
LVTTL HW Trigger with control via virtual Serial over USB. Works well with NUCLEO boards.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 * ---------------------------- 00003 * TriggerNiNo 1.0 (2016-09-26) 00004 * ---------------------------- 00005 * LVTTL HW Trigger with control via virtual Serial over USB. 00006 * Works well with NUCLEO-F031K6 and NUCLEO-F411RE. 00007 * 00008 * Copyright (c) 2016, Martin Wolker (neolker@gmail.com) 00009 * All rights reserved. 00010 * 00011 * Redistribution and use in source and binary forms, with or without 00012 * modification, are permitted provided that the following conditions are met: 00013 * - Redistributions of source code must retain the above copyright 00014 * notice, this list of conditions and the following disclaimer. 00015 * - Redistributions in binary form must reproduce the above copyright 00016 * notice, this list of conditions and the following disclaimer in the 00017 * documentation and/or other materials provided with the distribution. 00018 * - Neither the name of Martin Wolker nor the 00019 * names of its contributors may be used to endorse or promote products 00020 * derived from this software without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 00023 * ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00024 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00025 * DISCLAIMED. IN NO EVENT SHALL MARTIN WOLKER BE LIABLE FOR ANY 00026 * DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00027 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00028 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00029 * ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00030 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00031 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00032 */ 00033 00034 #include "mbed.h" 00035 #define TRIGGER_PULSE_LENGTH_MS 500 00036 00037 Serial pc(USBTX, USBRX); 00038 DigitalOut trigger_output_positive(D2); 00039 DigitalOut trigger_output_negative(D3); 00040 DigitalOut trigger_indication(LED1); 00041 00042 void trigger(void) 00043 { 00044 trigger_output_positive =! trigger_output_positive; 00045 trigger_output_negative =! trigger_output_negative; 00046 trigger_indication =! trigger_indication; 00047 } 00048 00049 void interrupt(void) 00050 { 00051 pc.getc(); 00052 trigger(); 00053 wait_ms(TRIGGER_PULSE_LENGTH_MS); 00054 trigger(); 00055 } 00056 00057 int main(void) 00058 { 00059 pc.attach(&interrupt); 00060 trigger_output_positive = 0; 00061 trigger_output_negative = 1; 00062 trigger_indication = 0; 00063 while(1) {} 00064 }
Generated on Tue Jul 12 2022 18:07:35 by
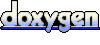