
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "greentea-client/test_env.h" 00003 #include "unity.h" 00004 #include "utest.h" 00005 #include <stdlib.h> 00006 #include <errno.h> 00007 00008 using namespace utest::v1; 00009 00010 // test configuration 00011 #ifndef MBED_TEST_FILESYSTEM 00012 #define MBED_TEST_FILESYSTEM FATFileSystem 00013 #endif 00014 00015 #ifndef MBED_TEST_FILESYSTEM_DECL 00016 #define MBED_TEST_FILESYSTEM_DECL MBED_TEST_FILESYSTEM fs("fs") 00017 #endif 00018 00019 #ifndef MBED_TEST_BLOCKDEVICE 00020 #define MBED_TEST_BLOCKDEVICE SDBlockDevice 00021 #define MBED_TEST_BLOCKDEVICE_DECL SDBlockDevice bd(MBED_CONF_SD_SPI_MOSI, MBED_CONF_SD_SPI_MISO, MBED_CONF_SD_SPI_CLK, MBED_CONF_SD_SPI_CS); 00022 #endif 00023 00024 #ifndef MBED_TEST_BLOCKDEVICE_DECL 00025 #define MBED_TEST_BLOCKDEVICE_DECL MBED_TEST_BLOCKDEVICE bd 00026 #endif 00027 00028 #ifndef MBED_TEST_FILES 00029 #define MBED_TEST_FILES 4 00030 #endif 00031 00032 #ifndef MBED_TEST_DIRS 00033 #define MBED_TEST_DIRS 4 00034 #endif 00035 00036 #ifndef MBED_TEST_BUFFER 00037 #define MBED_TEST_BUFFER 512 00038 #endif 00039 00040 #ifndef MBED_TEST_TIMEOUT 00041 #define MBED_TEST_TIMEOUT 120 00042 #endif 00043 00044 #ifndef MBED_THREAD_COUNT 00045 #define MBED_THREAD_COUNT MBED_TEST_FILES 00046 #endif 00047 00048 // declarations 00049 #define STRINGIZE(x) STRINGIZE2(x) 00050 #define STRINGIZE2(x) #x 00051 #define INCLUDE(x) STRINGIZE(x.h) 00052 00053 #include INCLUDE(MBED_TEST_FILESYSTEM) 00054 #include INCLUDE(MBED_TEST_BLOCKDEVICE) 00055 00056 MBED_TEST_FILESYSTEM_DECL; 00057 MBED_TEST_BLOCKDEVICE_DECL; 00058 00059 Dir dir[MBED_TEST_DIRS]; 00060 File file[MBED_TEST_FILES]; 00061 DIR *dd[MBED_TEST_DIRS]; 00062 FILE *fd[MBED_TEST_FILES]; 00063 struct dirent ent; 00064 struct dirent *ed; 00065 00066 volatile bool count_done = 0; 00067 00068 // tests 00069 00070 void test_file_tests() { 00071 int res = bd.init(); 00072 TEST_ASSERT_EQUAL(0, res); 00073 00074 { 00075 res = MBED_TEST_FILESYSTEM::format(&bd); 00076 TEST_ASSERT_EQUAL(0, res); 00077 } 00078 00079 res = bd.deinit(); 00080 TEST_ASSERT_EQUAL(0, res); 00081 } 00082 00083 void write_file_data (char count) { 00084 00085 char filename[10]; 00086 uint8_t wbuffer[MBED_TEST_BUFFER]; 00087 int res; 00088 00089 sprintf(filename, "%s%d", "data", count); 00090 res = file[count].open(&fs, filename, O_WRONLY | O_CREAT); 00091 TEST_ASSERT_EQUAL(0, res); 00092 00093 char letter = 'A'+ count; 00094 for (uint32_t i = 0; i < MBED_TEST_BUFFER; i++) { 00095 wbuffer[i] = letter++; 00096 if ('z' == letter) { 00097 letter = 'A' + count; 00098 } 00099 } 00100 00101 for (uint32_t i = 0; i < 5; i++) { 00102 res = file[count].write(wbuffer, MBED_TEST_BUFFER); 00103 TEST_ASSERT_EQUAL(MBED_TEST_BUFFER, res); 00104 } 00105 00106 res = file[count].close(); 00107 TEST_ASSERT_EQUAL(0, res); 00108 } 00109 00110 void read_file_data (char count) { 00111 char filename[10]; 00112 uint8_t rbuffer[MBED_TEST_BUFFER]; 00113 int res; 00114 00115 sprintf(filename, "%s%d", "data", count); 00116 res = file[count].open(&fs, filename, O_RDONLY); 00117 TEST_ASSERT_EQUAL(0, res); 00118 00119 for (uint32_t i = 0; i < 5; i++) { 00120 res = file[count].read(rbuffer, MBED_TEST_BUFFER); 00121 TEST_ASSERT_EQUAL(MBED_TEST_BUFFER, res); 00122 char letter = 'A' + count; 00123 for (uint32_t i = 0; i < MBED_TEST_BUFFER; i++) { 00124 res = rbuffer[i]; 00125 TEST_ASSERT_EQUAL(letter++, res); 00126 if ('z' == letter) { 00127 letter = 'A' + count; 00128 } 00129 } 00130 } 00131 00132 res = file[count].close(); 00133 TEST_ASSERT_EQUAL(0, res); 00134 } 00135 00136 void test_thread_access_test() { 00137 00138 Thread *data[MBED_THREAD_COUNT]; 00139 int res = bd.init(); 00140 TEST_ASSERT_EQUAL(0, res); 00141 res = fs.mount(&bd); 00142 TEST_ASSERT_EQUAL(0, res); 00143 00144 // Write threads in parallel 00145 for (char thread_count = 0; thread_count < MBED_THREAD_COUNT; thread_count++) { 00146 data[thread_count] = new Thread(osPriorityNormal); 00147 data[thread_count]->start(callback((void(*)(void*))write_file_data, (void*)thread_count)); 00148 } 00149 00150 // Wait for write thread to join before creating read thread 00151 for (char thread_count = 0; thread_count < MBED_THREAD_COUNT; thread_count++) { 00152 data[thread_count]->join(); 00153 delete data[thread_count]; 00154 data[thread_count] = new Thread(osPriorityNormal); 00155 data[thread_count]->start(callback((void(*)(void*))read_file_data, (void*)thread_count)); 00156 } 00157 00158 // Wait for read threads to join 00159 for (char thread_count = 0; thread_count < MBED_THREAD_COUNT; thread_count++) { 00160 data[thread_count]->join(); 00161 delete data[thread_count]; 00162 } 00163 res = fs.unmount(); 00164 TEST_ASSERT_EQUAL(0, res); 00165 res = bd.deinit(); 00166 TEST_ASSERT_EQUAL(0, res); 00167 } 00168 00169 // test setup 00170 utest::v1::status_t test_setup(const size_t number_of_cases) { 00171 GREENTEA_SETUP(MBED_TEST_TIMEOUT, "default_auto"); 00172 return verbose_test_setup_handler(number_of_cases); 00173 } 00174 00175 Case cases[] = { 00176 Case("File tests", test_file_tests), 00177 Case("Filesystem access from multiple threads", test_thread_access_test), 00178 }; 00179 00180 Specification specification(test_setup, cases); 00181 00182 int main() { 00183 return !Harness::run(specification); 00184 }
Generated on Tue Jul 12 2022 16:22:07 by
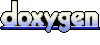