Humidity and Temperature Sensor - Sensirion SHT1x driver
Dependents: ProgrammePrincipal Wetterstation project1 4180_Project ... more
i2c.hpp
00001 /** 00002 * Copyright (c) 2010 Roy van Dam <roy@negative-black.org> 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without 00006 * modification, are permitted provided that the following conditions 00007 * are met: 00008 * 1. Redistributions of source code must retain the above copyright 00009 * notice, this list of conditions and the following disclaimer. 00010 * 2. Redistributions in binary form must reproduce the above copyright 00011 * notice, this list of conditions and the following disclaimer in the 00012 * documentation and/or other materials provided with the distribution. 00013 * 00014 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR AND CONTRIBUTORS ``AS IS'' AND 00015 * ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00016 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE 00017 * ARE DISCLAIMED. IN NO EVENT SHALL THE AUTHOR OR CONTRIBUTORS BE LIABLE 00018 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00019 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS 00020 * OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) 00021 * HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT 00022 * LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY 00023 * OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF 00024 * SUCH DAMAGE. 00025 */ 00026 00027 #ifndef _I2C_HPP_ 00028 #define _I2C_HPP_ 00029 00030 #include "mbed.h" 00031 00032 namespace SHTx { 00033 /** 00034 * Class: I2C 00035 * Humidity and Temperature Sensor - SHT15 00036 * I2C Bit-bang master driver. 00037 */ 00038 class I2C { 00039 private: 00040 DigitalInOut scl_pin; 00041 DigitalInOut sda_pin; 00042 00043 uint32_t frequency; 00044 00045 public: 00046 /** 00047 * Constructor: SHTx::I2C 00048 * Create an I2C Master interface, connected to the specified pins. 00049 * Bit-bang I2C driver to get around the lousy I2C implementation in the 00050 * SHTx interface... 00051 * 00052 * Variables: 00053 * sda - I2C data line pin 00054 * scl - I2C clock line pin 00055 */ 00056 I2C(PinName sda, PinName scl); 00057 00058 /** 00059 * Function: setFrequency 00060 * Set the frequency of the SHTx::I2C interface 00061 * 00062 * Variables: 00063 * hz - The bus frequency in hertz 00064 */ 00065 void setFrequency(uint32_t hz); 00066 00067 /** 00068 * Function: start 00069 * Issue start condition on the SHTx::I2C bus 00070 */ 00071 void start(void); 00072 00073 /** 00074 * Function: stop 00075 * Issue stop condition on the SHTx::I2C bus 00076 */ 00077 void stop(void); 00078 00079 /** 00080 * Function: wait 00081 * Wait for SHT15 to complete measurement. 00082 * Max timeout 500ms. 00083 * 00084 * Variables: 00085 * returns - true if an ACK was received, false otherwise 00086 */ 00087 bool wait(void); 00088 00089 /** 00090 * Function: reset 00091 * If communication with the device is lost 00092 * the command will reset the serial interface 00093 */ 00094 void reset(void); 00095 00096 /** 00097 * Function: write 00098 * Write single byte out on the SHTx::I2C bus 00099 * 00100 * Variables: 00101 * data - data to write out on bus 00102 * returns - true if an ACK was received, false otherwise 00103 */ 00104 bool write(uint8_t data); 00105 00106 /** 00107 * Function: write 00108 * Read single byte form the I2C bus 00109 * 00110 * Variables: 00111 * ack - indicates if the byte is to be acknowledged 00112 * returns - the read byte 00113 */ 00114 uint8_t read(bool ack); 00115 00116 private: 00117 /** 00118 * Function: output 00119 * Configures sda pin as output 00120 */ 00121 void output(void); 00122 00123 /** 00124 * Function: input 00125 * Configures sda pin as input 00126 */ 00127 void input(void); 00128 00129 /** 00130 * Function: sda 00131 * Drive sda pin. 00132 * 00133 * Variables: 00134 * value - drive pin high or low 00135 */ 00136 void sda(bool value); 00137 00138 /** 00139 * Function: scl 00140 * Drive scl pin. 00141 * 00142 * Variables: 00143 * value - drive pin high or low 00144 */ 00145 void scl(bool value); 00146 00147 /** 00148 * Function: shift_out 00149 * Write single bit out on the SHTx::I2C bus 00150 * 00151 * Variables: 00152 * bit - value of the bit to be written. 00153 */ 00154 void shift_out(bool bit); 00155 00156 /** 00157 * Function: shift_in 00158 * Read single bit from the SHTx::I2C bus 00159 * 00160 * Variables: 00161 * return - value of the bit read. 00162 */ 00163 bool shift_in(void); 00164 }; 00165 } 00166 00167 /* !_I2C_HPP_ */ 00168 #endif
Generated on Wed Jul 13 2022 17:19:11 by
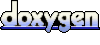