Bringing MBed serial ports back like it's 1999... or at least 2019.
Dependents: CC1200-MorseEncoder AccurateWaiter-Test
SerialStream.h
00001 #ifndef SERIALSTREAM_H 00002 #define SERIALSTREAM_H 00003 00004 #include <platform/Stream.h> 00005 00006 /** 00007 * SerialStream 00008 * Bringing MBed serial ports back like it's 1999... or at least 2019. 00009 * 00010 * This class adapts an MBed 6.0 serial port class into a Stream instance. 00011 * This lets you do two useful things with it: 00012 * - Call printf() and scanf() on it 00013 * - Pass it to code that expects a Stream to print things on. 00014 * 00015 */ 00016 template<class SerialClass> 00017 class SerialStream : public Stream 00018 { 00019 SerialClass & serialClass; 00020 00021 public: 00022 00023 /** 00024 * Create a SerialStream from a serial port. 00025 * @param _serialClass BufferedSerial or UnbufferedSerial instance 00026 * @param name The name of the stream associated with this serial port (optional) 00027 */ 00028 SerialStream(SerialClass & _serialClass, const char *name = nullptr): 00029 Stream(name), 00030 serialClass(_serialClass) 00031 { 00032 } 00033 00034 // override Stream::read() and write() to call serial class directly. 00035 // This avoids the overhead of feeding in individual characters. 00036 virtual ssize_t write(const void *buffer, size_t length) 00037 { 00038 return serialClass.write(buffer, length); 00039 } 00040 00041 virtual ssize_t read(void *buffer, size_t length) 00042 { 00043 return serialClass.read(buffer, length); 00044 } 00045 00046 protected: 00047 // Dummy implementations -- these will never be called because we override write() and read() instead. 00048 // but we have to override them since they're pure virtual. 00049 virtual int _putc(int c) { return 0; } 00050 virtual int _getc() { return 0; } 00051 }; 00052 00053 #endif //SERIALSTREAM_H
Generated on Thu Jul 14 2022 12:27:09 by
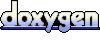