
GPS y GSM con envío de cadena de google maps.
Dependencies: GPSGSM TextLCD mbed
Fork of TAREA_4_GPS_GSM by
main.cpp
00001 #include "mbed.h" 00002 #include "DebouncedIn.h" 00003 #include "stdio.h" 00004 #include "string.h" 00005 #include "GPS.h" 00006 #include "TextLCD.h" 00007 00008 //Salidas digitales 00009 Timer t; 00010 Timer t2; 00011 DigitalOut LedVerde(LED2); 00012 DigitalOut LedRojo(LED1); 00013 DigitalOut LedAzul(LED3); 00014 00015 // Entrada análoga 00016 AnalogIn v(PTB0); 00017 float medi; 00018 00019 Serial GSM(PTE0,PTE1); 00020 Serial pc(USBTX,USBRX); 00021 GPS gps(PTE22, PTE23); 00022 TextLCD lcd(PTB10, PTB11, PTE2, PTE3, PTE4, PTE5); // rs, e, d4-d7 00023 DigitalOut led_alarma(PTA5); 00024 DigitalOut led_carro(PTA4); 00025 00026 char DE1[255]; 00027 char DS1[255]; 00028 char DE2[255]; 00029 char DS2[255]; 00030 char buffer[512]; 00031 char resp[6]; 00032 char tam[2]; 00033 char mensaje[100]; 00034 00035 //Variables enteras y caracteres 00036 int count; 00037 int i, K, LENOUT1, LENIN1, LENOUT2, LENIN2, C; 00038 int c=0; 00039 char r[]=""; 00040 char msg[256]; 00041 char char1; 00042 int ind; 00043 float med; 00044 char outmed[16], outmedn[16]; 00045 int ret = 1; 00046 char tel[15] = {'0', '3', '3', '4', '4', '7', '6', '2', '6', '1'}; 00047 bool noM2 = true, noM5 = true, noM3 = true, aviso = false, noOf = true; 00048 char mensajeM2[] = "Saliendo por la porteria del M2. Vehiculo apagado."; 00049 char mensajeM3[] = "Saliendo por la porteria del M3. Vehiculo apagado."; 00050 char mensajeM5[] = "Saliendo por la porteria del M5. Vehiculo apagado."; 00051 char mensajeOficina[255]; 00052 char buf[100]; 00053 char relle1[] = "0011000A91"; 00054 char relle2[] = "0000AA"; 00055 float latitud, longitud, frac_long, frac_lat; 00056 00057 // Usando el GPS se obtuvo las coordenadas de la porteria del bloque M2: 6.274062N, 75.592538W. 00058 float distanciaM2(float x, float y) { 00059 return sqrt(pow((x - 6.274062f), 2) + pow((y + 75.592538f), 2)); 00060 } 00061 00062 // Usando el GPS se obtuvo las coordenadas de la porteria del bloque M3: 6.274674N, 75.590680W. 00063 float distanciaM3(float x, float y) { 00064 return sqrt(pow((x - 6.274674f), 2) + pow((y + 75.590680f), 2)); 00065 } 00066 00067 // Usando el GPS se obtuvo las coordenadas de la porteria del bloque M5: 6.275458N, 75.591170W. 00068 float distanciaM5(float x, float y) { 00069 return sqrt(pow((x - 6.275458f), 2) + pow((y + 75.591170f), 2)); 00070 } 00071 00072 float distanciaOficina(float x, float y) { // https://www.google.com.co/maps/search/6.275206,-75.592836/ 00073 return sqrt(pow((x - 6.275206), 2) + pow((y + 75.592836f), 2)); 00074 } 00075 00076 // Funcion para enviar un mensaje de alerta: 00077 void alarma(char* msj) { 00078 strcpy(DE1,msj); 00079 LENIN1 = strlen(DE1); 00080 K = 0; 00081 C = 0; 00082 for (i = 0; i < LENIN1; i++){ 00083 DS1[i] = DE1[i + C] >> K | DE1[i + C + 1] << (7 - K); 00084 if(DS1[i] == 0x00) {LENOUT1 = i;} 00085 K++; 00086 if (K == 7) {K = 0; C++;} // se chequea que ya se acabaron los bits en un ciclo de conversion. 00087 } 00088 00089 // Concatenación del mensaje en formato PDU y envío del mismo. 00090 ind = 14 + LENOUT1 - 1; 00091 00092 GSM.printf("AT+CMGS=%d\r\n",ind); 00093 pc.printf("AT+CMGS=%d\r\n",ind); 00094 pc.printf(relle1); 00095 GSM.printf(relle1); 00096 00097 for (i=0 ;i<=9; i++) { 00098 pc.printf("%c",tel[i]); 00099 GSM.printf("%c",tel[i]); 00100 } 00101 00102 pc.printf(relle2); 00103 GSM.printf(relle2); 00104 pc.printf("%2X", LENIN1); 00105 GSM.printf("%2X", LENIN1); 00106 00107 for (i = 0; i < LENOUT1; i++){ 00108 pc.printf("%02X", DS1[i]&0x000000FF); 00109 GSM.printf("%02X", DS1[i]&0x000000FF); 00110 } 00111 wait(1); 00112 GSM.putc((char)0x1A); // Ctrl - Z. 00113 GSM.scanf("%s",buf); // Estado del mensaje (Envió o Error). 00114 pc.printf(">%s\n",buf); 00115 pc.printf("\n"); 00116 } 00117 00118 // Leer el buffer: 00119 int readBuffer(char *buffer,int count){ 00120 int i = 0; 00121 t.start(); 00122 while(true) { 00123 while (GSM.readable()) { 00124 char c = GSM.getc(); 00125 buffer[i++] = c; 00126 if(i > count + 1)break; 00127 } 00128 if(i > count)break; 00129 if(t.read() > 3) { 00130 t.stop(); 00131 t.reset(); 00132 break; 00133 } 00134 } 00135 wait(0.5); 00136 while(GSM.readable()) 00137 char c = GSM.getc(); 00138 return 0; 00139 } 00140 00141 void sendCmd(char *cmd){ 00142 GSM.puts(cmd); 00143 } 00144 00145 int waitForResp(char *resp, int timeout){ 00146 int len = strlen(resp); 00147 int sum = 0; 00148 t.start(); 00149 00150 while(true) { 00151 if(GSM.readable()) { 00152 char c = GSM.getc(); 00153 sum = (c == resp[sum]) ? sum + 1 : 0; 00154 if(sum == len)break; 00155 } 00156 if(t.read() > timeout) { 00157 t.stop(); 00158 t.reset(); 00159 return -1; 00160 } 00161 } 00162 t.stop(); 00163 t.reset(); 00164 while(GSM.readable()) { 00165 char c = GSM.getc(); 00166 } 00167 return 0; 00168 } 00169 00170 int sendCmdAndWaitForResp(char *cmd, char *resp, int timeout){ 00171 sendCmd(cmd); 00172 return waitForResp(resp,timeout); 00173 } 00174 00175 int init() { 00176 if (0 != sendCmdAndWaitForResp("AT\r\n", "OK", 3)) 00177 return -1; 00178 if (0 != sendCmdAndWaitForResp("AT+CNMI=1,1\r\n", "OK", 3)) 00179 return -1; 00180 if (0 != sendCmdAndWaitForResp("AT+CMGF=0\r\n", "OK", 3)) 00181 return -1; 00182 if (0 != sendCmdAndWaitForResp("AT+CBST=7,0,1\r\n", "OK", 3)) 00183 return -1; 00184 if (0 != sendCmdAndWaitForResp("ATE\r\n", "OK", 3)) 00185 return -1; 00186 LedVerde=0; 00187 return 0; 00188 } 00189 00190 int recibe_ok() { 00191 GSM.printf("AT+CMGS=27\n\r"); 00192 pc.printf("AT+CMGS=27\n\r"); 00193 wait_ms(100); 00194 GSM.printf("0011000A91%s0000AA10CDB27B1E569741F2F2382D4E93DF",tel); 00195 pc.printf("0011000A91%s0000AA10CDB27B1E569741F2F2382D4E93DF",tel); 00196 wait_ms(100); 00197 GSM.putc((char)0x1A); 00198 return 0; 00199 } 00200 00201 int main() { 00202 00203 t2.start(); 00204 GSM.baud(9600); 00205 GSM.format(8, Serial::None, 1); 00206 LedVerde = 1; 00207 LedRojo = 1; 00208 LedAzul = 1; 00209 led_carro = 1; 00210 led_alarma = 0; 00211 aviso = false; 00212 00213 inicio1: 00214 00215 // Configurando Modem... 00216 ret = init(); 00217 lcd.locate(0,0); 00218 lcd.printf("Configurando\nModem..."); 00219 wait(2); 00220 00221 if (ret == 0) { 00222 LedRojo = 1; 00223 LedVerde = 0; // Apagar LED Verde para confirmar la comunicación con el módem. 00224 pc.printf("Hecho!\n"); 00225 lcd.cls(); 00226 lcd.locate(0,0); 00227 lcd.printf("Hecho!"); 00228 wait(1); 00229 } 00230 else { 00231 wait(1); 00232 goto inicio1; 00233 } 00234 00235 lcd.cls(); 00236 lcd.locate(0,0); 00237 lcd.printf(" Alarma de \n seguridad. "); 00238 wait(5); 00239 00240 while(true) { 00241 00242 // A continuacion se verifica si esta entrando una llamada. 00243 // Si es el caso, se apaga el carro. 00244 00245 if (GSM.readable()) { 00246 00247 readBuffer(buffer, 110); 00248 00249 for(i=0; i < 5; i++) 00250 resp[i] = buffer[i]; 00251 00252 pc.printf(resp); 00253 00254 if (strcmp("\r\nRIN", resp) == 0) { 00255 lcd.cls(); 00256 led_carro = 0; 00257 lcd.locate(0,0); 00258 lcd.printf("Llamada!!!\nApagando carro.."); 00259 pc.printf("Llamada!!!\n\r"); 00260 wait(5); 00261 } 00262 } 00263 00264 char dir1[32]; 00265 char dir2[32]; 00266 char dirc[255]; 00267 00268 // Cada 3 segundos se lee el GPS para actualizar la posicion y enviar mensaje de alerta de ser necesario. 00269 if (int(t2.read()) % 3 == 0) { 00270 gps.sample(); 00271 latitud = gps.latitude; 00272 longitud = gps.longitude; 00273 00274 if (distanciaM5(latitud, longitud) <= 1000.00015f && !aviso) { 00275 aviso = true; 00276 led_alarma = 1; 00277 noM5 = false; 00278 sprintf(dir1, "%2.6f", latitud); 00279 sprintf(dir2, "%2.6f", longitud); 00280 strcpy(dirc, "https://www.google.com.co/maps/search/"); 00281 strcat(dirc, dir1); 00282 strcat(dirc, ","); 00283 strcat(dirc, dir2); 00284 alarma(dirc); 00285 lcd.cls(); 00286 lcd.locate(0,0); 00287 lcd.printf("M5: Enviando\nalerta..."); 00288 } 00289 else if (distanciaM5(latitud, longitud) > 0.00015f && aviso) 00290 noM5 = true; 00291 00292 if (distanciaM2(latitud, longitud) <= 0.00015f && !aviso) { 00293 aviso = true; 00294 led_alarma = 1; 00295 noM2 = false; 00296 sprintf(dir1, "%2.6f", latitud); 00297 sprintf(dir2, "%2.6f", longitud); 00298 strcpy(dirc, "https://www.google.com.co/maps/search/"); 00299 strcat(dirc, dir1); 00300 strcat(dirc, ","); 00301 strcat(dirc, dir2); 00302 alarma(dirc); 00303 lcd.cls(); 00304 lcd.locate(0,0); 00305 lcd.printf("M2: Enviando\nalerta..."); 00306 } 00307 else if (distanciaM2(latitud, longitud) > 0.00015f && aviso) 00308 noM2 = true; 00309 00310 if (distanciaM3(latitud, longitud) <= 0.00015f && !aviso) { 00311 aviso = true; 00312 led_alarma = 1; 00313 noM3 = false; 00314 sprintf(dir1, "%2.6f", latitud); 00315 sprintf(dir2, "%2.6f", longitud); 00316 strcpy(dirc, "https://www.google.com.co/maps/search/"); 00317 strcat(dirc, dir1); 00318 strcat(dirc, ","); 00319 strcat(dirc, dir2); 00320 alarma(dirc); 00321 lcd.cls(); 00322 lcd.locate(0,0); 00323 lcd.printf("M3: Enviando\nalerta..."); 00324 } 00325 else if (distanciaM3(latitud, longitud) > 0.00015f && aviso) 00326 noM3 = true; 00327 00328 if (noM2 && noM5 && noM3 && noOf && !aviso) { 00329 led_carro = 1; 00330 led_alarma = 0; 00331 } 00332 00333 // Si la distancia a la oficina es pequeña entonces envia la direccion de google Maps!!: 00334 if (distanciaOficina(latitud, longitud) <= 0.00015f && !aviso) { 00335 led_alarma = 1; 00336 aviso = true; 00337 noOf = false; 00338 sprintf(dir1, "%2.6f", latitud); 00339 sprintf(dir2, "%2.6f", longitud); 00340 strcpy(dirc, "https://www.google.com.co/maps/search/"); 00341 strcat(dirc, dir1); 00342 strcat(dirc, ","); 00343 strcat(dirc, dir2); 00344 pc.printf("%s\n", dirc); 00345 alarma(dirc); 00346 lcd.cls(); 00347 lcd.locate(0,0); 00348 lcd.printf("M3: Enviando\nalerta..."); 00349 wait(5); 00350 } 00351 else if (distanciaM3(latitud, longitud) > 0.00015f && aviso) 00352 noM3 = true; 00353 00354 lcd.cls(); 00355 lcd.locate(0,0); 00356 lcd.printf(" Lat: %02.6f\n Lon: %02.6f", latitud, longitud); 00357 00358 } 00359 00360 } 00361 }
Generated on Thu Jul 14 2022 20:01:33 by
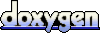