The IrcBot class can connect to a channel on an IRC network. Users on the same network can send messages to the bot that are parsed by message handlers. The included handlers read digital/analog inputs and write digital outputs or echo messages back to the command sender/channel. Users can write their own message handlers inheriting from the MessageHandler class to perform different actions.
IrcMessageHandlers.cpp
00001 #include "IrcMessageHandlers.h" 00002 00003 DigitalOutHandler::DigitalOutHandler(char * n, PinName p, bool v) : 00004 pin(p), verbose(v) 00005 { 00006 sprintf(name, "%s", n); 00007 pin = 0; 00008 } 00009 00010 IrcMessage DigitalOutHandler::handle(IrcMessage msg) { 00011 IrcMessage reply; 00012 if (verbose) { 00013 if (msg.to[0] == '#') { 00014 strcpy(reply.to, msg.to); 00015 } else { 00016 strcpy(reply.to, msg.from); 00017 } 00018 } 00019 char required[64]; 00020 sprintf(required, "WRITE %s", name); 00021 char * c = NULL; 00022 c = strstr(msg.msg, required); 00023 if (c != NULL) { 00024 c = strstr(msg.msg, "ON"); 00025 if (c != NULL) { 00026 pin = 1; 00027 if (verbose) { 00028 sprintf(reply.msg, "SET %s ON", name); 00029 } 00030 } 00031 c = strstr(msg.msg, "OFF"); 00032 if (c != NULL) { 00033 pin = 0; 00034 if (verbose) { 00035 sprintf(reply.msg, "SET %s OFF", name); 00036 } 00037 } 00038 } 00039 return reply; 00040 } 00041 00042 DigitalInHandler::DigitalInHandler(char * n, PinName p) : 00043 pin(p) 00044 { 00045 sprintf(name, "%s", n); 00046 } 00047 00048 IrcMessage DigitalInHandler::handle(IrcMessage msg) { 00049 IrcMessage reply; 00050 if (msg.to[0] == '#') { 00051 strcpy(reply.to, msg.to); 00052 } else { 00053 strcpy(reply.to, msg.from); 00054 } 00055 char required[64]; 00056 sprintf(required, "READ %s", name); 00057 char * c = NULL; 00058 c = strstr(msg.msg, required); 00059 if (c != NULL) { 00060 if (pin) { 00061 sprintf(reply.msg, "%s is ON", name); 00062 } else { 00063 sprintf(reply.msg, "%s is OFF", name); 00064 } 00065 } 00066 return reply; 00067 } 00068 00069 AnalogInHandler::AnalogInHandler(char * n, PinName p) : 00070 pin(p), scaleval(1.0) 00071 { 00072 sprintf(name, "%s", n); 00073 sprintf(unit, ""); 00074 } 00075 00076 void AnalogInHandler::scale(float s, char * u) { 00077 scaleval = s; 00078 sprintf(unit, "%s", u); 00079 } 00080 00081 IrcMessage AnalogInHandler::handle(IrcMessage msg) { 00082 IrcMessage reply; 00083 if (msg.to[0] == '#') { 00084 strcpy(reply.to, msg.to); 00085 } else { 00086 strcpy(reply.to, msg.from); 00087 } 00088 char required[64]; 00089 sprintf(required, "READ %s", name); 00090 char * c = NULL; 00091 c = strstr(msg.msg, required); 00092 if (c != NULL) { 00093 float v = pin * scaleval; 00094 sprintf(reply.msg, "%s = %f %s", name, v, unit); 00095 } 00096 return reply; 00097 } 00098 00099 IrcMessage EchoHandler::handle(IrcMessage msg) { 00100 IrcMessage reply; 00101 char * c = NULL; 00102 c = strstr(msg.msg, "ECHO "); 00103 if (c != NULL) { 00104 strcpy(reply.from, msg.to); 00105 if (msg.to[0] == '#') { 00106 strcpy(reply.to, msg.to); 00107 } else { 00108 strcpy(reply.to, msg.from); 00109 } 00110 strcpy(reply.msg, c + 5); 00111 } 00112 return reply; 00113 }
Generated on Tue Jul 12 2022 20:41:27 by
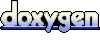