
FFT によるスペクトル解析器
Dependencies: Array_Matrix mbed SerialTxRxIntr UIT_FFT_Real DSP_ADDA
DoubleBuffer.hpp
00001 //-------------------------------------------------------- 00002 // ダブル・バッファの template クラス 00003 // 内部のバッファは通常の配列を使用 00004 // 00005 // 2019/11/22, Copyright (c) 2019 MIKAMI, Naoki 00006 //-------------------------------------------------------- 00007 00008 #ifndef DOUBLE_BUFFER_2DARRAY_HPP 00009 #define DOUBLE_BUFFER_2DARRAY_HPP 00010 00011 template<class T, int N> class DoubleBuffer 00012 { 00013 public: 00014 // コンストラクタ 00015 explicit DoubleBuffer(T initialValue) 00016 : ping_(0), pong_(1), index_(0), full_(false) 00017 { 00018 for (int k=0; k<2; k++) 00019 for (int n=0; n<N; n++) buf_[k][n] = initialValue; 00020 } 00021 00022 // データを格納 00023 void Store(T data) { buf_[ping_][index_++] = data; } 00024 00025 // 出力バッファからデータの取り出し 00026 T Get(int n) const { return buf_[pong_][n]; } 00027 00028 // バッファが満杯でバッファを切り替える 00029 void IfFullSwitch() 00030 { 00031 if (index_ < N) return; 00032 00033 ping_ ^= 0x1; // バッファ切換えのため 00034 pong_ ^= 0x1; // バッファ切換えのため 00035 index_ = 0; 00036 full_ = true; 00037 } 00038 00039 // バッファが満杯で,true を返す 00040 bool IsFull() 00041 { 00042 bool temp = full_; 00043 if (full_) full_ = false; 00044 return temp; 00045 } 00046 00047 private: 00048 T buf_[2][N]; // 標本化したデータのバッファ 00049 int ping_, pong_; // バッファ切替用 00050 int index_; // 入力データのカウンタ 00051 bool full_; // 満杯の場合 true 00052 00053 // コピー・コンストラクタおよび代入演算子の禁止のため 00054 DoubleBuffer(const DoubleBuffer&); 00055 DoubleBuffer& operator=(const DoubleBuffer&); 00056 }; 00057 #endif // DOUBLE_BUFFER_2DARRAY_HPP 00058
Generated on Fri Jul 15 2022 00:11:09 by
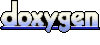