
Flappy Bird game on mbed with a micro LCD screen, class D amp, speaker, SD card reader/writer, 5-button navigation switch, and potentiometer speed control
Dependencies: 4DGL-uLCD-SE PinDetect SDFileSystem mbed-rtos mbed
soundBuilder.h
00001 #include "Speaker.h" 00002 00003 #ifndef SOUNDBUILDER_H 00004 #define SOUNDBUILDER_H 00005 00006 // class that represents a note played by a speaker 00007 class Note 00008 { 00009 public: 00010 // Constructor 00011 Note(); 00012 Note(float, float, float); 00013 // Set Functions 00014 void setFreq(float); // set the note's frequency 00015 void setLength(float); // set the note's length 00016 void setVolume(float); // set the note's volume 00017 // Get Functions 00018 float getFreq(); // return the note's frequency 00019 float getLength(); // return the note's length 00020 float getVolume(); // return the note's volume 00021 private: 00022 float freq; // the note's frequency 00023 float length; // the note's length 00024 float volume; // the note's volume 00025 }; 00026 00027 class SoundBuilder 00028 { 00029 public: 00030 // Set Song 00031 SoundBuilder (float [], float [], float [], int, Speaker *); 00032 // Set sound, where int is the note number starting from 0 00033 void setNote(float, float, float, int); 00034 // Play Song from given start and stop positions 00035 void playNotes(int, int); 00036 // Play Song 00037 void playSong(); 00038 // Clear Songs 00039 void clearSong(); 00040 00041 private: 00042 Note song[20]; // a song of up to 20 notes 00043 Speaker *speaker; // speaker that plays the song 00044 00045 }; 00046 00047 #endif // SOUNDBUILDER_H
Generated on Tue Jul 19 2022 06:40:37 by
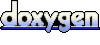