
Trigger by rising edge
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 * ----------------------------------------- 00003 * Trigger_LabSat_variant_2 1.0 (2018-04-05) 00004 * ----------------------------------------- 00005 * Works well with NUCLEO-F031K6 and NUCLEO-F411RE. 00006 * 00007 * In (D2): ___-______-___ 00008 * 00009 * Out (D3): ___--------___ 00010 * 00011 * negOut (D4): ---________--- 00012 * 00013 * LED (LED1): ___--------___ 00014 * 00015 * Copyright (c) 2018, Martin Wolker (neolker@gmail.com) 00016 * All rights reserved. 00017 * 00018 * Redistribution and use in source and binary forms, with or without 00019 * modification, are permitted provided that the following conditions are met: 00020 * - Redistributions of source code must retain the above copyright 00021 * notice, this list of conditions and the following disclaimer. 00022 * - Redistributions in binary form must reproduce the above copyright 00023 * notice, this list of conditions and the following disclaimer in the 00024 * documentation and/or other materials provided with the distribution. 00025 * - Neither the name of Martin Wolker nor the 00026 * names of its contributors may be used to endorse or promote products 00027 * derived from this software without specific prior written permission. 00028 * 00029 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 00030 * ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00031 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00032 * DISCLAIMED. IN NO EVENT SHALL MARTIN WOLKER BE LIABLE FOR ANY 00033 * DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00034 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00035 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00036 * ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00037 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00038 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00039 */ 00040 00041 #include "mbed.h" 00042 #define TRIGGER_IGNORE_LENGTH_MS 500 00043 00044 InterruptIn trigger_in(D2); 00045 DigitalOut trigger_out(D3); 00046 DigitalOut trigger_out_negative(D4); 00047 DigitalOut trigger_led(LED1); 00048 00049 void trigger(void) 00050 { 00051 trigger_out =! trigger_out; 00052 trigger_out_negative =! trigger_out_negative; 00053 trigger_led =! trigger_led; 00054 } 00055 00056 void interrupt(void) 00057 { 00058 trigger(); 00059 wait_ms(TRIGGER_IGNORE_LENGTH_MS); 00060 } 00061 00062 int main(void) 00063 { 00064 //Trigger interrupt by rising edge 00065 trigger_in.rise(&interrupt); 00066 00067 //Default state 00068 trigger_out = 0; 00069 trigger_out_negative = 1; 00070 trigger_led = 0; 00071 00072 //Endless loop 00073 while(1) { } 00074 }
Generated on Thu Jul 21 2022 09:04:09 by
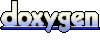