Simple interface for Mbed Cloud Client
Embed:
(wiki syntax)
Show/hide line numbers
sn_nsdl_lib.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2011-2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 /** 00018 * \file sn_nsdl_lib.h 00019 * 00020 * \brief NanoService Devices Library header file 00021 * 00022 * 00023 */ 00024 00025 #ifndef SN_NSDL_LIB_H_ 00026 #define SN_NSDL_LIB_H_ 00027 00028 #include "ns_list.h" 00029 #include "sn_client_config.h" 00030 00031 #ifdef __cplusplus 00032 extern "C" { 00033 #endif 00034 00035 #define SN_NSDL_ENDPOINT_NOT_REGISTERED 0 00036 #define SN_NSDL_ENDPOINT_IS_REGISTERED 1 00037 00038 #define MAX_TOKEN_SIZE 8 00039 #define MAX_URI_QUERY_LEN 255 00040 00041 #ifdef YOTTA_CFG_DISABLE_INTERFACE_DESCRIPTION 00042 #define DISABLE_INTERFACE_DESCRIPTION YOTTA_CFG_DISABLE_INTERFACE_DESCRIPTION 00043 #elif defined MBED_CONF_MBED_CLIENT_DISABLE_INTERFACE_DESCRIPTION 00044 #define DISABLE_INTERFACE_DESCRIPTION MBED_CONF_MBED_CLIENT_DISABLE_INTERFACE_DESCRIPTION 00045 #endif 00046 00047 #ifdef YOTTA_CFG_DISABLE_RESOURCE_TYPE 00048 #define DISABLE_RESOURCE_TYPE YOTTA_CFG_DISABLE_RESOURCE_TYPE 00049 #elif defined MBED_CONF_MBED_CLIENT_DISABLE_RESOURCE_TYPE 00050 #define DISABLE_RESOURCE_TYPE MBED_CONF_MBED_CLIENT_DISABLE_RESOURCE_TYPE 00051 #endif 00052 00053 /* Handle structure */ 00054 struct nsdl_s; 00055 00056 /** 00057 * \brief Received device server security 00058 */ 00059 typedef enum omalw_server_security_ { 00060 SEC_NOT_SET = -1, 00061 PSK = 0, 00062 RPK = 1, 00063 CERTIFICATE = 2, 00064 NO_SEC = 3 00065 } omalw_server_security_t; 00066 00067 /** 00068 * \brief Endpoint binding and mode 00069 */ 00070 typedef enum sn_nsdl_oma_binding_and_mode_ { 00071 BINDING_MODE_NOT_SET = 0, 00072 BINDING_MODE_U = 0x01, 00073 BINDING_MODE_Q = 0x02, 00074 BINDING_MODE_S = 0x04 00075 } sn_nsdl_oma_binding_and_mode_t; 00076 00077 //#define RESOURCE_ATTRIBUTES_LIST 00078 #ifdef RESOURCE_ATTRIBUTES_LIST 00079 /* 00080 * \brief Resource attributes types 00081 */ 00082 typedef enum sn_nsdl_resource_attribute_ { 00083 ATTR_RESOURCE_TYPE, 00084 ATTR_INTERFACE_DESCRIPTION, 00085 ATTR_ENDPOINT_NAME, 00086 ATTR_QUEUE_MODE, 00087 ATTR_LIFETIME, 00088 ATTR_NOP, 00089 ATTR_END 00090 } sn_nsdl_resource_attribute_t; 00091 00092 typedef struct sn_nsdl_attribute_item_ { 00093 sn_nsdl_resource_attribute_t attribute_name; 00094 char *value; 00095 } sn_nsdl_attribute_item_s; 00096 00097 #endif 00098 00099 /** 00100 * \brief Endpoint registration mode. 00101 * If REGISTER_WITH_RESOURCES, endpoint sends list of all resources during registration. 00102 * If REGISTER_WITH_TEMPLATE, endpoint sends registration without resource list. Device server must have 00103 * correctly configured template. 00104 */ 00105 typedef enum sn_nsdl_registration_mode_ { 00106 REGISTER_WITH_RESOURCES = 0, 00107 REGISTER_WITH_TEMPLATE 00108 } sn_nsdl_registration_mode_t; 00109 00110 /** 00111 * \brief Endpoint registration parameters 00112 */ 00113 typedef struct sn_nsdl_ep_parameters_ { 00114 uint8_t endpoint_name_len; 00115 uint8_t domain_name_len; 00116 uint8_t type_len; 00117 uint8_t lifetime_len; 00118 uint8_t location_len; 00119 00120 sn_nsdl_registration_mode_t ds_register_mode; /**< Defines registration mode */ 00121 sn_nsdl_oma_binding_and_mode_t binding_and_mode; /**< Defines endpoints binding and mode */ 00122 00123 uint8_t *endpoint_name_ptr; /**< Endpoint name */ 00124 uint8_t *domain_name_ptr; /**< Domain to register. If null, NSP uses default domain */ 00125 uint8_t *type_ptr; /**< Endpoint type */ 00126 uint8_t *lifetime_ptr; /**< Endpoint lifetime in seconds. eg. "1200" = 1200 seconds */ 00127 uint8_t *location_ptr; /**< Endpoint location in server, optional parameter,default is NULL */ 00128 } sn_nsdl_ep_parameters_s; 00129 00130 /** 00131 * \brief For internal use 00132 */ 00133 typedef struct sn_nsdl_sent_messages_ { 00134 uint8_t message_type; 00135 uint16_t msg_id_number; 00136 ns_list_link_t link; 00137 } sn_nsdl_sent_messages_s; 00138 00139 /** 00140 * \brief Includes resource path 00141 */ 00142 typedef struct sn_grs_resource_ { 00143 char *path; 00144 } sn_grs_resource_s; 00145 00146 /** 00147 * \brief Table of created resources 00148 */ 00149 typedef struct sn_grs_resource_list_ { 00150 uint8_t res_count; /**< Number of resources */ 00151 sn_grs_resource_s *res; 00152 } sn_grs_resource_list_s; 00153 00154 /** 00155 * \brief Resource access rights 00156 */ 00157 typedef enum sn_grs_resource_acl_ { 00158 SN_GRS_GET_ALLOWED = 0x01 , 00159 SN_GRS_PUT_ALLOWED = 0x02, 00160 SN_GRS_POST_ALLOWED = 0x04, 00161 SN_GRS_DELETE_ALLOWED = 0x08 00162 } sn_grs_resource_acl_e; 00163 00164 /** 00165 * \brief Defines the resource mode 00166 */ 00167 typedef enum sn_nsdl_resource_mode_ { 00168 SN_GRS_STATIC = 0, /**< Static resources have some value that doesn't change */ 00169 SN_GRS_DYNAMIC, /**< Dynamic resources are handled in application. Therefore one must give function callback pointer to them */ 00170 SN_GRS_DIRECTORY /**< Directory resources are unused and unsupported */ 00171 } sn_nsdl_resource_mode_e; 00172 00173 /** 00174 * Enum defining an status codes that can happen when 00175 * sending notification 00176 */ 00177 typedef enum { 00178 NOTIFICATION_STATUS_INIT = 0, // Initial state. 00179 NOTIFICATION_STATUS_BUILD_ERROR, // CoAP message building fails. 00180 NOTIFICATION_STATUS_RESEND_QUEUE_FULL, // CoAP resend queue full. 00181 NOTIFICATION_STATUS_SENT, // Notification sent to the server but ACK not yet received. 00182 NOTIFICATION_STATUS_DELIVERED, // Received ACK from server. 00183 NOTIFICATION_STATUS_SEND_FAILED, // Message sending failed (retransmission completed). 00184 NOTIFICATION_STATUS_SUBSCRIBED, // Server has started the observation 00185 NOTIFICATION_STATUS_UNSUBSCRIBED // Server has stopped the observation (RESET message or GET with observe 1) 00186 } NotificationDeliveryStatus; 00187 00188 /** Dummy alias to maintain compatibility with older version which had a typo in the enum name. */ 00189 typedef NotificationDeliveryStatus NoticationDeliveryStatus; 00190 00191 00192 /** 00193 * \brief Defines static parameters for the resource. 00194 */ 00195 typedef struct sn_nsdl_static_resource_parameters_ { 00196 #ifndef RESOURCE_ATTRIBUTES_LIST 00197 #ifndef DISABLE_RESOURCE_TYPE 00198 char *resource_type_ptr; /**< Type of the resource */ 00199 #endif 00200 #ifndef DISABLE_INTERFACE_DESCRIPTION 00201 char *interface_description_ptr; /**< Interface description */ 00202 #endif 00203 #else 00204 sn_nsdl_attribute_item_s *attributes_ptr; 00205 #endif 00206 char *path; /**< Resource path */ 00207 bool external_memory_block:1; /**< 0 means block messages are handled inside this library, 00208 otherwise block messages are passed to application */ 00209 unsigned mode:2; /**< STATIC etc.. */ 00210 bool free_on_delete:1; /**< 1 if struct is dynamic allocted --> to be freed */ 00211 } sn_nsdl_static_resource_parameters_s; 00212 00213 /** 00214 * \brief Defines dynamic parameters for the resource. 00215 */ 00216 typedef struct sn_nsdl_resource_parameters_ { 00217 uint8_t (*sn_grs_dyn_res_callback)(struct nsdl_s *, 00218 sn_coap_hdr_s *, 00219 sn_nsdl_addr_s *, 00220 sn_nsdl_capab_e); 00221 #ifdef MEMORY_OPTIMIZED_API 00222 const sn_nsdl_static_resource_parameters_s *static_resource_parameters; 00223 #else 00224 sn_nsdl_static_resource_parameters_s *static_resource_parameters; 00225 #endif 00226 uint8_t *resource; /**< NULL if dynamic resource */ 00227 ns_list_link_t link; 00228 uint16_t resourcelen; /**< 0 if dynamic resource, resource information in static resource */ 00229 uint16_t coap_content_type; /**< CoAP content type */ 00230 uint16_t msg_id; /**< Notification message id. */ 00231 unsigned access:4; /**< Allowed operation mode, GET, PUT, etc, 00232 TODO! This should be in static struct but current 00233 mbed-client implementation requires this to be changed at runtime */ 00234 unsigned registered:2; /**< Is resource registered or not */ 00235 bool publish_uri:1; /**< 1 if resource to be published to server */ 00236 bool free_on_delete:1; /**< 1 if struct is dynamic allocted --> to be freed */ 00237 bool observable:1; /**< Is resource observable or not */ 00238 bool auto_observable:1; /**< Is resource auto observable or not */ 00239 NotificationDeliveryStatus notification_status:3; /**< Notification delivery status */ 00240 } sn_nsdl_dynamic_resource_parameters_s; 00241 00242 /** 00243 * \brief Defines OMAlw server information 00244 */ 00245 typedef struct sn_nsdl_oma_server_info_ { 00246 sn_nsdl_addr_s *omalw_address_ptr; 00247 omalw_server_security_t omalw_server_security; 00248 00249 } sn_nsdl_oma_server_info_t; 00250 00251 /** 00252 * \brief Defines endpoint parameters to OMA bootstrap. 00253 */ 00254 typedef struct sn_nsdl_bs_ep_info_ { 00255 void (*oma_bs_status_cb)(sn_nsdl_oma_server_info_t *); /**< Callback for OMA bootstrap status */ 00256 00257 void (*oma_bs_status_cb_handle)(sn_nsdl_oma_server_info_t *, 00258 struct nsdl_s *); /**< Callback for OMA bootstrap status with nsdl handle */ 00259 } sn_nsdl_bs_ep_info_t; 00260 00261 /** 00262 * \fn struct nsdl_s *sn_nsdl_init (uint8_t (*sn_nsdl_tx_cb)(sn_nsdl_capab_e , uint8_t *, uint16_t, sn_nsdl_addr_s *), 00263 * uint8_t (*sn_nsdl_rx_cb)(sn_coap_hdr_s *, sn_nsdl_addr_s *), 00264 * sn_nsdl_mem_s *sn_memory) 00265 * 00266 * \brief Initialization function for NSDL library. Initializes NSDL, GRS, HTTP and CoAP. 00267 * 00268 * \param *sn_nsdl_tx_callback A callback function for sending messages. 00269 * 00270 * \param *sn_nsdl_rx_callback A callback function for parsed messages. If received message is not CoAP protocol message (eg. ACK), message for GRS (GET, PUT, POST, DELETE) or 00271 * reply for some DS messages (register message etc.), rx callback will be called. 00272 * 00273 * \param *sn_memory Memory structure which includes function pointers to the allocation and free functions. 00274 * 00275 * \return pointer to created handle structure. NULL if failed 00276 */ 00277 struct nsdl_s *sn_nsdl_init(uint8_t (*sn_nsdl_tx_cb)(struct nsdl_s *, sn_nsdl_capab_e , uint8_t *, uint16_t, sn_nsdl_addr_s *), 00278 uint8_t (*sn_nsdl_rx_cb)(struct nsdl_s *, sn_coap_hdr_s *, sn_nsdl_addr_s *), 00279 void *(*sn_nsdl_alloc)(uint16_t), void (*sn_nsdl_free)(void *), 00280 uint8_t (*sn_nsdl_auto_obs_token_cb)(struct nsdl_s *, const char *, uint8_t *)); 00281 00282 /** 00283 * \fn extern uint16_t sn_nsdl_register_endpoint(struct nsdl_s *handle, sn_nsdl_ep_parameters_s *endpoint_info_ptr, const char *uri_query_parameters); 00284 * 00285 * \brief Registers endpoint to mbed Device Server. 00286 * \param *handle Pointer to nsdl-library handle 00287 * \param *endpoint_info_ptr Contains endpoint information. 00288 * \param *uri_query_parameters Uri query parameters. 00289 * 00290 * \return registration message ID, 0 if failed 00291 */ 00292 extern uint16_t sn_nsdl_register_endpoint(struct nsdl_s *handle, 00293 sn_nsdl_ep_parameters_s *endpoint_info_ptr, 00294 const char *uri_query_parameters); 00295 00296 /** 00297 * \fn extern int32_t sn_nsdl_unregister_endpoint(struct nsdl_s *handle) 00298 * 00299 * \brief Sends unregister-message to mbed Device Server. 00300 * 00301 * \param *handle Pointer to nsdl-library handle 00302 * 00303 * \return unregistration message ID, 0 if failed 00304 */ 00305 extern int32_t sn_nsdl_unregister_endpoint(struct nsdl_s *handle); 00306 00307 /** 00308 * \fn extern int32_t sn_nsdl_update_registration(struct nsdl_s *handle, uint8_t *lt_ptr, uint8_t lt_len); 00309 * 00310 * \brief Update the registration with mbed Device Server. 00311 * 00312 * \param *handle Pointer to nsdl-library handle 00313 * \param *lt_ptr Pointer to lifetime value string in ascii form, eg. "1200" 00314 * \param lt_len Length of the lifetime string 00315 * 00316 * \return registration update message ID, <0 if failed 00317 */ 00318 extern int32_t sn_nsdl_update_registration(struct nsdl_s *handle, uint8_t *lt_ptr, uint8_t lt_len); 00319 00320 /** 00321 * \fn extern int8_t sn_nsdl_set_endpoint_location(struct nsdl_s *handle, uint8_t *location_ptr, uint8_t location_len); 00322 * 00323 * \brief Sets the location receievd from Device Server. 00324 * 00325 * \param *handle Pointer to nsdl-library handle 00326 * \param *lt_ptr Pointer to location value string , eg. "s322j4k" 00327 * \param lt_len Length of the location string 00328 * 00329 * \return success, <0 if failed 00330 */ 00331 extern int8_t sn_nsdl_set_endpoint_location(struct nsdl_s *handle, uint8_t *location_ptr, uint8_t location_len); 00332 00333 /** 00334 * \fn extern int8_t sn_nsdl_is_ep_registered(struct nsdl_s *handle) 00335 * 00336 * \brief Checks if endpoint is registered. 00337 * 00338 * \param *handle Pointer to nsdl-library handle 00339 * 00340 * \return 1 Endpoint registration is done successfully 00341 * \return 0 Endpoint is not registered 00342 */ 00343 extern int8_t sn_nsdl_is_ep_registered(struct nsdl_s *handle); 00344 00345 /** 00346 * \fn extern void sn_nsdl_nsp_lost(struct nsdl_s *handle); 00347 * 00348 * \brief A function to inform mbed Device C client library if application detects a fault in mbed Device Server registration. 00349 * 00350 * \param *handle Pointer to nsdl-library handle 00351 * 00352 * After calling this function sn_nsdl_is_ep_registered() will return "not registered". 00353 */ 00354 extern void sn_nsdl_nsp_lost(struct nsdl_s *handle); 00355 00356 /** 00357 * \fn extern uint16_t sn_nsdl_send_observation_notification(struct nsdl_s *handle, uint8_t *token_ptr, uint8_t token_len, 00358 * uint8_t *payload_ptr, uint16_t payload_len, 00359 * sn_coap_observe_e observe, 00360 * sn_coap_msg_type_e message_type, sn_coap_content_format_e content_format) 00361 * 00362 * 00363 * \brief Sends observation message to mbed Device Server 00364 * 00365 * \param *handle Pointer to nsdl-library handle 00366 * \param *token_ptr Pointer to token to be used 00367 * \param token_len Token length 00368 * \param *payload_ptr Pointer to payload to be sent 00369 * \param payload_len Payload length 00370 * \param observe Observe option value to be sent 00371 * \param message_type Observation message type (confirmable or non-confirmable) 00372 * \param content_format Observation message payload content format 00373 * \param message_id -1 means stored value to be used otherwise new one is generated 00374 * 00375 * \return >0 Success, observation messages message ID 00376 * \return <=0 Failure 00377 */ 00378 extern int32_t sn_nsdl_send_observation_notification(struct nsdl_s *handle, uint8_t *token_ptr, uint8_t token_len, 00379 uint8_t *payload_ptr, uint16_t payload_len, 00380 sn_coap_observe_e observe, 00381 sn_coap_msg_type_e message_type, 00382 sn_coap_content_format_e content_format, 00383 const int32_t message_id); 00384 00385 /** 00386 * \fn extern uint32_t sn_nsdl_get_version(void) 00387 * 00388 * \brief Version query function. 00389 * 00390 * Used to retrieve the version information from the mbed Device C Client library. 00391 * 00392 * \return Pointer to library version string 00393 */ 00394 extern char *sn_nsdl_get_version(void); 00395 00396 /** 00397 * \fn extern int8_t sn_nsdl_process_coap(struct nsdl_s *handle, uint8_t *packet, uint16_t packet_len, sn_nsdl_addr_s *src) 00398 * 00399 * \brief To push CoAP packet to mbed Device C Client library 00400 * 00401 * Used to push an CoAP packet to mbed Device C Client library for processing. 00402 * 00403 * \param *handle Pointer to nsdl-library handle 00404 * 00405 * \param *packet Pointer to a uint8_t array containing the packet (including the CoAP headers). 00406 * After successful execution this array may contain the response packet. 00407 * 00408 * \param *packet_len Pointer to length of the packet. After successful execution this array may contain the length 00409 * of the response packet. 00410 * 00411 * \param *src Pointer to packet source address information. After successful execution this array may contain 00412 * the destination address of the response packet. 00413 * 00414 * \return 0 Success 00415 * \return -1 Failure 00416 */ 00417 extern int8_t sn_nsdl_process_coap(struct nsdl_s *handle, uint8_t *packet, uint16_t packet_len, sn_nsdl_addr_s *src); 00418 00419 /** 00420 * \fn extern int8_t sn_nsdl_exec(struct nsdl_s *handle, uint32_t time); 00421 * 00422 * \brief CoAP retransmission function. 00423 * 00424 * Used to give execution time for the mbed Device C Client library for retransmissions. 00425 * 00426 * \param *handle Pointer to nsdl-library handle 00427 * 00428 * \param time Time in seconds. 00429 * 00430 * \return 0 Success 00431 * \return -1 Failure 00432 */ 00433 extern int8_t sn_nsdl_exec(struct nsdl_s *handle, uint32_t time); 00434 00435 /** 00436 * \fn extern int8_t sn_nsdl_put_resource(struct nsdl_s *handle, const sn_nsdl_dynamic_resource_parameters_s *res); 00437 * 00438 * \brief Resource putting function. 00439 * 00440 * Used to put a static or dynamic CoAP resource without creating copy of it. 00441 * NOTE: Remember that only resource will be owned, not data that it contains 00442 * NOTE: The resource may be removed from list by sn_nsdl_pop_resource(). 00443 * 00444 * \param *res Pointer to a structure of type sn_nsdl_dynamic_resource_parameters_s that contains the information 00445 * about the resource. 00446 * 00447 * \return 0 Success 00448 * \return -1 Failure 00449 * \return -2 Resource already exists 00450 * \return -3 Invalid path 00451 * \return -4 List adding failure 00452 */ 00453 extern int8_t sn_nsdl_put_resource(struct nsdl_s *handle, sn_nsdl_dynamic_resource_parameters_s *res); 00454 00455 /** 00456 * \fn extern int8_t sn_nsdl_pop_resource(struct nsdl_s *handle, const sn_nsdl_dynamic_resource_parameters_s *res); 00457 * 00458 * \brief Resource popping function. 00459 * 00460 * Used to remove a static or dynamic CoAP resource from lists without deleting it. 00461 * NOTE: This function is a counterpart of sn_nsdl_put_resource(). 00462 * 00463 * \param *res Pointer to a structure of type sn_nsdl_dynamic_resource_parameters_s that contains the information 00464 * about the resource. 00465 * 00466 * \return 0 Success 00467 * \return -1 Failure 00468 * \return -3 Invalid path 00469 */ 00470 extern int8_t sn_nsdl_pop_resource(struct nsdl_s *handle, sn_nsdl_dynamic_resource_parameters_s *res); 00471 00472 /** 00473 * \fn extern int8_t sn_nsdl_delete_resource(struct nsdl_s *handle, char *path) 00474 * 00475 * \brief Resource delete function. 00476 * 00477 * Used to delete a resource. If resource has a subresources, these all must also be removed. 00478 * 00479 * \param *handle Pointer to nsdl-library handle 00480 * \param *path_ptr A pointer to an array containing the path. 00481 * 00482 * \return 0 Success 00483 * \return -1 Failure (No such resource) 00484 */ 00485 extern int8_t sn_nsdl_delete_resource(struct nsdl_s *handle, const char *path); 00486 00487 /** 00488 * \fn extern sn_nsdl_dynamic_resource_parameters_s *sn_nsdl_get_resource(struct nsdl_s *handle, char *path) 00489 * 00490 * \brief Resource get function. 00491 * 00492 * Used to get a resource. 00493 * 00494 * \param *handle Pointer to nsdl-library handle 00495 * \param *path A pointer to an array containing the path. 00496 * 00497 * \return !NULL Success, pointer to a sn_nsdl_dynamic_resource_parameters_s that contains the resource information\n 00498 * \return NULL Failure 00499 */ 00500 extern sn_nsdl_dynamic_resource_parameters_s *sn_nsdl_get_resource(struct nsdl_s *handle, const char *path); 00501 00502 /** 00503 * \fn extern sn_grs_resource_list_s *sn_nsdl_list_resource(struct nsdl_s *handle, char *path) 00504 * 00505 * \brief Resource list function. 00506 * 00507 * \param *handle Pointer to nsdl-library handle 00508 * \param *path A pointer to an array containing the path or a NULL pointer. 00509 * 00510 * \return !NULL A pointer to a sn_grs_resource_list_s structure containing the resource listing. 00511 * \return NULL Failure with an unspecified error 00512 */ 00513 sn_grs_resource_list_s *sn_nsdl_list_resource(struct nsdl_s *handle, const char *path); 00514 00515 /** 00516 * \fn extern void sn_nsdl_free_resource_list(struct nsdl_s *handle, sn_grs_resource_list_s *list) 00517 * 00518 * \brief Free a resource list obtained from sn_nsdl_list_resource() 00519 * 00520 * \param list The list to free, or NULL. 00521 */ 00522 void sn_nsdl_free_resource_list(struct nsdl_s *handle, sn_grs_resource_list_s *list); 00523 00524 /** 00525 * \fn extern int8_t sn_nsdl_send_coap_message(struct nsdl_s *handle, sn_nsdl_addr_s *address_ptr, sn_coap_hdr_s *coap_hdr_ptr); 00526 * 00527 * \brief Send an outgoing CoAP request. 00528 * 00529 * \param *handle Pointer to nsdl-library handle 00530 * \param *address_ptr Pointer to source address struct 00531 * \param *coap_hdr_ptr Pointer to CoAP message to be sent 00532 * 00533 * \return 0 Success 00534 * \return -1 Failure 00535 */ 00536 extern int8_t sn_nsdl_send_coap_message(struct nsdl_s *handle, sn_nsdl_addr_s *address_ptr, sn_coap_hdr_s *coap_hdr_ptr); 00537 00538 /** 00539 * \fn extern int32_t sn_nsdl_send_get_data_request(struct nsdl_s *handle); 00540 * 00541 * \brief Send an outgoing CoAP GET request. 00542 * 00543 * \param *handle Pointer to nsdl-library handle 00544 * \param *uri_path Path to the data 00545 * \param *token Message token 00546 * 00547 * \return 0 Success 00548 * \return -1 Failure 00549 */ 00550 extern int32_t sn_nsdl_send_get_data_request(struct nsdl_s *handle, 00551 const char *uri_path, 00552 const uint32_t token, 00553 const size_t offset); 00554 00555 /** 00556 * \fn extern int8_t set_NSP_address(struct nsdl_s *handle, uint8_t *NSP_address, uint8_t address_length, uint16_t port, sn_nsdl_addr_type_e address_type); 00557 * 00558 * \brief This function is used to set the mbed Device Server address given by an application. 00559 * 00560 * \param *handle Pointer to nsdl-library handle 00561 * \return 0 Success 00562 * \return -1 Failed to indicate that internal address pointer is not allocated (call nsdl_init() first). 00563 */ 00564 extern int8_t set_NSP_address(struct nsdl_s *handle, uint8_t *NSP_address, uint8_t address_length, uint16_t port, sn_nsdl_addr_type_e address_type); 00565 00566 /** 00567 * \fn extern int8_t sn_nsdl_destroy(struct nsdl_s *handle); 00568 * 00569 * \param *handle Pointer to nsdl-library handle 00570 * \brief This function releases all allocated memory in mbed Device C Client library. 00571 */ 00572 extern int8_t sn_nsdl_destroy(struct nsdl_s *handle); 00573 00574 /** 00575 * \fn extern uint16_t sn_nsdl_oma_bootstrap(struct nsdl_s *handle, sn_nsdl_addr_s *bootstrap_address_ptr, sn_nsdl_ep_parameters_s *endpoint_info_ptr, sn_nsdl_bs_ep_info_t *bootstrap_endpoint_info_ptr); 00576 * 00577 * \brief Starts OMA bootstrap process 00578 * 00579 * \param *handle Pointer to nsdl-library handle 00580 * 00581 * \return bootstrap message ID, 0 if failed 00582 */ 00583 extern uint16_t sn_nsdl_oma_bootstrap(struct nsdl_s *handle, 00584 sn_nsdl_addr_s *bootstrap_address_ptr, 00585 sn_nsdl_ep_parameters_s *endpoint_info_ptr, 00586 sn_nsdl_bs_ep_info_t *bootstrap_endpoint_info_ptr, 00587 const char *uri_query_parameters); 00588 00589 /** 00590 * \fn sn_coap_hdr_s *sn_nsdl_build_response(struct nsdl_s *handle, sn_coap_hdr_s *coap_packet_ptr, uint8_t msg_code) 00591 * 00592 * \brief Prepares generic response packet from a request packet. This function allocates memory for the resulting sn_coap_hdr_s 00593 * 00594 * \param *handle Pointer to library handle 00595 * \param *coap_packet_ptr The request packet pointer 00596 * \param msg_code response messages code 00597 * 00598 * \return *coap_packet_ptr The allocated and pre-filled response packet pointer 00599 * NULL Error in parsing the request 00600 * 00601 */ 00602 extern sn_coap_hdr_s *sn_nsdl_build_response(struct nsdl_s *handle, sn_coap_hdr_s *coap_packet_ptr, uint8_t msg_code); 00603 00604 /** 00605 * \brief Allocates and initializes options list structure 00606 * 00607 * \param *handle Pointer to library handle 00608 * \param *coap_msg_ptr is pointer to CoAP message that will contain the options 00609 * 00610 * If the message already has a pointer to an option structure, that pointer 00611 * is returned, rather than a new structure being allocated. 00612 * 00613 * \return Return value is pointer to the CoAP options structure.\n 00614 * In following failure cases NULL is returned:\n 00615 * -Failure in given pointer (= NULL)\n 00616 * -Failure in memory allocation (malloc() returns NULL) 00617 */ 00618 extern sn_coap_options_list_s *sn_nsdl_alloc_options_list(struct nsdl_s *handle, sn_coap_hdr_s *coap_msg_ptr); 00619 00620 /** 00621 * \fn void sn_nsdl_release_allocated_coap_msg_mem(struct nsdl_s *handle, sn_coap_hdr_s *freed_coap_msg_ptr) 00622 * 00623 * \brief Releases memory of given CoAP message 00624 * 00625 * Note!!! Does not release Payload part 00626 * 00627 * \param *handle Pointer to library handle 00628 * 00629 * \param *freed_coap_msg_ptr is pointer to released CoAP message 00630 */ 00631 extern void sn_nsdl_release_allocated_coap_msg_mem(struct nsdl_s *handle, sn_coap_hdr_s *freed_coap_msg_ptr); 00632 00633 /** 00634 * \fn int8_t sn_nsdl_set_retransmission_parameters(struct nsdl_s *handle, uint8_t resending_count, uint8_t resending_intervall) 00635 * 00636 * \brief If re-transmissions are enabled, this function changes resending count and interval. 00637 * 00638 * \param *handle Pointer to library handle 00639 * \param uint8_t resending_count max number of resendings for message 00640 * \param uint8_t resending_intervall message resending intervall in seconds 00641 * \return 0 = success, -1 = failure 00642 */ 00643 extern int8_t sn_nsdl_set_retransmission_parameters(struct nsdl_s *handle, uint8_t resending_count, uint8_t resending_interval); 00644 00645 /** 00646 * \fn int8_t sn_nsdl_set_retransmission_buffer(struct nsdl_s *handle, uint8_t buffer_size_messages, uint16_t buffer_size_bytes) 00647 * 00648 * \brief If re-transmissions are enabled, this function changes message retransmission queue size. 00649 * Set size to '0' to disable feature. If both are set to '0', then re-sendings are disabled. 00650 * 00651 * \param *handle Pointer to library handle 00652 * \param uint8_t buffer_size_messages queue size - maximum number of messages to be saved to queue 00653 * \param uint8_t buffer_size_bytes queue size - maximum size of messages saved to queue 00654 * \return 0 = success, -1 = failure 00655 */ 00656 extern int8_t sn_nsdl_set_retransmission_buffer(struct nsdl_s *handle, 00657 uint8_t buffer_size_messages, uint16_t buffer_size_bytes); 00658 00659 /** 00660 * \fn int8_t sn_nsdl_set_block_size(struct nsdl_s *handle, uint16_t block_size) 00661 * 00662 * \brief If block transfer is enabled, this function changes the block size. 00663 * 00664 * \param *handle Pointer to library handle 00665 * \param uint16_t block_size maximum size of CoAP payload. Valid sizes are 16, 32, 64, 128, 256, 512 and 1024 bytes 00666 * \return 0 = success, -1 = failure 00667 */ 00668 extern int8_t sn_nsdl_set_block_size(struct nsdl_s *handle, uint16_t block_size); 00669 00670 /** 00671 * \fn int8_t sn_nsdl_set_duplicate_buffer_size(struct nsdl_s *handle,uint8_t message_count) 00672 * 00673 * \brief If dublicate message detection is enabled, this function changes buffer size. 00674 * 00675 * \param *handle Pointer to library handle 00676 * \param uint8_t message_count max number of messages saved for duplicate control 00677 * \return 0 = success, -1 = failure 00678 */ 00679 extern int8_t sn_nsdl_set_duplicate_buffer_size(struct nsdl_s *handle, uint8_t message_count); 00680 00681 /** 00682 * \fn void *sn_nsdl_set_context(const struct nsdl_s *handle, void *context) 00683 * 00684 * \brief Set the application defined context parameter for given handle. 00685 * This is useful for example when interfacing with c++ objects where a 00686 * pointer to object is set as the context, and in the callback functions 00687 * the context pointer can be used to call methods for the correct instance 00688 * of the c++ object. 00689 * 00690 * \param *handle Pointer to library handle 00691 * \param *context Pointer to the application defined context 00692 * \return 0 = success, -1 = failure 00693 */ 00694 extern int8_t sn_nsdl_set_context(struct nsdl_s * const handle, void * const context); 00695 00696 /** 00697 * \fn void *sn_nsdl_get_context(const struct nsdl_s *handle) 00698 * 00699 * \brief Get the application defined context parameter for given handle. 00700 * This is useful for example when interfacing with c++ objects where a 00701 * pointer to object is set as the context, and in the callback functions 00702 * the context pointer can be used to call methods for the correct instance 00703 * of the c++ object. 00704 * 00705 * \param *handle Pointer to library handle 00706 * \return Pointer to the application defined context 00707 */ 00708 extern void *sn_nsdl_get_context(const struct nsdl_s * const handle); 00709 00710 /** 00711 * \fn int8_t sn_nsdl_clear_coap_resending_queue(struct nsdl_s *handle) 00712 * 00713 * \brief Clean confirmable message list. 00714 * 00715 * \param *handle Pointer to library handle 00716 * \return 0 = success, -1 = failure 00717 */ 00718 extern int8_t sn_nsdl_clear_coap_resending_queue(struct nsdl_s *handle); 00719 00720 /** 00721 * \fn int8_t sn_nsdl_clear_coap_sent_blockwise_messages(struct nsdl_s *handle) 00722 * 00723 * \brief Clears the sent blockwise messages from the linked list. 00724 * 00725 * \param *handle Pointer to library handle 00726 * \return 0 = success, -1 = failure 00727 */ 00728 extern int8_t sn_nsdl_clear_coap_sent_blockwise_messages(struct nsdl_s *handle); 00729 00730 /** 00731 * \fn int8_t sn_nsdl_handle_block2_response_internally(struct nsdl_s *handle, uint8_t handle_response) 00732 * 00733 * \brief This function change the state whether CoAP library sends the block 2 response automatically or not. 00734 * 00735 * \param *handle Pointer to NSDL library handle 00736 * \param handle_response 1 if CoAP library handles the response sending otherwise 0. 00737 * 00738 * \return 0 = success, -1 = failure 00739 */ 00740 extern int8_t sn_nsdl_handle_block2_response_internally(struct nsdl_s *handle, uint8_t handle_response); 00741 00742 #ifdef RESOURCE_ATTRIBUTES_LIST 00743 /** 00744 * \fn int8_t sn_nsdl_free_resource_attributes_list(struct nsdl_s *handle, sn_nsdl_static_resource_parameters_s *params) 00745 * 00746 * \brief Free resource attributes list if free_on_delete is true for params. This will also free all attributes values 00747 * if they are pointer types. 00748 * 00749 * \param *params Pointer to resource static parameters 00750 */ 00751 extern void sn_nsdl_free_resource_attributes_list(sn_nsdl_static_resource_parameters_s *params); 00752 00753 /* 00754 * \fn bool sn_nsdl_set_resource_attribute(sn_nsdl_static_resource_parameters_s *params, sn_nsdl_attribute_item_s attribute) 00755 * 00756 * \brief Set resource link-format attribute value, create if it doesn't exist yet. 00757 * 00758 * \param *params Pointer to resource static parameters 00759 * \param attribute sn_nsdl_attribute_item_s structure containing attribute to set 00760 * \return True if successful, false on error 00761 */ 00762 extern bool sn_nsdl_set_resource_attribute(sn_nsdl_static_resource_parameters_s *params, const sn_nsdl_attribute_item_s *attribute); 00763 00764 /* 00765 * \fn bool sn_nsdl_get_resource_attribute(sn_nsdl_static_resource_parameters_s *params, sn_nsdl_resource_attribute_t attribute) 00766 * 00767 * \brief Get resource link-format attribute value 00768 * 00769 * \param *params Pointer to resource static parameters 00770 * \param attribute sn_nsdl_resource_attribute_t enum value for attribute to get 00771 * \return Pointer to value or null if attribute did not exist or had no value 00772 */ 00773 extern const char* sn_nsdl_get_resource_attribute(const sn_nsdl_static_resource_parameters_s *params, sn_nsdl_resource_attribute_t attribute); 00774 00775 /* 00776 * \fn bool sn_nsdl_remove_resource_attribute(sn_nsdl_static_resource_parameters_s *params, sn_nsdl_resource_attribute_t attribute) 00777 * 00778 * \brief Remove resource link-format attribute value 00779 * 00780 * \param *params Pointer to resource static parameters 00781 * \param attribute sn_nsdl_resource_attribute_t enum value for attribute to remove 00782 */ 00783 extern bool sn_nsdl_remove_resource_attribute(sn_nsdl_static_resource_parameters_s *params, sn_nsdl_resource_attribute_t attribute); 00784 #endif 00785 #ifdef __cplusplus 00786 } 00787 #endif 00788 00789 #endif /* SN_NSDL_LIB_H_ */
Generated on Tue Jul 12 2022 19:01:37 by
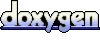