
Version using MEMS microphone and CODEC for the program "F746_RealtimeSpectrumAnalyzer". "F746_RealtimeSpectrumAnalyzer" の入力を MEMS のマイクと CODEC に変更.このプログラムは Tomona Nanase さんが作成し DISCO-F746NG_Oscilloscope の名前で登録しているプログラムで, CODEC を使って入力する部分を参考にして作成.このプログラムの説明は,CQ出版社のインターフェース誌,2016年4月号に掲載.
Dependencies: BSP_DISCO_F746NG BUTTON_GROUP LCD_DISCO_F746NG TS_DISCO_F746NG UIT_FFT_Real mbed
SpectrumDisplay.cpp
00001 //------------------------------------------------------- 00002 // Class for display spectrum 00003 // 00004 // 2016/01/10, Copyright (c) 2015 MIKAMI, Naoki 00005 //------------------------------------------------------- 00006 00007 #include "SpectrumDisplay.hpp" 00008 00009 namespace Mikami 00010 { 00011 SpectrumDisplay::SpectrumDisplay( 00012 LCD_DISCO_F746NG &lcd, 00013 int nFft, int x0, int y0, float offset, 00014 float db1, int bin, float maxDb, int fs, 00015 uint32_t axisColor, uint32_t lineColor, 00016 uint32_t backColor) 00017 : N_FFT_(nFft), X0_(x0), Y0_(y0), OFFSET_(offset), 00018 DB1_(db1), BIN_(bin), MAX_DB_(maxDb), 00019 FS_(fs), AXIS_COLOR_(axisColor), 00020 LINE_COLOR_(lineColor), BACK_COLOR_(backColor), 00021 lcd_(lcd) 00022 { 00023 AxisX(); 00024 AxisY(); 00025 } 00026 00027 void SpectrumDisplay::Draw(float db[]) 00028 { 00029 static const int TOP = Y0_ - (int)(MAX_DB_*DB1_); 00030 lcd_.SetTextColor(BACK_COLOR_); 00031 lcd_.FillRect(X0_+1, Y0_-(int)(MAX_DB_*DB1_), 00032 N_FFT_*BIN_/2, MAX_DB_*DB1_); 00033 00034 float h = ((db[1] + OFFSET_) > 0)? db[1] + OFFSET_ : 0; 00035 if (h > MAX_DB_) h = MAX_DB_; 00036 int y1 = Y0_ - (int)(h*DB1_); 00037 lcd_.SetTextColor(LINE_COLOR_); 00038 for (int n=1; n<N_FFT_/2; n++) 00039 { 00040 float h2 = ((db[n+1] + OFFSET_) > 0)? db[n+1] + OFFSET_ : 0; 00041 if (h2 > MAX_DB_) h2 = MAX_DB_; 00042 int y2 = Y0_ - (int)(h2*DB1_); 00043 00044 if ( (y1 <= TOP) && (y2 <= TOP) ) 00045 { // Out of displaying boundaries 00046 lcd_.SetTextColor(LCD_COLOR_RED); 00047 lcd_.DrawHLine(X0_+n, TOP, 1); 00048 lcd_.SetTextColor(LINE_COLOR_); 00049 } 00050 else 00051 lcd_.DrawLine(X0_+n, y1, X0_+n+1, y2); 00052 if (y1 <= TOP) lcd_.DrawPixel(X0_+n, y1, LCD_COLOR_RED); 00053 y1 = y2; 00054 } 00055 lcd_.SetTextColor(AXIS_COLOR_); 00056 lcd_.DrawLine(X0_, Y0_, X0_+BIN_*N_FFT_/2, Y0_); 00057 } 00058 00059 // Clear Spectrum 00060 void SpectrumDisplay::Clear() 00061 { 00062 lcd_.SetTextColor(BACK_COLOR_); 00063 lcd_.FillRect(X0_+1, Y0_-(int)(MAX_DB_*DB1_), 00064 N_FFT_*BIN_/2, MAX_DB_*DB1_); 00065 } 00066 00067 // x-axis 00068 void SpectrumDisplay::AxisX() 00069 { 00070 lcd_.SetFont(&Font12); 00071 00072 lcd_.SetTextColor(AXIS_COLOR_); 00073 lcd_.DrawLine(X0_, Y0_, X0_+BIN_*N_FFT_/2, Y0_); 00074 float dx = BIN_*(N_FFT_*1000.0f)/(float)FS_; 00075 for (int n=0; n<=(FS_/1000)/2; n++) 00076 { 00077 int xTick = X0_ + (int)(dx*n + 0.5f); 00078 char s[5]; 00079 sprintf(s, "%d", n); 00080 DrawString(xTick-3, Y0_+10, s); 00081 lcd_.DrawLine(xTick, Y0_, xTick, Y0_+5); 00082 } 00083 DrawString(X0_+74, Y0_+24, "Frequency [kHz]"); 00084 } 00085 00086 // y-axis 00087 void SpectrumDisplay::AxisY() 00088 { 00089 lcd_.SetFont(&Font12); 00090 00091 lcd_.SetTextColor(AXIS_COLOR_); 00092 lcd_.DrawLine(X0_, Y0_+5, X0_, Y0_-(int)(MAX_DB_*DB1_)); 00093 for (int n=0; n<=(int)MAX_DB_; n+=20) 00094 { 00095 int yTick = Y0_-(int)(DB1_*n); 00096 char s[5]; 00097 sprintf(s, "%3d", n); 00098 DrawString(X0_-30, yTick-5, s); 00099 lcd_.DrawLine(X0_-5, yTick, X0_, yTick); 00100 } 00101 DrawString(X0_-27, Y0_-(int)(DB1_*MAX_DB_)-18, "[dB]"); 00102 } 00103 } 00104
Generated on Sun Jul 17 2022 12:37:24 by
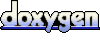