
test cli
Dependencies: mbed-os-example-mbed5-lorawan
Fork of Projet_de_bachelor_code by
trace_helper.cpp
00001 /** 00002 * Copyright (c) 2017, Arm Limited and affiliates. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 00018 /** 00019 * If we have tracing library available, we can see traces from within the 00020 * stack. The library could be made unavailable by removing FEATURE_COMMON_PAL 00021 * from the mbed_app.json to save RAM. 00022 */ 00023 #if defined(FEATURE_COMMON_PAL) 00024 00025 #include "platform/PlatformMutex.h" 00026 #include "mbed_trace.h" 00027 00028 /** 00029 * Local mutex object for synchronization 00030 */ 00031 static PlatformMutex mutex; 00032 00033 static void serial_lock(); 00034 static void serial_unlock(); 00035 00036 /** 00037 * Sets up trace for the application 00038 * Wouldn't do anything if the FEATURE_COMMON_PAL is not added 00039 * or if the trace is disabled using mbed_app.json 00040 */ 00041 void setup_trace() 00042 { 00043 // setting up Mbed trace. 00044 mbed_trace_mutex_wait_function_set(serial_lock); 00045 mbed_trace_mutex_release_function_set(serial_unlock); 00046 mbed_trace_init(); 00047 } 00048 00049 /** 00050 * Lock provided for serial printing used by trace library 00051 */ 00052 static void serial_lock() 00053 { 00054 mutex.lock(); 00055 } 00056 00057 /** 00058 * Releasing lock provided for serial printing used by trace library 00059 */ 00060 static void serial_unlock() 00061 { 00062 mutex.unlock(); 00063 } 00064 00065 #else 00066 00067 void setup_trace() 00068 { 00069 } 00070 00071 #endif 00072
Generated on Thu Jul 14 2022 11:45:28 by
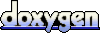