
Output the audio signal (*.bin) with filtering by IIR filter in the SD card using onboard CODEC. For *.wav file, F746_SD_WavPlayer and F746_SD_GraphicEqualiser are published on mbed. SD カードのオーディオ信号 (*.bin) を遮断周波数可変の IIR フィルタを通して,ボードに搭載されているCODEC で出力する.*.wav 形式のファイル用には,F746_SD_WavPlayer と F746_SD_GraphicEqualiser を mbed で公開している.
Dependencies: BSP_DISCO_F746NG_patch_fixed F746_GUI LCD_DISCO_F746NG SDFileSystem_Warning_Fixed TS_DISCO_F746NG mbed
DesignerDrawer.cpp
00001 //------------------------------------------------------------------------------ 00002 // IIR フィルタを双一次 z 変換で設計し,その周波数特性を描画するためのクラス 00003 // 00004 // 2016/04/17, Copyright (c) 2016 MIKAMI, Naoki 00005 //------------------------------------------------------------------------------ 00006 00007 #include "DesignerDrawer.hpp" 00008 00009 namespace Mikami 00010 { 00011 // Constructor 00012 DesignerDrawer::DesignerDrawer(uint16_t x0, uint16_t y0, 00013 uint16_t db10, int fs, int order, 00014 float fc, uint16_t fL, uint16_t fH, 00015 BilinearDesign::Type lpHp) 00016 : lcd_(GuiBase::GetLcdPtr()), ts_(GuiBase::GetTsPtr()), 00017 X0_(x0), Y0_(y0), DB10_(db10), ORDER_(order), 00018 CURSOR_Y0_(y0+1-db10*6), CURSOR_LENGTH_(db10*6-1), 00019 LOWER_F_(fL), HIGHER_F_(fH), 00020 CURSOR_COLOR_(0xFFE000D0), CURSOR_TOUCHED_COLOR_(0xFFFF80FF) 00021 { 00022 drawerObj_ = new FrqRespDrawer(x0, 100.0f, 8000.0f, 150, 00023 y0, -60, 0, db10, fs); 00024 00025 // 双一次 z 変換による IIR フィルタの設計 00026 designObj_ = new BilinearDesign(order, fs); 00027 coefs_ = new BilinearDesign::Coefs[order/2]; 00028 ck_ = (Biquad::Coefs *)coefs_; 00029 00030 fC_ = fc; // 最初に与える遮断周波数 00031 designObj_->Execute(fC_, lpHp, coefs_, g0_); 00032 frqResp_.SetParams(ORDER_, g0_, ck_); 00033 00034 // 周波数特性の描画 00035 lblFrq_ = new NumericLabel<int>(110, 30); 00036 DrawResponse(); 00037 tp_ = new TouchPanelDetectorX( 00038 drawerObj_->X(LOWER_F_), drawerObj_->X(HIGHER_F_), 00039 CURSOR_Y0_, y0); 00040 lp_ = lpHp; 00041 cursorRedraw_ = false; 00042 } 00043 00044 DesignerDrawer::~DesignerDrawer() 00045 { 00046 delete tp_; 00047 delete lblFrq_; 00048 delete coefs_; 00049 delete designObj_; 00050 delete drawerObj_; 00051 } 00052 00053 // フィルタの再設計と周波数特性の再描画 00054 bool DesignerDrawer::ReDesignAndDraw(Biquad::Coefs *ck, float &g0, 00055 BilinearDesign::Type lpHp) 00056 { 00057 bool changed = (lpHp != lp_) ? true : false; 00058 bool tch = tp_->IsTouched(cursorX_, cursorX_); 00059 if (tch || changed) 00060 { 00061 int newFc = Frq10(drawerObj_->PosToFrq(cursorX_)); 00062 newFc = (newFc > HIGHER_F_) ? HIGHER_F_ : newFc; 00063 if ((abs(newFc - fC_) >= 10) || changed) 00064 { 00065 fC_ = newFc; 00066 lblFrq_->Draw("Cutoff frequency = %4d Hz", newFc); 00067 designObj_->Execute(newFc, lpHp, coefs_, g0_); 00068 GetCoefficients(ck, g0); 00069 00070 frqResp_.SetParams(ORDER_, g0_, ck_); 00071 drawerObj_->Erase(); 00072 drawerObj_->DrawAxis(); // 目盛線の描画 00073 drawerObj_->DrawGraph(frqResp_); // 周波数特性のグラフのカーブを描画する 00074 00075 if (tch) // カーソルの移動 00076 { 00077 lcd_->SetTextColor(CURSOR_TOUCHED_COLOR_); 00078 lcd_->DrawVLine(cursorX_, CURSOR_Y0_, CURSOR_LENGTH_); 00079 } 00080 cursorRedraw_ = true; 00081 oldCursorX_ = cursorX_; 00082 lp_ = lpHp; 00083 return true; 00084 } 00085 } 00086 00087 if (!tch && cursorRedraw_) // カーソルを元の色に戻す 00088 { 00089 lcd_->SetTextColor(CURSOR_COLOR_); 00090 lcd_->DrawVLine(oldCursorX_, CURSOR_Y0_, CURSOR_LENGTH_); 00091 cursorRedraw_ = false; 00092 } 00093 return false; 00094 } 00095 00096 // 周波数特性の描画 00097 void DesignerDrawer::DrawResponse() 00098 { 00099 lblFrq_->Draw("Cutoff frequency = %4d Hz", fC_); 00100 DrawAxisNum(); // 目盛値の描画 00101 drawerObj_->DrawAxis(); // 目盛線の描画 00102 drawerObj_->DrawGraph(frqResp_); // 周波数特性のカーブの描画 00103 00104 cursorX_ = drawerObj_->X(fC_); 00105 lcd_->SetTextColor(CURSOR_COLOR_); 00106 lcd_->DrawVLine(cursorX_, CURSOR_Y0_, CURSOR_LENGTH_); 00107 } 00108 00109 // フィルタ係数の取得 00110 void DesignerDrawer::GetCoefficients(Biquad::Coefs *c, float &g0) 00111 { 00112 for (int k=0; k<ORDER_/2; k++) c[k] = ck_[k]; 00113 g0 = g0_; 00114 } 00115 00116 // 周波数を 10, 20, 50, 100 Hz の倍数にする 00117 int DesignerDrawer::Frq10(float f) 00118 { 00119 if (f < 1000) 00120 return ((int)(f/10.0f + 0.5f))*10; 00121 if (f < 2000) 00122 return ((int)(f/20.0f + 0.5f))*20; 00123 if (f < 3000) 00124 return ((int)(f/50.0f + 0.5f))*50; 00125 else 00126 return ((int)(f/100.0f + 0.5f))*100; 00127 } 00128 00129 // 周波数特性の目盛値の描画 00130 void DesignerDrawer::DrawAxisNum() 00131 { 00132 const int16_t OFS = 6; 00133 drawerObj_->DrawCharX(100, OFS, "0.1"); 00134 drawerObj_->DrawCharX(300, OFS, "0.3"); 00135 drawerObj_->DrawCharX(1000, OFS, "1.0"); 00136 drawerObj_->DrawCharX(3000, OFS, "3.0"); 00137 drawerObj_->DrawCharX(8000, OFS, "8.0"); 00138 00139 Label l_frq(drawerObj_->X(900), Y0_+20, "Frequency [kHz]", 00140 Label::CENTER); 00141 drawerObj_->DrawNumericY(-24, -6, 4, 20, "%3d"); // 縦軸の目盛は 20 dB 間隔 00142 Label l_dB(X0_-24, Y0_-DB10_*6-20, "[dB]"); 00143 } 00144 } 00145
Generated on Wed Jul 13 2022 03:37:09 by
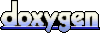