FrqRespDrawer class to draw frequency response for digital filter. ディジタルフィルタの周波数特性を,周波数軸をログスケールで描画するための FrqRespDrawer クラス. このライブラリを登録した際のプログラム:「F746_FrequencyResponseDrawer_Demo」
Dependents: F746_SD_WavPlayer F746_SD_GraphicEqualizer_ren0620 F746_FrequencyResponseDrawer_Demo F746_SD_VarableFilter ... more
FrquencyResponseDrawer.hpp
00001 //------------------------------------------------------------------------ 00002 // ディジタルフィルタの周波数特性を,周波数軸が対数スケールで描画するクラス(ヘッダ) 00003 // FrqRespDrawer class (header) 00004 // 00005 // 2017/03/16, Copyright (c) 2017 MIKAMI, Naoki 00006 //------------------------------------------------------------------------ 00007 00008 #ifndef F746_FRQ_RESP_DRAWER_HPP 00009 #define F746_FRQ_RESP_DRAWER_HPP 00010 00011 #include "NumericLabel.hpp" 00012 #include "FrequancyResponseBase.hpp" 00013 00014 namespace Mikami 00015 { 00016 class FrqRespDrawer 00017 { 00018 public: 00019 // 横軸の目盛値を描画する際に使う構造体 00020 struct AxisX_Char { uint16_t frq; string str; }; 00021 00022 // Constructor 00023 FrqRespDrawer(uint16_t orgX, float min, float max, 00024 uint16_t dec, // 周波数の 10 倍に対応する長さ(ピクセル数) 00025 uint16_t orgY, 00026 float minDb, // 表示する dB 値の最小値 00027 float maxDb, // 表示する dB 値の最大値 00028 float db1Pixel, // 1 dB に対応するピクセル数 00029 float ySpace, // 目盛線の縦方向の間隔(dB 単位) 00030 float fs, 00031 uint32_t lineColor = 0xFF00B0FF, 00032 uint32_t axisColor = LCD_COLOR_LIGHTGRAY, 00033 uint32_t backColor = GuiBase::ENUM_BACK) 00034 : lcd_(GuiBase::GetLcd()), 00035 ORGX_(orgX), MIN_(min), MAX_(max), DEC_(dec), 00036 ORGY_(orgY), MIN_DB_(minDb), MAX_DB_(maxDb), 00037 DB1_(db1Pixel), Y_SPACE_(db1Pixel*ySpace), FS_(fs), 00038 LINE_COLOR_(lineColor), 00039 AXIS_COLOR_(axisColor), 00040 BACK_COLOR_(backColor) {} 00041 00042 // 周波数に対応する x 座標値の取得 00043 int X(float frq) 00044 { return Round(ORGX_ + DEC_*log10f(frq/MIN_)); } 00045 00046 // x 座標値を周波数に変換 00047 float PosToFrq(uint16_t x) 00048 { return MIN_*powf(10.0f, (x - ORGX_)/(float)DEC_); } 00049 00050 // 目盛線の描画 00051 void DrawAxis(); 00052 00053 // 横軸の目盛値の表示 00054 void DrawNumericX(AxisX_Char xChar[], int nDisp, int offsetY, 00055 string str, sFONT &fonts = Font12, 00056 uint32_t textColor = LCD_COLOR_WHITE); 00057 00058 // 縦軸の目盛値の表示 00059 void DrawNumericY(int offsetX, int offsetY, uint16_t d_dB, 00060 const char fmt[], sFONT &fonts = Font12, 00061 uint32_t textColor = LCD_COLOR_WHITE, 00062 string str = "[dB]"); 00063 00064 // 周波数特性のグラフの描画 00065 void DrawGraph(FrequencyResponse &frqResp, uint32_t color); 00066 void DrawGraph(FrequencyResponse &frqResp) 00067 { DrawGraph(frqResp, LINE_COLOR_);} 00068 00069 // 消去 00070 // upDb : 消去する範囲の上限(dB 単位) 00071 void Erase(float upDb = 0); 00072 00073 private: 00074 LCD_DISCO_F746NG &lcd_; 00075 const uint16_t ORGX_; // 横軸の目盛の最小値に対応する位置 00076 const float MIN_; // 横軸の目盛の最小値 00077 const float MAX_; // 横軸の目盛の最大値 00078 const uint16_t DEC_; // 周波数の 10 倍に対応する長さ (pixels) 00079 const uint16_t ORGY_; // 縦軸の目盛の最小値に対応する位置 00080 const float MIN_DB_; // 縦軸の目盛の最小値 [dB] 00081 const float MAX_DB_; // 縦軸の目盛の最大値 [dB] 00082 const float DB1_; // 1 dB に対応する pixels 数 00083 const float Y_SPACE_; // 縦軸の目盛線の間隔に対応する pixels 数 00084 const float FS_; // 標本化周波数 00085 const uint32_t LINE_COLOR_; 00086 const uint32_t AXIS_COLOR_; 00087 const uint32_t BACK_COLOR_; 00088 00089 // 丸め 00090 int Round(float x) { return x + 0.5f - (x < 0); } 00091 00092 // 10 のべき乗かどうかの検査 00093 bool PowersOf10(float x) 00094 { return fabsf(log10f(x) - Round(log10f(x))) < 0.01f; } 00095 00096 // disallow copy constructor and assignment operator 00097 FrqRespDrawer(const FrqRespDrawer&); 00098 FrqRespDrawer& operator=(const FrqRespDrawer&); 00099 }; 00100 } 00101 #endif // F746_FRQ_RESP_DRAWER_HPP
Generated on Thu Jul 21 2022 23:30:51 by
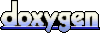