Sigfox library with integration of ATParser
Embed:
(wiki syntax)
Show/hide line numbers
Sigfox.h
00001 /* Copyright (c) 2015 ARM Limited 00002 * 00003 * Licensed under the Apache License, Version 2.0 (the "License"); 00004 * you may not use this file except in compliance with the License. 00005 * You may obtain a copy of the License at 00006 * 00007 * http://www.apache.org/licenses/LICENSE-2.0 00008 * 00009 * Unless required by applicable law or agreed to in writing, software 00010 * distributed under the License is distributed on an "AS IS" BASIS, 00011 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00012 * See the License for the specific language governing permissions and 00013 * limitations under the License. 00014 * 00015 * @section DESCRIPTION 00016 * 00017 * Send command to Sigfox 00018 * 00019 */ 00020 #ifndef SIGFOX_H 00021 #define SIGFOX_H 00022 00023 #include "ATParser.h" 00024 #include "mbed.h" 00025 00026 // https://www.disk91.com/2016/technology/sigfox/test-of-sigfox-wisol-wssfm10r1-module/ 00027 00028 typedef struct _sigfoxvoltage { 00029 double current; 00030 double last; 00031 } sigfoxvoltage_t; 00032 00033 class Sigfox 00034 { 00035 private: 00036 ATParser *_at; 00037 char ID[9]; 00038 char PAC[17]; 00039 00040 public: 00041 Sigfox(ATParser &at) : _at(&at) {}; 00042 00043 bool ready(); 00044 00045 bool send(const char *data, char* response=NULL); 00046 /** 00047 * true = public 00048 * false = private 00049 */ 00050 bool setKey(bool type); 00051 00052 bool setPower(uint8_t power=15); 00053 00054 bool setPowerMode(uint8_t power); 00055 00056 void wakeup(DigitalInOut sig_rst, float time=0.2); 00057 00058 bool saveConfig(); 00059 00060 char *getID(); 00061 00062 char *getPAC(); 00063 00064 sigfoxvoltage_t getVoltages(); 00065 00066 float getTemperature(); 00067 }; 00068 00069 #endif /* SIGFOX_H */
Generated on Wed Jul 20 2022 06:34:23 by
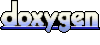