Fixed version not to issue warning messages. Original: Revision 26:8f15aa3b052b by Dieter Graef. https://developer.mbed.org/users/DieterGraef/code/SDFileSystem/
Dependents: F746_AudioPlayerSD F746_SD_WavPlayer F746_SD_GraphicEqualizer_ren0620 F746_SD_TextFile_RW ... more
SD_Helper.c
00001 #include "SD_Helper.h" 00002 #include "stm32746g_discovery_sd.h" 00003 00004 #define _CCSIDR_LSSHIFT(x) (((x) & SCB_CCSIDR_LINESIZE_Msk) >> SCB_CCSIDR_LINESIZE_Pos) 00005 00006 static SD_HandleTypeDef uSdHandle; 00007 static int RX_Busy; 00008 static int TX_Busy; 00009 static int SD_Busy; 00010 00011 00012 00013 /* cache flush */ 00014 void CPU_CACHE_Flush(uint32_t * buffer, uint32_t length) 00015 { 00016 // SCB_InvalidateDCache_by_Addr(buffer,length); 00017 uint32_t ccsidr; 00018 uint32_t smask; 00019 uint32_t sshift; 00020 uint32_t ways; 00021 uint32_t wshift; 00022 uint32_t ssize; 00023 uint32_t sets; 00024 uint32_t sw; 00025 uint32_t start; 00026 uint32_t ende; 00027 00028 start=(uint32_t)buffer; 00029 ende=start+length; 00030 /* Get the characteristics of the D-Cache */ 00031 00032 ccsidr = SCB->CCSIDR; 00033 smask = CCSIDR_SETS(ccsidr); /* (Number of sets) - 1 */ 00034 sshift = _CCSIDR_LSSHIFT(ccsidr) + 4; /* log2(cache-line-size-in-bytes) */ 00035 ways = CCSIDR_WAYS(ccsidr); /* (Number of ways) - 1 */ 00036 00037 /* Calculate the bit offset for the way field in the DCCISW register by 00038 * counting the number of leading zeroes. For example: 00039 * 00040 * Number of Value of ways Field 00041 * Ways 'ways' Offset 00042 * 2 1 31 00043 * 4 3 30 00044 * 8 7 29 00045 * ... 00046 */ 00047 00048 wshift = __CLZ(ways) & 0x1f; 00049 00050 /* Clean and invalidate the D-Cache over the range of addresses */ 00051 00052 ssize = (1 << sshift); 00053 start &= ~(ssize - 1); 00054 __DSB(); 00055 00056 do 00057 { 00058 int32_t tmpways = ways; 00059 00060 /* Isolate the cache line associated with this address. For example 00061 * if the cache line size is 32 bytes and the cache size is 16KB, then 00062 * 00063 * sshift = 5 : Offset to the beginning of the set field 00064 * smask = 0x007f : Mask of the set field 00065 */ 00066 00067 sets = ((uint32_t)start >> sshift) & smask; 00068 00069 /* Clean and invalidate each way for this cacheline */ 00070 00071 do 00072 { 00073 sw = ((tmpways << wshift) | (sets << sshift)); 00074 SCB->DCCISW=sw; 00075 00076 } 00077 while (tmpways--); 00078 00079 /* Increment the address by the size of one cache line. */ 00080 00081 start += ssize; 00082 } 00083 while (start < ende); 00084 00085 __DSB(); 00086 __ISB(); 00087 } 00088 00089 void BSP_SD_CommandTransaction(char cmd, unsigned int arg) 00090 { 00091 SDMMC_CmdInitTypeDef sdmmc_cmdinitstructure; 00092 uSdHandle.Instance = SDMMC1; 00093 sdmmc_cmdinitstructure.Argument = (uint32_t)arg; 00094 sdmmc_cmdinitstructure.CmdIndex = cmd; 00095 sdmmc_cmdinitstructure.Response = SDMMC_RESPONSE_SHORT; 00096 sdmmc_cmdinitstructure.WaitForInterrupt = SDMMC_WAIT_NO; 00097 sdmmc_cmdinitstructure.CPSM = SDMMC_CPSM_ENABLE; 00098 SDMMC_SendCommand(uSdHandle.Instance, &sdmmc_cmdinitstructure); 00099 } 00100 00101 void BSP_SD_Set_Busy(void) 00102 { 00103 SD_Busy=1; 00104 } 00105 void BSP_SD_Clear_Busy(void) 00106 { 00107 SD_Busy=0; 00108 } 00109 int BSP_SD_Get_Busy(void) 00110 { 00111 return SD_Busy; 00112 } 00113 00114 void BSP_SD_Set_RX_Busy(void) 00115 { 00116 RX_Busy=1; 00117 } 00118 void BSP_SD_Clear_RX_Busy(void) 00119 { 00120 RX_Busy=0; 00121 } 00122 int BSP_SD_Get_RX_Busy(void) 00123 { 00124 return RX_Busy; 00125 } 00126 00127 void BSP_SD_Set_TX_Busy(void) 00128 { 00129 TX_Busy=1; 00130 } 00131 void BSP_SD_Clear_TX_Busy(void) 00132 { 00133 TX_Busy=0; 00134 } 00135 int BSP_SD_Get_TX_Busy(void) 00136 { 00137 return TX_Busy; 00138 }
Generated on Tue Jul 12 2022 23:17:10 by
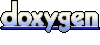