The IrcBot class can connect to a channel on an IRC network. Users on the same network can send messages to the bot that are parsed by message handlers. The included handlers read digital/analog inputs and write digital outputs or echo messages back to the command sender/channel. Users can write their own message handlers inheriting from the MessageHandler class to perform different actions.
IrcMessageHandlers.h
00001 #ifndef __mbed_irc_handlers_h__ 00002 #define __mbed_irc_handlers_h__ 00003 #include "IrcBot.h" 00004 00005 /// DigitalOutHandler lets users control a DigitalOut using "WRITE <name> ON/OFF" 00006 class DigitalOutHandler : private MessageHandler { 00007 public: 00008 /** Create a DigitalOutHandler 00009 * @param name Name of output used in command on IRC 00010 * @param pin Pin the output is connected to. 00011 * @param verbose Whether or not a reply is sent to IRC confirming the output state 00012 */ 00013 DigitalOutHandler(char * name, PinName pin, bool verbose); 00014 /// Set output pin on or off, reply "SET <name> ON/OFF" if verbose. 00015 IrcMessage handle(IrcMessage); 00016 private: 00017 DigitalOut pin; 00018 char name[32]; 00019 bool verbose; 00020 }; 00021 00022 /// DigitalInHandler lets users read a DigitalIn using "READ <name>" 00023 class DigitalInHandler : private MessageHandler { 00024 public: 00025 /** Create a DigitalInHandler 00026 * @param name Name of input used in command on IRC 00027 * @param pin Pin the input is connected to. 00028 */ 00029 DigitalInHandler(char * name, PinName pin); 00030 /// Reply "<name> IS ON/OFF" 00031 IrcMessage handle(IrcMessage); 00032 private: 00033 DigitalIn pin; 00034 char name[32]; 00035 }; 00036 00037 /// AnalogInHandler lets users read a DigitalIn using "READ <name>" 00038 class AnalogInHandler : private MessageHandler { 00039 public: 00040 /** Create an AnalogInHandler 00041 * @param name Name of input used in command on IRC 00042 * @param pin Pin the input is connected to. 00043 */ 00044 AnalogInHandler(char * name, PinName pin); 00045 /** Define a scaling factor for the measured value [0.0 - 1.0] 00046 * @param scale The scaling factor 00047 * @param unit The units used in the message to IRC 00048 */ 00049 void scale(float scale, char * unit); 00050 /// Measure input, scale and reply "<name> = <value> <units>" 00051 IrcMessage handle(IrcMessage); 00052 private: 00053 AnalogIn pin; 00054 char name[32]; 00055 char unit[32]; 00056 float scaleval; 00057 }; 00058 00059 /// A handler to echo back any messages of the form "ECHO <x>" 00060 class EchoHandler : private MessageHandler { 00061 public: 00062 EchoHandler(){}; 00063 /// Reply to "ECHO <x>" with "<x>" 00064 IrcMessage handle(IrcMessage msg); 00065 }; 00066 00067 #endif
Generated on Tue Jul 12 2022 20:41:27 by
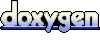