
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
update_client_hub.c
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // SPDX-License-Identifier: Apache-2.0 00005 // 00006 // Licensed under the Apache License, Version 2.0 (the "License"); 00007 // you may not use this file except in compliance with the License. 00008 // You may obtain a copy of the License at 00009 // 00010 // http://www.apache.org/licenses/LICENSE-2.0 00011 // 00012 // Unless required by applicable law or agreed to in writing, software 00013 // distributed under the License is distributed on an "AS IS" BASIS, 00014 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 // See the License for the specific language governing permissions and 00016 // limitations under the License. 00017 // ---------------------------------------------------------------------------- 00018 00019 #include "update-client-hub/update_client_hub.h" 00020 00021 #include "update-client-common/arm_uc_common.h" 00022 #include "update-client-control-center/arm_uc_control_center.h" 00023 #include "update-client-control-center/arm_uc_pre_shared_key.h" 00024 #include "update-client-control-center/arm_uc_certificate.h" 00025 #include "update-client-source-manager/arm_uc_source_manager.h" 00026 #include "update-client-firmware-manager/arm_uc_firmware_manager.h" 00027 #include "update-client-manifest-manager/update-client-manifest-manager.h" 00028 00029 #include "update_client_hub_state_machine.h" 00030 #include "update_client_hub_event_handlers.h" 00031 #include "update_client_hub_error_handler.h" 00032 00033 #include "pal4life-device-identity/pal_device_identity.h" 00034 00035 #define HANDLE_INIT_ERROR(retval, msg, ...)\ 00036 if (retval.error != ERR_NONE)\ 00037 {\ 00038 ARM_UC_HUB_setState(ARM_UC_HUB_STATE_UNINITIALIZED);\ 00039 UC_HUB_ERR_MSG(msg " error code %s", ##__VA_ARGS__, ARM_UC_err2Str(retval));\ 00040 return retval;\ 00041 } 00042 00043 static const ARM_UPDATE_SOURCE** arm_uc_sources = NULL; 00044 static uint8_t arm_uc_sources_size = 0; 00045 00046 static arm_uc_mmContext_t manifestManagerInitContext = { 0 }; 00047 static arm_uc_mmContext_t* pManifestManagerInitContext = &manifestManagerInitContext; 00048 00049 /** 00050 * @brief Call initialiser of all components of the client. 00051 * finish asynchronously, will invoke callback when 00052 * initialisation is done. 00053 * @param init_cb the callback to be invoked at the end of initilastion. 00054 */ 00055 arm_uc_error_t ARM_UC_HUB_Initialize(void (*init_cb)(int32_t)) 00056 { 00057 arm_uc_error_t retval; 00058 00059 if (ARM_UC_HUB_getState() != ARM_UC_HUB_STATE_UNINITIALIZED) 00060 { 00061 UC_HUB_ERR_MSG("Already Initialized"); 00062 return (arm_uc_error_t){ ERR_INVALID_PARAMETER }; 00063 } 00064 00065 ARM_UC_HUB_setInitializationCallback(init_cb); 00066 00067 /* Register event handler with Control Center. */ 00068 retval = ARM_UC_ControlCenter_Initialize(ARM_UC_HUB_ControlCenterEventHandler); 00069 HANDLE_INIT_ERROR(retval, "Control Center init failed") 00070 00071 /* Register event handler with Firmware Manager */ 00072 retval = ARM_UC_FirmwareManager.Initialize(ARM_UC_HUB_FirmwareManagerEventHandler); 00073 HANDLE_INIT_ERROR(retval, "Firmware Manager init failed") 00074 00075 /* Register event handler with Source Manager */ 00076 retval = ARM_UC_SourceManager.Initialize(ARM_UC_HUB_SourceManagerEventHandler); 00077 HANDLE_INIT_ERROR(retval, "Source Manager init failed") 00078 00079 for (uint8_t index = 0; index < arm_uc_sources_size; index++) 00080 { 00081 ARM_UC_SourceManager.AddSource(arm_uc_sources[index]); 00082 } 00083 00084 /* Register event handler and add config store implementation to manifest 00085 manager. 00086 */ 00087 retval = ARM_UC_mmInit(&pManifestManagerInitContext, 00088 ARM_UC_HUB_ManifestManagerEventHandler, 00089 NULL); 00090 HANDLE_INIT_ERROR(retval, "Manifest manager init failed") 00091 00092 /* add hard coded certificates to the manifest manager */ 00093 // retval = ARM_UC_mmStoreCertificate(CA_PATH, cert, CERT_SIZE); 00094 // if ((retval.error != ERR_NONE) && (retval.code != MFST_ERR_PENDING)) 00095 // { 00096 // HANDLE_INIT_ERROR(retval, "Manifest manager StoreCertificate failed") 00097 // } 00098 00099 return (arm_uc_error_t){ ERR_NONE }; 00100 } 00101 00102 /** 00103 * @brief Process events in the event queue. 00104 */ 00105 arm_uc_error_t ARM_UC_HUB_ProcessEvents() 00106 { 00107 ARM_UC_ProcessQueue(); 00108 00109 return (arm_uc_error_t){ ERR_NONE }; 00110 } 00111 00112 /** 00113 * @brief Register callback function for when callbacks are added to an empty queue. 00114 */ 00115 arm_uc_error_t ARM_UC_HUB_AddNotificationHandler(void (*handler)(void)) 00116 { 00117 ARM_UC_AddNotificationHandler(handler); 00118 00119 return (arm_uc_error_t){ ERR_NONE }; 00120 } 00121 00122 /** 00123 * @brief Add source to the Update Client. 00124 */ 00125 arm_uc_error_t ARM_UC_HUB_SetSources(const ARM_UPDATE_SOURCE* sources[], 00126 uint8_t size) 00127 { 00128 arm_uc_sources = sources; 00129 arm_uc_sources_size = size; 00130 00131 return (arm_uc_error_t){ ERR_NONE }; 00132 } 00133 00134 /** 00135 * Set PAAL Update implementation 00136 */ 00137 arm_uc_error_t ARM_UC_HUB_SetStorage(const ARM_UC_PAAL_UPDATE* implementation) 00138 { 00139 return ARM_UCP_SetPAALUpdate(implementation); 00140 } 00141 00142 /** 00143 * @brief Add monitor to the control center. 00144 */ 00145 arm_uc_error_t ARM_UC_HUB_AddMonitor(const ARM_UPDATE_MONITOR* monitor) 00146 { 00147 return ARM_UC_ControlCenter_AddMonitor(monitor); 00148 } 00149 00150 /** 00151 * @brief Temporary error reporting function. 00152 */ 00153 void ARM_UC_HUB_AddErrorCallback(void (*callback)(int32_t error)) 00154 { 00155 ARM_UC_HUB_AddErrorCallbackInternal(callback); 00156 } 00157 00158 /** 00159 * @brief Authorize request. 00160 */ 00161 arm_uc_error_t ARM_UC_Authorize(arm_uc_request_t request) 00162 { 00163 return ARM_UC_ControlCenter_Authorize(request); 00164 } 00165 00166 /** 00167 * @brief Set callback for receiving download progress. 00168 * @details User application call for setting callback handler. 00169 * The callback function takes the progreess in percent as argument. 00170 * 00171 * @param callback Function pointer to the progress function. 00172 * @return Error code. 00173 */ 00174 arm_uc_error_t ARM_UC_SetProgressHandler(void (*callback)(uint32_t progress, uint32_t total)) 00175 { 00176 return ARM_UC_ControlCenter_SetProgressHandler(callback); 00177 } 00178 00179 /** 00180 * @brief Set callback function for authorizing requests. 00181 * @details User application call for setting callback handler. 00182 * The callback function takes an enum request and an authorization 00183 * function pointer. To authorize the given request, the caller 00184 * invokes the authorization function. 00185 * 00186 * @param callback Function pointer to the authorization function. 00187 * @return Error code. 00188 */ 00189 arm_uc_error_t ARM_UC_SetAuthorizeHandler(void (*callback)(int32_t)) 00190 { 00191 return ARM_UC_ControlCenter_SetAuthorityHandler(callback); 00192 } 00193 00194 /** 00195 * @brief Override update authorization handler. 00196 * @details Force download and update to progress regardless of authorization 00197 * handler. This function is used for unblocking an update in a buggy 00198 * application. 00199 */ 00200 void ARM_UC_OverrideAuthorization(void) 00201 { 00202 ARM_UC_ControlCenter_OverrideAuthorization(); 00203 } 00204 00205 /** 00206 * @brief Add certificate. 00207 * @details [long description] 00208 * 00209 * @param certificate Pointer to certiface being added. 00210 * @param certificate_length Certificate length. 00211 * @param fingerprint Pointer to the fingerprint of the certificate being added. 00212 * @param fingerprint_length Fingerprint length. 00213 * @return Error code. 00214 */ 00215 arm_uc_error_t ARM_UC_AddCertificate(const uint8_t* certificate, 00216 uint16_t certificate_length, 00217 const uint8_t* fingerprint, 00218 uint16_t fingerprint_length, 00219 void (*callback)(arm_uc_error_t, const arm_uc_buffer_t*)) 00220 { 00221 return ARM_UC_Certificate_Add(certificate, 00222 certificate_length, 00223 fingerprint, 00224 fingerprint_length, 00225 callback); 00226 } 00227 00228 /** 00229 * @brief Set pointer to pre-shared-key with the given size. 00230 * 00231 * @param key Pointer to pre-shared-key. 00232 * @param bits Key size in bits. 00233 * 00234 * @return Error code. 00235 */ 00236 arm_uc_error_t ARM_UC_AddPreSharedKey(const uint8_t* key, uint16_t bits) 00237 { 00238 return ARM_UC_PreSharedKey_SetKey(key, bits); 00239 } 00240 00241 /** 00242 * @brief Function for setting the vendor ID. 00243 * @details The ID is copied to a 16 byte struct. Any data after the first 00244 * 16 bytes will be ignored. 00245 * @param id Pointer to ID. 00246 * @param length Length of ID. 00247 * @return Error code. 00248 */ 00249 arm_uc_error_t ARM_UC_SetVendorId(const uint8_t* id, uint8_t length) 00250 { 00251 arm_uc_guid_t uuid = { 0 }; 00252 00253 if (id) 00254 { 00255 for (uint8_t index = 0; 00256 (index < sizeof(arm_uc_guid_t) && (index < length)); 00257 index++) 00258 { 00259 ((uint8_t*) uuid)[index] = id[index]; 00260 } 00261 } 00262 00263 return pal_setVendorGuid(&uuid); 00264 } 00265 00266 /** 00267 * @brief Function for setting the class ID. 00268 * @details The ID is copied to a 16 byte struct. Any data after the first 00269 * 16 bytes will be ignored. 00270 * @param id Pointer to ID. 00271 * @param length Length of ID. 00272 * @return Error code. 00273 */ 00274 arm_uc_error_t ARM_UC_SetClassId(const uint8_t* id, uint8_t length) 00275 { 00276 arm_uc_guid_t uuid = { 0 }; 00277 00278 if (id) 00279 { 00280 for (uint8_t index = 0; 00281 (index < sizeof(arm_uc_guid_t) && (index < length)); 00282 index++) 00283 { 00284 ((uint8_t*) uuid)[index] = id[index]; 00285 } 00286 } 00287 00288 return pal_setClassGuid(&uuid); 00289 } 00290 00291 /** 00292 * @brief Function for setting the device ID. 00293 * @details The ID is copied to a 16 byte struct. Any data after the first 00294 * 16 bytes will be ignored. 00295 * @param id Pointer to ID. 00296 * @param length Length of ID. 00297 * @return Error code. 00298 */ 00299 arm_uc_error_t ARM_UC_SetDeviceId(const uint8_t* id, uint8_t length) 00300 { 00301 arm_uc_guid_t uuid = { 0 }; 00302 00303 if (id) 00304 { 00305 for (uint8_t index = 0; 00306 (index < sizeof(arm_uc_guid_t) && (index < length)); 00307 index++) 00308 { 00309 ((uint8_t*) uuid)[index] = id[index]; 00310 } 00311 } 00312 00313 return pal_setDeviceGuid(&uuid); 00314 } 00315 00316 /** 00317 * @brief Function for reporting the vendor ID. 00318 * @details 16 bytes are copied into the supplied buffer. 00319 * @param id Pointer to storage for ID. MUST be at least 16 bytes long. 00320 * @param id_max the size of the ID buffer 00321 * @param id_size pointer to a variable to receive the size of the ID 00322 * written into the buffer (always 16). 00323 * @return Error code. 00324 */ 00325 arm_uc_error_t ARM_UC_GetVendorId(uint8_t* id, 00326 const size_t id_max, 00327 size_t* id_size) 00328 { 00329 arm_uc_guid_t guid = {0}; 00330 arm_uc_error_t err = {ERR_NONE}; 00331 if (id_max < sizeof(arm_uc_guid_t)) 00332 { 00333 err.code = ARM_UC_DI_ERR_SIZE; 00334 } 00335 if (err.error == ERR_NONE) 00336 { 00337 err = pal_getVendorGuid(&guid); 00338 } 00339 if (err.error == ERR_NONE) 00340 { 00341 memcpy(id, guid, sizeof(arm_uc_guid_t)); 00342 if (id_size != NULL) 00343 { 00344 *id_size = sizeof(arm_uc_guid_t); 00345 } 00346 } 00347 return err; 00348 } 00349 00350 /** 00351 * @brief Function for reporting the class ID. 00352 * @details 16 bytes are copied into the supplied buffer. 00353 * @param id Pointer to storage for ID. MUST be at least 16 bytes long. 00354 * @param id_max the size of the ID buffer 00355 * @param id_size pointer to a variable to receive the size of the ID 00356 * written into the buffer (always 16). 00357 * @return Error code. 00358 */ 00359 arm_uc_error_t ARM_UC_GetClassId(uint8_t* id, 00360 const size_t id_max, 00361 size_t* id_size) 00362 { 00363 arm_uc_guid_t guid = {0}; 00364 arm_uc_error_t err = {ERR_NONE}; 00365 if (id_max < sizeof(arm_uc_guid_t)) 00366 { 00367 err.code = ARM_UC_DI_ERR_SIZE; 00368 } 00369 if (err.error == ERR_NONE) 00370 { 00371 err = pal_getClassGuid(&guid); 00372 } 00373 if (err.error == ERR_NONE) 00374 { 00375 memcpy(id, guid, sizeof(arm_uc_guid_t)); 00376 if (id_size != NULL) 00377 { 00378 *id_size = sizeof(arm_uc_guid_t); 00379 } 00380 } 00381 return err; 00382 } 00383 00384 /** 00385 * @brief Function for reporting the device ID. 00386 * @details 16 bytes are copied into the supplied buffer. 00387 * @param id Pointer to storage for ID. MUST be at least 16 bytes long. 00388 * @param id_max the size of the ID buffer 00389 * @param id_size pointer to a variable to receive the size of the ID 00390 * written into the buffer (always 16). 00391 * @return Error code. 00392 */ 00393 arm_uc_error_t ARM_UC_GetDeviceId(uint8_t* id, 00394 const size_t id_max, 00395 size_t* id_size) 00396 { 00397 arm_uc_guid_t guid = {0}; 00398 arm_uc_error_t err = {ERR_NONE}; 00399 if (id_max < sizeof(arm_uc_guid_t)) 00400 { 00401 err.code = ARM_UC_DI_ERR_SIZE; 00402 } 00403 if (err.error == ERR_NONE) 00404 { 00405 err = pal_getDeviceGuid(&guid); 00406 } 00407 if (err.error == ERR_NONE) 00408 { 00409 memcpy(id, guid, sizeof(arm_uc_guid_t)); 00410 if (id_size != NULL) 00411 { 00412 *id_size = sizeof(arm_uc_guid_t); 00413 } 00414 } 00415 return err; 00416 }
Generated on Tue Jul 12 2022 16:22:13 by
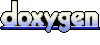