
Describes predefine macros for mbed online compiler (armcc)
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file main.cpp 00004 * @author Toyomasa Watarai 00005 * @brief armcc pre-defined macro check progmra 00006 ****************************************************************************** 00007 * @attention 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); 00010 * you may not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, 00017 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 */ 00021 #include "mbed.h" 00022 00023 #define xstr(s) str(s) 00024 #define str(s) #s 00025 00026 DigitalOut myled(LED1); 00027 00028 int main() { 00029 printf("\n"); 00030 printf("System Clock = %ld\n", SystemCoreClock); 00031 #ifdef MBED_USERNAME 00032 printf("mbed username: %s\n", xstr(MBED_USERNAME)); 00033 #endif 00034 #ifdef MBED_LIBRARY_VERSION 00035 printf("mbed library version: %d\n", MBED_LIBRARY_VERSION); 00036 #endif 00037 #ifdef MBED_CONF_RTOS_PRESENT 00038 printf("Mbed OS %d.%d.%d\n", MBED_MAJOR_VERSION, MBED_MINOR_VERSION, MBED_PATCH_VERSION); 00039 #endif 00040 00041 #ifdef __CC_ARM 00042 #ifdef __MICROLIB 00043 printf("Built with ARM compiler and micro library (uARM)\n"); 00044 #else 00045 printf("Built with ARM compiler and standard library (ARM)\n"); 00046 #endif 00047 printf("ARM Compiler version : %d\n", __ARMCC_VERSION); 00048 00049 #ifdef __ARM_NEON__ 00050 printf("NEON is available\n"); 00051 #else 00052 printf("NEON is not available\n"); 00053 #endif 00054 00055 #ifdef __APCS_INTERWORK 00056 printf("ARM/Thumb Interworking is used\n"); 00057 #endif 00058 00059 #ifdef __APCS_ROPI 00060 printf("--apcs /ropi is used\n"); 00061 #endif 00062 00063 #ifdef __APCS_RWPI 00064 printf("--apcs /rwpi is used\n"); 00065 #endif 00066 00067 #ifdef __APCS_FPIC 00068 printf("--apcs /fpic is used\n"); 00069 #endif 00070 00071 #ifdef __BIG_ENDIAN 00072 printf("Target is big endian\n"); 00073 #else 00074 printf("Target is little endian\n"); 00075 #endif 00076 00077 #ifdef __CHAR_UNSIGNED__ 00078 printf("char type is unsigned\n"); 00079 #else 00080 printf("char type is signed\n"); 00081 #endif 00082 printf("EDG front-end version : %d\n", __EDG_VERSION__); 00083 00084 printf("Emulated GNU version : %d.%d\n", __GNUC__, __GNUC_MINOR__); 00085 printf("Current emulated GNU version : %s\n", __VERSION__); 00086 00087 printf("Optimize level : %d\n", __OPTIMISE_LEVEL); 00088 00089 #ifdef __OPTIMISE_SPACE 00090 printf("Optimized by size\n"); 00091 #endif 00092 00093 #ifdef __OPTIMISE_TIME 00094 printf("Optimized by speed\n"); 00095 #endif 00096 00097 printf("Target ARM architecture : %d\n", __TARGET_ARCH_ARM); 00098 printf("Target Thumb architecture : %d\n", __TARGET_ARCH_THUMB); 00099 00100 #elif defined (__GNUC__) 00101 #if defined (__ARMCC_VERSION) 00102 printf("Built with ARM compiler 6\n"); 00103 printf("Arm compiler version %d\n", __ARMCC_VERSION); 00104 #else 00105 printf("Built with GNU compiler\n"); 00106 #endif 00107 printf("Compatible GCC version %d.%d, %s\n", __GNUC__, __GNUC_MINOR__, __VERSION__); 00108 printf("Target ARM architecture : %d-%c\n", __ARM_ARCH, __ARM_ARCH_PROFILE); 00109 #else 00110 printf("Not build with ARM compiler\n"); 00111 #endif 00112 00113 printf("Compile date : %s\n", __DATE__); 00114 printf("Compile time : %s\n", __TIME__); 00115 00116 00117 while(1) { 00118 myled = !myled; 00119 #if (MBED_MAJOR_VERSION >= 5) && (MBED_MINOR_VERSION >= 15) 00120 thread_sleep_for(400); 00121 #else 00122 wait(0.4); 00123 #endif 00124 } 00125 }
Generated on Wed Jul 13 2022 00:27:22 by
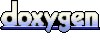