = SPI_T(0x00); // recieve LSB 00041 cs=1; 00042 00043 temp[0] &=~ 0xF0;//mask out the first 4 bits 00044 EncoderByteData = temp[0] << 8; //shift MSB to correct EncoderByteData in EncoderByteData message 00045 EncoderByteData += temp[1]; // add LSB to EncoderByteData message to complete message 00046 wait_ms(1);//again,give time to read. Timmig is critical 00047 } 00048 00049 int main() 00050 { 00051 EncoderSpi.format(8,0); 00052 EncoderSpi.frequency(8000000); 00053 00054 while(true) 00055 { 00056 00057 if (EncoderByteData != Encoderposition_last) //if nothing has changed dont wast time sending position 00058 { 00059 Encoderposition_last = EncoderByteData ; //set last position to current position 00060 } 00061 else 00062 { 00063 start(); //if something has changed in position, catch it 00064 } 00065 Steps = EncoderByteData; 00066 //pc.printf("Data = %d \n", EncoderByteData ); //actual byte reading 00067 pc.printf("Data = %03d \n", Steps/16 ); 00068 } 00069 00070 }
Generated on Sun Jul 17 2022 17:00:54 by
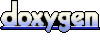