
Realtime sound spectrogram using FFT or linear prediction. Spectrogram is displayed on the display of PC. リアルタイム・スペクトログラム.解析の手法:FFT,線形予測法.スペクトログラムは PC のディスプレー装置に表示される.PC 側のプログラム:F446_Spectrogram.
Dependencies: Array_Matrix mbed SerialTxRxIntr F446_AD_DA UIT_FFT_Real
LinearPrediction.hpp
00001 //----------------------------------------------------- 00002 // Class for linear prediction (Header) 00003 // 00004 // 2017/02/11, Copyright (c) 2017 MIKAMI, Naoki 00005 //----------------------------------------------------- 00006 00007 #ifndef LINEAR_PREDICTION_HPP 00008 #define LINEAR_PREDICTION_HPP 00009 00010 #include "Array.hpp" 00011 00012 namespace Mikami 00013 { 00014 class LinearPred 00015 { 00016 public: 00017 LinearPred(int nData, int order); 00018 ~LinearPred() {} 00019 bool Execute(const float x[], float a[], float &em); 00020 private: 00021 const uint16_t N_DATA_; 00022 00023 uint16_t order_; 00024 00025 Array<float> r_; // for auto-correlation 00026 Array<float> k_; // for PARCOR coefficients 00027 Array<float> am_; // working area 00028 00029 void AutoCorr(const float x[]); 00030 bool Durbin(float a[], float &em); 00031 00032 // disallow copy constructor and assignment operator 00033 LinearPred(const LinearPred& ); 00034 LinearPred& operator=(const LinearPred& ); 00035 }; 00036 } 00037 #endif // LINEAR_PREDICTION_HPP
Generated on Mon Jul 25 2022 07:38:49 by
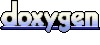