
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
timer_sys.h
00001 /* 00002 * Copyright (c) 2014-2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef _PL_NANO_TIMER_SYS_H_ 00017 #define _PL_NANO_TIMER_SYS_H_ 00018 00019 #ifdef __cplusplus 00020 extern "C" { 00021 #endif 00022 00023 #include "eventOS_event.h" 00024 00025 /* We borrow base event storage, including its list link, and add a time field */ 00026 typedef struct sys_timer_struct_s { 00027 arm_event_storage_t event; 00028 uint32_t launch_time; // tick value 00029 uint32_t period; 00030 } sys_timer_struct_s; 00031 00032 00033 /** 00034 * Initialize system timer 00035 * */ 00036 extern void timer_sys_init(void); 00037 00038 extern uint32_t timer_get_runtime_ticks(void); 00039 int8_t timer_sys_wakeup(void); 00040 void timer_sys_disable(void); 00041 void timer_sys_event_free(struct arm_event_storage *event); 00042 00043 // This require lock to be held 00044 void timer_sys_event_cancel_critical(struct arm_event_storage *event); 00045 00046 /** 00047 * System Timer update and synch after sleep 00048 * 00049 * \param ticks Time in 10 ms resolution 00050 * 00051 * \return none 00052 * 00053 * */ 00054 void system_timer_tick_update(uint32_t ticks); 00055 00056 #ifdef __cplusplus 00057 } 00058 #endif 00059 00060 #endif /*_PL_NANO_TIMER_SYS_H_*/
Generated on Tue Jul 12 2022 16:22:13 by
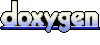