Basic Implementation of JSON. Capable of create a JSON string and parse a JSON string. Uses the "lazy" JSON implementation. Incapable of modify and delete variables from the objects. Contains 2 objects: JSONArray and JSONObject. Inspired in Java-Android implementation of JSON. Version 0.5.
oJSON.h
00001 /* Copyright (c) 2012 Otavio Netto Zani, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 00020 00021 #include "mbed.h" 00022 #include <string> 00023 #include <sstream> 00024 00025 //CLASSES 00026 00027 class JSONArray; 00028 00029 class JSONObject{ 00030 00031 int nValues; 00032 string* labels; 00033 string* values; 00034 00035 public: 00036 00037 JSONObject (); 00038 JSONObject (string); 00039 JSONObject (char*); 00040 00041 int getInt (string); 00042 int getInt (char*); 00043 float getFloat (string); 00044 float getFloat (char*); 00045 string getString (string); 00046 string getString (char*); 00047 JSONArray getArray (string); 00048 JSONArray getArray (char*); 00049 JSONObject getObject (string); 00050 JSONObject getObject (char*); 00051 bool getBoolean(char*); 00052 bool getBoolean(string); 00053 bool isNull(char*); 00054 bool isNull(string); 00055 00056 string toString(); 00057 00058 void add(string, string); 00059 void add(string, int); 00060 void add(string, float); 00061 void add(string, bool); 00062 void add(string, JSONObject); 00063 void add(string, JSONArray); 00064 00065 private: 00066 void criar(string); 00067 00068 }; 00069 00070 class JSONArray{ 00071 00072 int nValues; 00073 string *values; 00074 00075 00076 public: 00077 JSONArray (); 00078 JSONArray (string); 00079 JSONArray (char*); 00080 00081 int getInt(int); 00082 float getFloat (int); 00083 string getString (int); 00084 JSONObject getObject (int); 00085 JSONArray getArray (int); 00086 bool getBoolean(int); 00087 bool isNull(int); 00088 00089 string toString(); 00090 00091 void add(string); 00092 void add(int); 00093 void add(float); 00094 void add(bool); 00095 void add(JSONObject); 00096 void add(JSONArray); 00097 00098 private: 00099 void criar(string); 00100 00101 }; 00102 00103 int numberOfChars(char, string); 00104 string clear(string); 00105 string *explode(char, string);
Generated on Thu Jul 14 2022 01:08:59 by
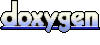