
Realtime sound spectrogram using FFT or linear prediction. Spectrogram is displayed on the display of PC. リアルタイム・スペクトログラム.解析の手法:FFT,線形予測法.スペクトログラムは PC のディスプレー装置に表示される.PC 側のプログラム:F446_Spectrogram.
Dependencies: Array_Matrix mbed SerialTxRxIntr F446_AD_DA UIT_FFT_Real
AnalyzerBase.hpp
00001 //------------------------------------------------------- 00002 // Base abstract class for spectrum analysis (Header) 00003 // 00004 // 2018/11/04, Copyright (c) 2018 MIKAMI, Naoki 00005 //------------------------------------------------------- 00006 00007 #ifndef BASE_ANALYZER_HPP 00008 #define BASE_ANALYZER_HPP 00009 00010 #include "Array.hpp" 00011 #include "fftReal.hpp" 00012 #include "Hamming.hpp" 00013 00014 namespace Mikami 00015 { 00016 class AnalyzerBase 00017 { 00018 public: 00019 // nData: Number of data to be analyzed 00020 // nFft: Number of FFT points 00021 // nUse: FFT, cepstrum: window width + zero padding 00022 // Linear prediction: window width 00023 AnalyzerBase(int nData, int nFft, int nUse); 00024 virtual ~AnalyzerBase() {} 00025 void Execute(const float xn[], float db[]); 00026 // 高域強調の程度を決める定数の設定(b1 = 1 で差分,b1 = 0 で高域強調なし) 00027 void SetHighEmphasizer(float b1) { b1_ = b1; } 00028 00029 protected: 00030 const int N_DATA_; 00031 const int N_FFT_; 00032 00033 FftReal fft_; 00034 00035 float Norm(Complex x) 00036 { return x.real()*x.real() + x.imag()*x.imag(); } 00037 00038 private: 00039 HammingWindow wHm_; 00040 float b1_; 00041 00042 Array<float> xData_; // data to be analyzed 00043 Array<float> wData_; // windowd data 00044 00045 virtual void Analyze(const float wData[], float db[]) = 0; 00046 00047 // disallow copy constructor and assignment operator 00048 AnalyzerBase(const AnalyzerBase& ); 00049 AnalyzerBase& operator=(const AnalyzerBase& ); 00050 }; 00051 } 00052 #endif // BASE_ANALYZER_HPP
Generated on Mon Jul 25 2022 07:38:49 by
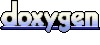