
CQエレクトロニクス・セミナ「実習・マイコンを動かしながら学ぶディジタル・フィルタ」で使うプログラムを,入力として STM32F746 の内蔵 ADC を使うように変更したもの. http://seminar.cqpub.co.jp/ccm/ES18-0020
Dependencies: mbed Array_Matrix BSP_DISCO_F746NG LCD_DISCO_F746NG TS_DISCO_F746NG
F746_ADC_Interrupt.hpp
00001 //---------------------------------------------------------- 00002 // Simultanuous AD Conversion by interrupt using 00003 // ADC1 and ADC3 on STM32F746 ---- Header 00004 // 00005 // STM32F746 の ADC1, ADC3 を使って同時に AD 変換を開始し, 00006 // 割り込みによりアナログ信号を入力するクラス(ヘッダ) 00007 // AdcDual クラスの派生クラス 00008 // 00009 // 2017/08/16, Copyright (c) 2017 MIKAMI, Naoki 00010 //---------------------------------------------------------- 00011 00012 #ifndef F746_ADC_DUAL_INTERRUPT_HPP 00013 #define F746_ADC_DUAL_INTERRUPT_HPP 00014 00015 #include "F746_ADC.hpp" 00016 00017 namespace Mikami 00018 { 00019 class AdcDual_Intr : public AdcDual 00020 { 00021 public: 00022 explicit AdcDual_Intr(int frequency) : AdcDual(frequency) 00023 { ADC1->CR1 |= ADC_CR1_EOCIE; } 00024 00025 virtual ~AdcDual_Intr() {} 00026 00027 // -1.0f <= ad1, ad2 <= 1.0f 00028 virtual void Read(float &ad1, float &ad2) 00029 { 00030 ad1 = ToFloat(ADC1->DR); 00031 ad2 = ToFloat(ADC3->DR); 00032 } 00033 00034 // 0 <= ad1, ad2 <= 4095 00035 virtual void Read(uint16_t &ad1, uint16_t &ad2) 00036 { 00037 ad1 = ADC1->DR; 00038 ad2 = ADC3->DR; 00039 } 00040 00041 // Set interrupt vector 00042 void SetIntrVec(void (*Func)()) 00043 { 00044 NVIC_SetVector(ADC_IRQn, (uint32_t)Func); // See "cmsis_nvic.h" 00045 } 00046 00047 void EnableAdcIntr() 00048 { 00049 NVIC_EnableIRQ(ADC_IRQn); // See "core_cm7.h" 00050 } 00051 00052 void DisableAdcIntr() 00053 { 00054 NVIC_DisableIRQ(ADC_IRQn); // See "core_cm7.h" 00055 } 00056 00057 private: 00058 // for inhibition of copy constructor 00059 AdcDual_Intr(const AdcDual_Intr&); 00060 // for inhibition of substitute operator 00061 AdcDual_Intr& operator=(const AdcDual_Intr&); 00062 }; 00063 } 00064 #endif // F746_ADC_DUAL_INTERRUPT_HPP 00065
Generated on Sun Jul 17 2022 01:09:34 by
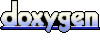