
Output the audio signal with filtering by IIR filter in the *.wav file on the SD card using onboard CODEC. SD カードの *.wav ファイルのオーディオ信号を遮断周波数可変の IIR フィルタを通して,ボードに搭載されているCODEC で出力する.
Dependencies: BSP_DISCO_F746NG F746_GUI LCD_DISCO_F746NG SDFileSystem_Warning_Fixed TS_DISCO_F746NG mbed FrequencyResponseDrawer F746_SAI_IO Array_Matrix
BilinearDesignLH.hpp
00001 //------------------------------------------------------------------------------ 00002 // Design of Butterworth LPF and HPF using bilinear transform -- Header 00003 // 00004 // 2016/03/31, Copyright (c) 2016 MIKAMI, Naoki 00005 //------------------------------------------------------------------------------ 00006 00007 #ifndef BILINEAR_BUTTERWORTH_HPP 00008 #define BILINEAR_BUTTERWORTH_HPP 00009 00010 #include "mbed.h" 00011 #include <complex> // requisite 00012 00013 namespace Mikami 00014 { 00015 typedef complex<float> Complex; // define "Complex" 00016 00017 class BilinearDesign 00018 { 00019 public: 00020 struct Coefs { float a1, a2, b1, b2; }; 00021 enum Type { LPF, HPF }; 00022 00023 // Constructor 00024 BilinearDesign(int order, float fs) 00025 : PI_FS_(PI_/fs), ORDER_(order) 00026 { 00027 sP_ = new Complex[order/2]; 00028 zP_ = new Complex[order/2]; 00029 ck_ = new Coefs[order/2]; 00030 } 00031 00032 // Destractor 00033 ~BilinearDesign() 00034 { 00035 delete[] sP_; 00036 delete[] zP_; 00037 delete[] ck_; 00038 } 00039 00040 // Execution of design 00041 void Execute(float fc, Type pb, Coefs c[], float& g); 00042 00043 private: 00044 static const float PI_ = 3.1415926536f; 00045 const float PI_FS_; 00046 const int ORDER_; 00047 00048 Complex* sP_; // Poles on s-plane 00049 Complex* zP_; // Poles on z-plane 00050 Coefs* ck_; // Coefficients of transfer function for cascade form 00051 float gain_; // Gain factor for cascade form 00052 00053 void Butterworth(); 00054 void Bilinear(float fc); 00055 void ToCascade(Type pb); 00056 void GetGain(Type pb); 00057 void GetCoefs(Coefs c[], float& gain); 00058 }; 00059 } 00060 #endif // BILINEAR_BUTTERWORTH_HPP
Generated on Wed Jul 27 2022 22:49:01 by
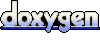