
Initial publish
Embed:
(wiki syntax)
Show/hide line numbers
menu.cpp
00001 #include "main.h" 00002 #include "menu.h" 00003 #include "constants.h" 00004 #include "game.h" 00005 #include "hud.h" 00006 #include "models.h" 00007 #include "gameobject.h" 00008 #include "stars.h" 00009 00010 Hud highScore; 00011 GameObject cursor; 00012 GameObject pressASprite; 00013 Stars menuStars; 00014 00015 Menu::Menu() { // NOTE to self: The constructor for declerering intial states of variables. 00016 // As soon as Menu menu; happens in main, the zero is addressed to the variable. 00017 current_option = 0; // Another form of declering intial values. 00018 // cheking whether the joystick was moved to point at other options in the menu. 00019 } 00020 00021 bool Menu::updateAndDraw() { 00022 checkJoystick(); 00023 drawPointer(); // Drawing pointer only ones. 00024 highScore.drawHighScore(); 00025 menuStars.starsSpawnDelay(); 00026 menuStars.updateAndDrawSmallStars(); 00027 menuStars.updateAndDrawMediumStars(); 00028 00029 lcd.printString("Start Game",1,2); 00030 lcd.printString("Tutorial",1,3); 00031 lcd.printString("Settings",1,4); 00032 DrawPressAIcon(); 00033 00034 bool option_picked = false; // Checking for the selecting button to be pressed and returning the boolean statement. 00035 if (gamepad.check_event(gamepad.A_PRESSED)){ 00036 gamepad.check_event(gamepad.A_PRESSED); 00037 option_picked = true; 00038 } 00039 00040 return option_picked; 00041 } 00042 00043 ScreenOption Menu::getCurrentScreenSelection() { // checking the current position of the pointer and main 00044 if (current_option == 0) { // if the "Game" was selected and button be pressed, intilise game. 00045 return ScreenOption_Game; // TASK for future: creat file with settings and tutorial. 00046 } 00047 if (current_option == 1) { 00048 return ScreenOption_Tutorial; 00049 } 00050 if (current_option == 2) { 00051 return ScreenOption_Settings; 00052 } 00053 return ScreenOption_Menu; 00054 } 00055 00056 void Menu::drawPointer(){ // Checking what option was selected and drawing the pointer 00057 // to indicate that postion. 00058 cursor.pos.x = 70; 00059 cursor.pos.y = 17; 00060 if (current_option == 0){ 00061 cursor.pos.y = 17; 00062 } 00063 else if (current_option == 1){ 00064 cursor.pos.y = 25; 00065 } 00066 else if (current_option == 2){ 00067 cursor.pos.y = 32; 00068 } 00069 drawSprite(cursor.pos, menu_cursor_sprite); 00070 } 00071 00072 void Menu::DrawPressAIcon(){ 00073 pressASprite.pos.x = screen_width - PressAWidth - 10; 00074 pressASprite.pos.y = screen_height - PressAHeight; 00075 drawSpriteOnTop(pressASprite.pos, Press_A_Icon_Sprite); 00076 } 00077 00078 void Menu::checkJoystick(){ 00079 if(y_dir.read() > joy_threshold_max_y){ 00080 current_option -= 1; 00081 wait_ms(time_delay); 00082 } 00083 else if (y_dir.read() < joy_threshold_min_y){ 00084 current_option += 1; 00085 wait_ms(time_delay); 00086 } 00087 if (current_option < 0) { 00088 current_option += total_options; 00089 } 00090 if (current_option >= total_options) { 00091 current_option -= total_options; 00092 } 00093 }
Generated on Wed Dec 20 2023 20:30:17 by
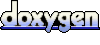