
USB mouse example for KL25 Freedom board.
Dependencies: MMA8451Q TSI USBDevice mbed
main.cpp
00001 #include "mbed.h" 00002 #include "MMA8451Q.h" 00003 #include "USBMouse.h" 00004 #include "TSISensor.h" 00005 00006 #define MMA8451_I2C_ADDRESS (0x1d<<1) 00007 00008 #define DEBUGCONSOLE 1 /* 9600bd serial to OpenSWD COM port */ 00009 00010 USBMouse mouse; 00011 Serial pc(USBTX,USBRX); 00012 00013 void mbutton_detect( float percent) 00014 { 00015 #define LBTN_MIN 75 00016 #define LBTN_MAX 100 00017 #define RBTN_MIN 1 00018 #define RBTN_MAX 30 00019 #define DBL_MIN 35 00020 #define DBL_MAX 70 00021 00022 int pos = percent * 100; 00023 static bool ltouchflg = false; 00024 static bool rtouchflg = false; 00025 00026 /* left button */ 00027 if((pos >= DBL_MIN)) 00028 { 00029 if( ltouchflg == false ) 00030 { 00031 mouse.press(MOUSE_LEFT); 00032 ltouchflg = true; 00033 #if DEBUGCONSOLE 00034 pc.printf("ltouch \n"); 00035 #endif 00036 } 00037 } 00038 00039 /* right button */ 00040 if((pos <= DBL_MAX) && (pos > RBTN_MIN)) 00041 { 00042 if( rtouchflg == false ) 00043 { 00044 mouse.press(MOUSE_RIGHT); 00045 rtouchflg = true; 00046 #if DEBUGCONSOLE 00047 pc.printf("rtouch \n"); 00048 #endif 00049 } 00050 } 00051 00052 /* release left */ 00053 if( (pos < DBL_MIN)) 00054 { 00055 if( ltouchflg == true ) 00056 { 00057 mouse.release(MOUSE_LEFT); 00058 ltouchflg = false; 00059 #if DEBUGCONSOLE 00060 pc.printf("lrelease \n"); 00061 #endif 00062 } 00063 } 00064 00065 /* release right */ 00066 if( (pos < RBTN_MIN) || (pos >= DBL_MAX) ) 00067 { 00068 if( rtouchflg == true ) 00069 { 00070 mouse.release(MOUSE_RIGHT); 00071 rtouchflg = false; 00072 #if DEBUGCONSOLE 00073 pc.printf("rrelease \n"); 00074 #endif 00075 } 00076 } 00077 } 00078 00079 00080 int main(void) { 00081 00082 /* acc sensor init */ 00083 MMA8451Q acc(PTE25, PTE24, MMA8451_I2C_ADDRESS); 00084 00085 /* TSI init */ 00086 TSISensor tsi; 00087 00088 /* LED init */ 00089 PwmOut rled(LED_RED); 00090 PwmOut gled(LED_GREEN); 00091 PwmOut bled(LED_BLUE); 00092 00093 while (true) { 00094 00095 /* -1*accY correspond to mouse axe X; acc X correspond to mouse Y */ 00096 mouse.move( -1*int(acc.getAccY()*20), int(acc.getAccX()*20) ); 00097 00098 mbutton_detect( tsi.readPercentage()); 00099 00100 rled = 1.0 - abs(acc.getAccX()); 00101 gled = 1.0 - abs(acc.getAccY()); 00102 bled = 1.0 - abs(acc.getAccZ()); 00103 00104 wait(0.001); 00105 } 00106 } 00107
Generated on Tue Jul 19 2022 01:25:54 by
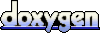