The IrcBot class can connect to a channel on an IRC network. Users on the same network can send messages to the bot that are parsed by message handlers. The included handlers read digital/analog inputs and write digital outputs or echo messages back to the command sender/channel. Users can write their own message handlers inheriting from the MessageHandler class to perform different actions.
IrcBot.cpp
00001 #include "IrcBot.h" 00002 00003 IrcMessage::IrcMessage(char * f, char * t, char * m) { 00004 sprintf(from, "%s", f); 00005 sprintf(to, "%s", t); 00006 sprintf(msg, "%s", m); 00007 } 00008 00009 IrcMessage::IrcMessage() { 00010 from[0] = '\0'; 00011 to[0] = '\0'; 00012 msg[0] = '\0'; 00013 } 00014 00015 IrcBot::IrcBot(char * nick, char * net, int p, char * chan) : 00016 pc(USBTX, USBRX), sock(), port(p), ident(false), connected(false), 00017 setup(false), joined(false), parseindex(0) 00018 { 00019 sprintf(nickname, "%s", nick); 00020 sprintf(password, "%s", ""); 00021 sprintf(network, "%s", net); 00022 sprintf(channel, "%s", chan); 00023 pc.printf("Created IrcBot obj for %s.\n", nickname); 00024 } 00025 00026 void IrcBot::connect() { 00027 pc.printf("Connecting to %s:%d...\n", network, port); 00028 sock.connect(network, port); 00029 pc.printf("Done.\n"); 00030 connected = true; 00031 } 00032 00033 void IrcBot::disconnect() { 00034 if (connected) { 00035 pc.printf("Disconnecting.\n"); 00036 sock.close(); 00037 connected = false; 00038 } 00039 } 00040 00041 void IrcBot::add(MessageHandler * handler) { 00042 handlers.push_back(handler); 00043 } 00044 00045 bool IrcBot::read() { 00046 if (!connected) return 0; 00047 int ret = sock.receive(readbuffer, sizeof(readbuffer) - 1); 00048 bool parsed = false; 00049 if (ret >= 0) { 00050 readbuffer[ret] = '\0'; 00051 pc.printf("Received %d chars: ---%s--\n", ret, readbuffer); 00052 for (int i = 0; i < ret; i++) { 00053 parsebuffer[parseindex] = readbuffer[i]; 00054 parseindex++; 00055 if (readbuffer[i] == '\n') { 00056 parsebuffer[parseindex] = '\0'; 00057 parse(); 00058 parsed = true; 00059 parseindex = 0; 00060 } 00061 } 00062 } 00063 return parsed; 00064 } 00065 00066 void IrcBot::send(char * cmd) { 00067 if (!connected) return; 00068 int i = 0; 00069 bool ok = true; 00070 while (cmd[i] != '\0') { 00071 i++; 00072 if (i > 512) { 00073 ok = false; 00074 break; 00075 } 00076 } 00077 if (!ok) return; 00078 pc.printf("Sending: ---%s---\n", cmd); 00079 sock.send_all(cmd, i); 00080 } 00081 00082 void IrcBot::handle(IrcMessage msg) { 00083 pc.printf("Handling message from %s, to %s: %s\n", msg.from, msg.to, msg.msg); 00084 for (int i = 0; i < handlers.size(); i++) { 00085 IrcMessage out = handlers.at(i)->handle(msg); 00086 if (out.to[0] != '\0') { 00087 sprintf(out.from, "%s", nickname); 00088 pc.printf("Need to send: ---%s--- from %s to %s.\n", out.msg, out.from, out.to); 00089 char cmd[512]; 00090 sprintf(cmd, "PRIVMSG %s :%s\r\n", out.to, out.msg); 00091 send(cmd); 00092 } 00093 } 00094 } 00095 00096 void IrcBot::parse() { 00097 pc.printf("Parsing: --%s--\n", parsebuffer); 00098 if (setup && (!joined)) { 00099 char cmd[256]; 00100 sprintf(cmd, ":source JOIN :%s\r\n", channel); 00101 send(cmd); 00102 joined = true; 00103 return; 00104 } 00105 if (!setup) { 00106 char cmd[256]; 00107 sprintf(cmd, "NICK %s\r\nUSER %s 0 * :Lester\r\n", nickname, nickname); 00108 send(cmd); 00109 setup = true; 00110 return; 00111 } 00112 char * c = NULL; 00113 c = strstr(parsebuffer, "PING"); 00114 if (c != NULL) { 00115 char cmd[] = "PONG\r\n"; 00116 send(cmd); 00117 } 00118 c = strstr(parsebuffer, "PRIVMSG"); 00119 if (c != NULL) { 00120 char f[32]; 00121 char t[32]; 00122 char m[256]; 00123 int i; 00124 for (i = 1; i < 32; i++) { 00125 if (parsebuffer[i] == '!') { 00126 f[i - 1] = '\0'; 00127 break; 00128 } 00129 f[i - 1] = parsebuffer[i]; 00130 } 00131 int nspace = 0; 00132 bool inmsg = false; 00133 int toindex = 0, msgindex = 0; 00134 for (; i < sizeof(parsebuffer); i++) { 00135 if (parsebuffer[i] == '\r') break; 00136 if (parsebuffer[i] == '\n') break; 00137 if (parsebuffer[i] == '\0') break; 00138 if (parsebuffer[i] == ' ') { 00139 nspace++; 00140 } 00141 if ((nspace == 2) && (parsebuffer[i] != ' ')) { 00142 t[toindex] = parsebuffer[i]; 00143 toindex++; 00144 } 00145 if (inmsg) { 00146 m[msgindex] = parsebuffer[i]; 00147 msgindex++; 00148 } 00149 if ((parsebuffer[i] == ':') && (!inmsg)) { 00150 inmsg = true; 00151 } 00152 } 00153 t[toindex] = '\0'; 00154 m[msgindex] = '\0'; 00155 IrcMessage msg(f, t, m); 00156 handle(msg); 00157 } 00158 }
Generated on Tue Jul 12 2022 20:41:27 by
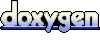