
Output the audio signal (*.bin) with filtering by IIR filter in the SD card using onboard CODEC. For *.wav file, F746_SD_WavPlayer and F746_SD_GraphicEqualiser are published on mbed. SD カードのオーディオ信号 (*.bin) を遮断周波数可変の IIR フィルタを通して,ボードに搭載されているCODEC で出力する.*.wav 形式のファイル用には,F746_SD_WavPlayer と F746_SD_GraphicEqualiser を mbed で公開している.
Dependencies: BSP_DISCO_F746NG_patch_fixed F746_GUI LCD_DISCO_F746NG SDFileSystem_Warning_Fixed TS_DISCO_F746NG mbed
FileSelector.hpp
00001 //-------------------------------------------------------------- 00002 // FileSelector class 00003 // SD カード内のファイル名の一覧を表示し,ファイルを選択する 00004 // 00005 // 2016/04/10, Copyright (c) 2016 MIKAMI, Naoki 00006 //-------------------------------------------------------------- 00007 00008 #ifndef FILE_SELECTOR_HPP 00009 #define FILE_SELECTOR_HPP 00010 00011 #include "mbed.h" 00012 #include "Label.hpp" 00013 #include "ButtonGroup.hpp" 00014 #include "SD_BinaryReader.hpp" 00015 #include "SDFileSystem.h" 00016 #include <algorithm> // sort() で使用 00017 #include <string> 00018 00019 namespace Mikami 00020 { 00021 class FileSelector 00022 { 00023 public: 00024 FileSelector(uint8_t x0, uint8_t y0, int maxFiles, 00025 int maxNameLength, SD_BinaryReader &reader) 00026 : X_(x0), Y_(y0), W_H_(24), V_L_(36), 00027 MAX_FILES_(maxFiles), MAX_NAME_LENGTH_(maxNameLength), 00028 BASE_COLOR_(0xFF80FFA0), TOUCHED_COLOR_(0xFF80FFFF), 00029 fileNames_(new string[maxFiles]), 00030 sortedFileNames_(new string[maxFiles]), 00031 lcd_(GuiBase::GetLcdPtr()), 00032 sdReader_(reader), prev_(-1) {} 00033 00034 ~FileSelector() 00035 { 00036 for (int n=0; n<fileCount_; n++) 00037 delete fileNameLabels_[n]; 00038 delete[] fileNameLabels_; 00039 delete[] rect_; 00040 delete[] nonString_; 00041 delete[] sortedFileNames_; 00042 delete[] fileNames_; 00043 } 00044 bool CreateTable() 00045 { 00046 DIR* dp = opendir("/sd"); 00047 fileCount_ = 0; 00048 if (dp != NULL) 00049 { 00050 dirent* entry; 00051 for (int n=0; n<256; n++) 00052 { 00053 entry = readdir(dp); 00054 if (entry == NULL) break; 00055 00056 string strName = entry->d_name; 00057 if ( (strName.find(".bin") != string::npos) || 00058 (strName.find(".BIN") != string::npos) ) 00059 { 00060 sdReader_.Open(strName); // ファイルオープン 00061 // 小さすぎるファイルは除外 00062 if ((sdReader_.ReadSize()) > 4096) 00063 { 00064 fileNames_[fileCount_] = strName; 00065 fileCount_++; 00066 } 00067 sdReader_.Close(); 00068 } 00069 if (fileCount_ >= MAX_FILES_) break; 00070 } 00071 closedir(dp); 00072 } 00073 else 00074 return false; 00075 00076 if (fileCount_ == 0) return false; 00077 00078 nonString_ = new string[fileCount_]; 00079 for (int n=0; n<fileCount_; n++) nonString_[n] = ""; 00080 rect_ = new ButtonGroup(X_, Y_, W_H_, W_H_, fileCount_, 00081 nonString_, 0, V_L_-W_H_, 1, 00082 -1, Font12, 0, GuiBase::ENUM_BACK, 00083 BASE_COLOR_, TOUCHED_COLOR_); 00084 for (int n=0; n<fileCount_; n++) rect_->Erase(n); 00085 CreateLabels(); 00086 00087 return true; 00088 } 00089 00090 // ファイルを選択する 00091 bool Select(string &fileName) 00092 { 00093 int n; 00094 if (rect_->GetTouchedNumber(n)) 00095 { 00096 fileNameLabels_[n]->Draw(GetFileNameNoExt(n), TOUCHED_COLOR_); 00097 if ((prev_ >= 0) && (prev_ != n)) 00098 fileNameLabels_[prev_]->Draw(GetFileNameNoExt(prev_)); 00099 prev_ = n; 00100 fileName = sortedFileNames_[n]; 00101 return true; 00102 } 00103 else 00104 return false; 00105 } 00106 00107 // ファイルの一覧の表示 00108 void DisplayFileList(bool sort = true) 00109 { 00110 for (int n=0; n<fileCount_; n++) 00111 sortedFileNames_[n] = fileNames_[n]; 00112 if (sort) 00113 std::sort(sortedFileNames_, sortedFileNames_+fileCount_); 00114 00115 Erase(MAX_NAME_LENGTH_*((sFONT *)(&Font16))->Width, 270-Y_); 00116 rect_->DrawAll(); 00117 for (int n=0; n<fileCount_; n++) 00118 fileNameLabels_[n]->Draw(GetFileNameNoExt(n)); 00119 } 00120 00121 void Erase(uint16_t width, uint16_t height, 00122 uint32_t color = GuiBase::ENUM_BACK) 00123 { 00124 lcd_->SetTextColor(color); 00125 lcd_->FillRect(X_, Y_, width, height); 00126 } 00127 00128 private: 00129 const uint8_t X_, Y_, W_H_, V_L_; 00130 const int MAX_FILES_; 00131 const int MAX_NAME_LENGTH_; 00132 const uint32_t BASE_COLOR_; 00133 const uint32_t TOUCHED_COLOR_; 00134 00135 string *fileNames_; 00136 string *sortedFileNames_; 00137 string *nonString_; 00138 ButtonGroup *rect_; 00139 Label **fileNameLabels_; 00140 LCD_DISCO_F746NG *lcd_; 00141 SD_BinaryReader &sdReader_; 00142 int fileCount_; 00143 int prev_; 00144 00145 // Label を生成 00146 void CreateLabels() 00147 { 00148 fileNameLabels_ = new Label *[fileCount_+1]; 00149 for (int n=0; n<fileCount_; n++) 00150 fileNameLabels_[n] = new Label(X_+30, Y_+5+V_L_*n, "", 00151 Label::LEFT, Font16, 00152 BASE_COLOR_); 00153 } 00154 00155 // 拡張子を削除した文字列を取得 00156 string GetFileNameNoExt(int n) 00157 { 00158 string name = sortedFileNames_[n]; 00159 name.erase(name.find(".")); 00160 return name.substr(0, MAX_NAME_LENGTH_); 00161 } 00162 00163 // disallow copy constructor and assignment operator 00164 FileSelector(const FileSelector&); 00165 FileSelector& operator=(const FileSelector&); 00166 }; 00167 } 00168 #endif // FILE_SELECTOR_HPP
Generated on Wed Jul 13 2022 03:37:09 by
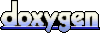