
Werkend EMG script
Dependencies: HIDScope MODSERIAL biquadFilter mbed
Fork of EMG by
main.cpp
00001 #include "mbed.h" 00002 #include "HIDScope.h" 00003 #include "MODSERIAL.h" 00004 #include "biquadFilter.h" 00005 00006 //Define objects 00007 AnalogIn EMG_left(A0); //Analog input 00008 AnalogIn EMG_right(A1); 00009 Ticker SampleEMG; 00010 Ticker ScopeTimer; 00011 Ticker serial; 00012 HIDScope scope(3); 00013 DigitalOut led(LED_RED); 00014 MODSERIAL pc(USBTX, USBRX); 00015 00016 00017 // Declaring variables 00018 double EMG_L_f_v1 = 0, EMG_L_f_v2 = 0; 00019 double EMG_L_fh=0; 00020 double EMG_left_value; 00021 double EMG_f1; 00022 double EMG_f2; 00023 double Threshold = 0.08; 00024 00025 // coëfficiënten 00026 const double BiGainEMG_Lh = 0.723601, BiGainEMG_Ll=0.983892; 00027 const double EMGh_a1 = -1.74355513773*BiGainEMG_Lh, EMGh_a2 = 0.80079826172*BiGainEMG_Lh, EMGh_b0 = 1.0*BiGainEMG_Lh, EMGh_b1 = -1.99697722433*BiGainEMG_Lh, EMGh_b2 = 1.0*BiGainEMG_Lh; //coefficients for high-pass filter 00028 const double EMGl_a1 = 1.96775103303*BiGainEMG_Ll, EMGl_a2 = 0.96827054038*BiGainEMG_Ll, EMGl_b0 = 1.0*BiGainEMG_Ll, EMGl_b1 = 1.99999993582*BiGainEMG_Ll, EMGl_b2 = 1.0*BiGainEMG_Ll; // coefficients for low-pass filter 00029 00030 // Filter creation 00031 biquadFilter EMG_highpass (EMGh_a1, EMGh_a2, EMGh_b0, EMGh_b1, EMGh_b2); // creates the high pass filter 00032 biquadFilter EMG_lowpass (EMGl_a1, EMGl_a2, EMGl_b0, EMGl_b1, EMGl_b2); // creates the low pass filter 00033 00034 // EMG filtering function 00035 void EMGfilter() 00036 { 00037 EMG_left_value = EMG_left.read(); 00038 EMG_f1 = EMG_highpass.step(EMG_left_value); 00039 EMG_f2 = EMG_lowpass.step(EMG_f1); 00040 } 00041 00042 // HIDScope 00043 void ScopeSend() 00044 { 00045 scope.set(0, EMG_left_value); 00046 scope.set(1, EMG_f1); 00047 scope.set(2, EMG_f2); 00048 scope.send(); 00049 } 00050 00051 // Serial communication 00052 void serial_() 00053 { 00054 pc.baud(115200); 00055 pc.printf("%d\n", EMG_L_fh); 00056 } 00057 00058 int main() 00059 { 00060 SampleEMG.attach(&EMGfilter, 0.002); 00061 ScopeTimer.attach(&ScopeSend, 0.002); 00062 while(1) 00063 { 00064 if (fabs(EMG_f2) > Threshold) 00065 { 00066 led.write(0); 00067 } 00068 00069 else 00070 { 00071 led.write(1); 00072 } 00073 } 00074 }
Generated on Fri Jul 29 2022 03:32:35 by
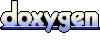