The IrcBot class can connect to a channel on an IRC network. Users on the same network can send messages to the bot that are parsed by message handlers. The included handlers read digital/analog inputs and write digital outputs or echo messages back to the command sender/channel. Users can write their own message handlers inheriting from the MessageHandler class to perform different actions.
IrcBot.h
00001 #ifndef __mbed_irc_h__ 00002 #define __mbed_irc_h__ 00003 00004 #include "mbed.h" 00005 #include "EthernetInterface.h" 00006 #include <vector> 00007 00008 /** 00009 * IrcBot responds to commands on IRC using message users' handlers 00010 */ 00011 class IrcMessage { 00012 public: 00013 /** Create empty IrcMessage */ 00014 IrcMessage(); 00015 00016 /** Create an IrcMessage 00017 * @param from The user sending the message. 00018 * @param to The user/channel receiving the message. 00019 * @param message The message. 00020 */ 00021 IrcMessage(char * from, char * to, char * message); 00022 char from[32], to[32], msg[256]; 00023 }; 00024 00025 /** 00026 * Base MessageHandler class. 00027 * Users should write classes inheriting from MessageHandler to parse and 00028 * respond to incoming IRC messages. 00029 */ 00030 class MessageHandler { 00031 public: 00032 MessageHandler() {}; 00033 virtual IrcMessage handle(IrcMessage msg) {return IrcMessage();} 00034 }; 00035 00036 /** 00037 * IrcBot connects to an IRC network and joins a channel. Users can add message 00038 * handlers which parse incoming private messages and respond to them. 00039 */ 00040 class IrcBot { 00041 public: 00042 /** Create an IrcBot 00043 * 00044 * @param nickname Bot's nickname 00045 * @param network IRC network to join 00046 * @param port Port to connect to network on 00047 * @param channel Channel to connect to 00048 */ 00049 IrcBot(char * nickname, char * network, int port, char * channel); 00050 /** Connect to the network. 00051 * 00052 * Users should have already created a network interface 00053 * (Ethernet/Wifi/3G/whatever) to carry the connection. 00054 */ 00055 void connect(); 00056 /// Disconnect from the network. 00057 void disconnect(); 00058 /// Add a handler for incoming messages. 00059 void add(MessageHandler *); 00060 00061 /** Read data from internet connection, parse input and handle any 00062 * incoming private messages 00063 */ 00064 bool read(); 00065 private: 00066 void handle(IrcMessage); 00067 void parse(); 00068 void send(char *); 00069 Serial pc; 00070 TCPSocketConnection sock; 00071 char network[64]; 00072 char channel[64]; 00073 int port; 00074 char nickname[64]; 00075 char password[64]; 00076 bool ident, connected, setup, joined; 00077 char readbuffer[512]; 00078 char parsebuffer[512]; 00079 int parseindex; 00080 vector<MessageHandler *> handlers; 00081 }; 00082 00083 #endif
Generated on Tue Jul 12 2022 20:41:27 by
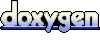