emw3162 driver for mbed os 5
Fork of emw3162-driver by
Embed:
(wiki syntax)
Show/hide line numbers
EMW3162Interface.cpp
00001 /* EMW3162 implementation of NetworkInterfaceAPI 00002 * Copyright (c) 2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 00018 #include "EMW3162Interface.h" 00019 00020 // Various timeouts for different EMW3162 operations 00021 #define EMW3162_CONNECT_TIMEOUT 15000 00022 #define EMW3162_SEND_TIMEOUT 500 00023 #define EMW3162_RECV_TIMEOUT 0 00024 #define EMW3162_MISC_TIMEOUT 500 00025 00026 00027 // EMW3162Interface implementation 00028 EMW3162Interface::EMW3162Interface(PinName tx, PinName rx, bool debug) 00029 : _esp(tx, rx, debug) 00030 { 00031 memset(_ids, 0, sizeof(_ids)); 00032 memset(_cbs, 0, sizeof(_cbs)); 00033 00034 _esp.attach(this, &EMW3162Interface::event); 00035 } 00036 00037 int EMW3162Interface::connect() 00038 { 00039 return 0; 00040 } 00041 int EMW3162Interface::connect(const char *ssid, const char *pass, nsapi_security_t security, 00042 uint8_t channel) 00043 { 00044 _esp.setTimeout(EMW3162_CONNECT_TIMEOUT); 00045 00046 if (!_esp.startup()) { 00047 return NSAPI_ERROR_DEVICE_ERROR; 00048 } 00049 00050 if (!_esp.dhcp(true)) { 00051 return NSAPI_ERROR_DHCP_FAILURE; 00052 } 00053 00054 if (!_esp.connect(ssid, pass)) { 00055 return NSAPI_ERROR_NO_CONNECTION; 00056 } 00057 00058 if (!_esp.getIPAddress()) { 00059 return NSAPI_ERROR_DHCP_FAILURE; 00060 } 00061 00062 return NSAPI_ERROR_OK; 00063 } 00064 00065 int EMW3162Interface::set_credentials(const char *ssid, const char *pass, nsapi_security_t security) 00066 { 00067 return 0; 00068 } 00069 00070 int EMW3162Interface::set_channel(uint8_t channel) 00071 { 00072 return NSAPI_ERROR_UNSUPPORTED; 00073 } 00074 00075 int EMW3162Interface::disconnect() 00076 { 00077 _esp.setTimeout(EMW3162_MISC_TIMEOUT); 00078 00079 if (!_esp.disconnect()) { 00080 return NSAPI_ERROR_DEVICE_ERROR; 00081 } 00082 00083 return 0; 00084 } 00085 00086 const char* EMW3162Interface::get_ip_address() 00087 { 00088 return _esp.getIPAddress(); 00089 } 00090 00091 const char* EMW3162Interface::get_mac_address() 00092 { 00093 return _esp.getMACAddress(); 00094 } 00095 00096 const char *EMW3162Interface::get_gateway() 00097 { 00098 return NULL; 00099 } 00100 00101 const char *EMW3162Interface::get_netmask() 00102 { 00103 return NULL; 00104 } 00105 00106 int8_t EMW3162Interface::get_rssi() 00107 { 00108 return 0; 00109 } 00110 00111 int EMW3162Interface::scan(WiFiAccessPoint *res, unsigned count) 00112 { 00113 return 0; 00114 } 00115 00116 struct EMW3162_socket { 00117 int id; 00118 int socketId; 00119 nsapi_protocol_t proto; 00120 bool connected; 00121 }; 00122 00123 int EMW3162Interface::socket_open(void **handle, nsapi_protocol_t proto) 00124 { 00125 // Look for an unused socket 00126 int id = -1; 00127 00128 for (int i = 1; i < EMW3162_SOCKET_COUNT; i++) { 00129 if (!_ids[i]) { 00130 id = i; 00131 _ids[i] = true; 00132 break; 00133 } 00134 } 00135 00136 if (id == -1) { 00137 return NSAPI_ERROR_NO_SOCKET; 00138 } 00139 00140 struct EMW3162_socket *socket = new struct EMW3162_socket; 00141 if (!socket) { 00142 return NSAPI_ERROR_NO_SOCKET; 00143 } 00144 00145 socket->id = id; 00146 socket->socketId = 0; 00147 socket->proto = proto; 00148 socket->connected = false; 00149 *handle = socket; 00150 return 0; 00151 } 00152 00153 int EMW3162Interface::socket_close(void *handle) 00154 { 00155 struct EMW3162_socket *socket = (struct EMW3162_socket *)handle; 00156 int err = 0; 00157 _esp.setTimeout(EMW3162_MISC_TIMEOUT); 00158 00159 if (!_esp.close(socket->socketId)) { 00160 err = NSAPI_ERROR_DEVICE_ERROR; 00161 } 00162 00163 _ids[socket->id] = false; 00164 delete socket; 00165 return err; 00166 } 00167 00168 int EMW3162Interface::socket_bind(void *handle, const SocketAddress &address) 00169 { 00170 return NSAPI_ERROR_UNSUPPORTED; 00171 } 00172 00173 int EMW3162Interface::socket_listen(void *handle, int backlog) 00174 { 00175 return NSAPI_ERROR_UNSUPPORTED; 00176 } 00177 00178 int EMW3162Interface::socket_connect(void *handle, const SocketAddress &addr) 00179 { 00180 struct EMW3162_socket *socket = (struct EMW3162_socket *)handle; 00181 _esp.setTimeout(EMW3162_MISC_TIMEOUT); 00182 00183 const char *proto = (socket->proto == NSAPI_UDP) ? "UNICAST" : "CLIENT"; 00184 socket -> socketId = _esp.open(proto, socket->id, addr.get_ip_address(), addr.get_port()); 00185 if (!(socket -> socketId)) { 00186 return NSAPI_ERROR_DEVICE_ERROR; 00187 } 00188 00189 socket->connected = true; 00190 return 0; 00191 } 00192 00193 int EMW3162Interface::socket_accept(void *handle, void **socket, SocketAddress *address) 00194 { 00195 return NSAPI_ERROR_UNSUPPORTED; 00196 } 00197 00198 int EMW3162Interface::socket_send(void *handle, const void *data, unsigned size) 00199 { 00200 struct EMW3162_socket *socket = (struct EMW3162_socket *)handle; 00201 _esp.setTimeout(EMW3162_SEND_TIMEOUT); 00202 00203 if (!_esp.send(socket->socketId, data, size)) { 00204 return NSAPI_ERROR_DEVICE_ERROR; 00205 } 00206 00207 return size; 00208 } 00209 00210 int EMW3162Interface::socket_recv(void *handle, void *data, unsigned size) 00211 { 00212 struct EMW3162_socket *socket = (struct EMW3162_socket *)handle; 00213 _esp.setTimeout(EMW3162_RECV_TIMEOUT); 00214 00215 int32_t recv = _esp.recv(socket->socketId, data, size); 00216 if (recv < 0) { 00217 return NSAPI_ERROR_WOULD_BLOCK; 00218 } 00219 00220 return recv; 00221 } 00222 00223 int EMW3162Interface::socket_sendto(void *handle, const SocketAddress &addr, const void *data, unsigned size) 00224 { 00225 struct EMW3162_socket *socket = (struct EMW3162_socket *)handle; 00226 if (!socket->connected) { 00227 int err = socket_connect(socket, addr); 00228 if (err < 0) { 00229 return err; 00230 } 00231 } 00232 00233 return socket_send(socket, data, size); 00234 } 00235 00236 int EMW3162Interface::socket_recvfrom(void *handle, SocketAddress *addr, void *data, unsigned size) 00237 { 00238 struct EMW3162_socket *socket = (struct EMW3162_socket *)handle; 00239 return socket_recv(socket, data, size); 00240 } 00241 00242 void EMW3162Interface::socket_attach(void *handle, void (*callback)(void *), void *data) 00243 { 00244 struct EMW3162_socket *socket = (struct EMW3162_socket *)handle; 00245 _cbs[socket->id].callback = callback; 00246 _cbs[socket->id].data = data; 00247 } 00248 00249 void EMW3162Interface::event() { 00250 for (int i = 0; i < EMW3162_SOCKET_COUNT; i++) { 00251 if (_cbs[i].callback) { 00252 _cbs[i].callback(_cbs[i].data); 00253 } 00254 } 00255 }
Generated on Tue Jul 12 2022 21:41:13 by
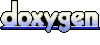