
Output the audio signal (*.bin) with filtering by IIR filter in the SD card using onboard CODEC. For *.wav file, F746_SD_WavPlayer and F746_SD_GraphicEqualiser are published on mbed. SD カードのオーディオ信号 (*.bin) を遮断周波数可変の IIR フィルタを通して,ボードに搭載されているCODEC で出力する.*.wav 形式のファイル用には,F746_SD_WavPlayer と F746_SD_GraphicEqualiser を mbed で公開している.
Dependencies: BSP_DISCO_F746NG_patch_fixed F746_GUI LCD_DISCO_F746NG SDFileSystem_Warning_Fixed TS_DISCO_F746NG mbed
FrquencyResponseDrawer.hpp
00001 //----------------------------------------------------------- 00002 // 周波数が対数スケールの周波数特性を描画するクラス 00003 // FrqRespDrawer class Header 00004 // 00005 // 2016/04/17, Copyright (c) 2016 MIKAMI, Naoki 00006 //----------------------------------------------------------- 00007 00008 #ifndef F746_FRQ_RESP_DRAWER_HPP 00009 #define F746_FRQ_RESP_DRAWER_HPP 00010 00011 #include "NumericLabel.hpp" 00012 #include "FrequancyResponseBase.hpp" 00013 00014 namespace Mikami 00015 { 00016 class FrqRespDrawer 00017 { 00018 public: 00019 // Constructor 00020 FrqRespDrawer(uint16_t org, float min, float max, uint16_t dec, 00021 uint16_t orgY, float minDb, float maxDb, uint16_t db10, 00022 float fs, 00023 uint32_t lineColor = 0xFF00B0FF, 00024 uint32_t axisColor = LCD_COLOR_LIGHTGRAY, 00025 uint32_t backColor = GuiBase::ENUM_BACK) 00026 : lcd_(GuiBase::GetLcdPtr()), 00027 ORG_(org), MIN_(min), MAX_(max), DEC_(dec), 00028 ORGY_(orgY), MIN_DB_(minDb), MAX_DB_(maxDb), DB10_(db10), 00029 FS_(fs), 00030 LINE_COLOR_(lineColor), 00031 AXIS_COLOR_(axisColor), 00032 BACK_COLOR_(backColor), 00033 DB1_(db10*0.1f) {} 00034 00035 // 周波数に対応する x 座標値の取得 00036 int X(float frq) 00037 { return Round(ORG_ + DEC_*log10f(frq/MIN_)); } 00038 00039 // x 座標値を周波数に変換 00040 float PosToFrq(uint16_t x) 00041 { return MIN_*powf(10.0f, (x - ORG_)/(float)DEC_); } 00042 00043 // 目盛線の描画 00044 void DrawAxis(); 00045 00046 // 横軸の目盛値の表示用 00047 void DrawCharX(uint32_t frq, int offsetY, 00048 const char str[], sFONT &fonts = Font12, 00049 uint32_t textColor = LCD_COLOR_WHITE) 00050 { Label frequency(X(frq), ORGY_+offsetY, str, Label::CENTER); } 00051 00052 // 縦軸の目盛値の表示 00053 void DrawNumericY(int offsetX, int offsetY, int count, 00054 uint16_t d_dB, const char fmt[], sFONT &fonts = Font12, 00055 uint32_t textColor = LCD_COLOR_WHITE); 00056 00057 // 周波数特性のグラフの描画 00058 void DrawGraph(FrequencyResponse &frqResp, uint32_t color); 00059 void DrawGraph(FrequencyResponse &frqResp) 00060 { DrawGraph(frqResp, LINE_COLOR_);} 00061 00062 // 消去 00063 void Erase(); 00064 00065 private: 00066 LCD_DISCO_F746NG *lcd_; 00067 const uint16_t ORG_; // 横軸の目盛の最小値に対応する位置 00068 const float MIN_; // 横軸の目盛の最小値 00069 const float MAX_; // 横軸の目盛の最大値 00070 const uint16_t DEC_; // 周波数の 10 倍に対応する長さ (pixels) 00071 const uint16_t ORGY_; // 縦軸の目盛の最小値に対応する位置 00072 const float MIN_DB_; // 縦軸の目盛の最小値 [dB] 00073 const float MAX_DB_; // 縦軸の目盛の最大値 [dB] 00074 const uint16_t DB10_; // 10 dB 対応する長さ (pixels) 00075 const float FS_; // 標本化周波数 00076 const uint32_t LINE_COLOR_; 00077 const uint32_t AXIS_COLOR_; 00078 const uint32_t BACK_COLOR_; 00079 const float DB1_; 00080 00081 // 丸め 00082 int Round(float x) { return x + 0.5f - (x < 0); } 00083 00084 // 10 のべき乗かどうかの検査 00085 bool PowersOf10(float x) 00086 { return fabsf(log10f(x) - Round(log10f(x))) < 0.01f; } 00087 00088 // disallow copy constructor and assignment operator 00089 FrqRespDrawer(const FrqRespDrawer&); 00090 FrqRespDrawer& operator=(const FrqRespDrawer&); 00091 }; 00092 } 00093 #endif // F746_FRQ_RESP_DRAWER_HPP
Generated on Wed Jul 13 2022 03:37:09 by
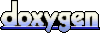